In the Go language, to convert a string into a hexadecimal string, that is, to encode the string into a string in hexadecimal format, you can use the hex
package in the standard library. hex
Package provides functions to convert byte arrays and strings to hexadecimal strings.
The following describes how to convert a string into a hexadecimal string.
- Use the EncodeToString function of the hex package
Sample code:
package main import ( "encoding/hex" "fmt" ) func main() { str := "hello world" encodedStr := hex.EncodeToString([]byte(str)) fmt.Println(encodedStr) }
Running result:
68656c6c6f20776f726c64
- Traverse the string And converted to hexadecimal
Sample code:
package main import "fmt" func main() { str := "hello world" hexStr := "" for _, c := range str { hexStr += fmt.Sprintf("%x", c) } fmt.Println(hexStr) }
Running result:
68656c6c6f20776f726c64
In the above code, we use a for loop to traverse the string Each character, then use the Sprintf
function to format the character into a hexadecimal string, and finally splice the hexadecimal value of each character to obtain the final hexadecimal string.
It should be noted that the Sprintf
function used in the above code uses UTF-8 encoding when converting characters into hexadecimal strings. If you need to use other encoding methods, you can use the strconv
function in the standard library.
Summary:
In Go language, to convert a string into a hexadecimal string, you can use the function provided by the hex
package, or use a loop to traverse the string and convert each character into a hexadecimal string. Both methods can get the desired results. It may be simpler and more efficient to use the functions provided by the hex
package.
The above is the detailed content of golang string to hex. For more information, please follow other related articles on the PHP Chinese website!
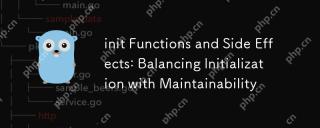
Toensureinitfunctionsareeffectiveandmaintainable:1)Minimizesideeffectsbyreturningvaluesinsteadofmodifyingglobalstate,2)Ensureidempotencytohandlemultiplecallssafely,and3)Breakdowncomplexinitializationintosmaller,focusedfunctionstoenhancemodularityandm
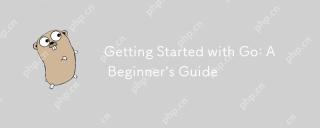
Goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity,efficiency,andconcurrencyfeatures.1)InstallGofromtheofficialwebsiteandverifywith'goversion'.2)Createandrunyourfirstprogramwith'gorunhello.go'.3)Exploreconcurrencyusinggorout
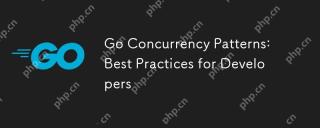
Developers should follow the following best practices: 1. Carefully manage goroutines to prevent resource leakage; 2. Use channels for synchronization, but avoid overuse; 3. Explicitly handle errors in concurrent programs; 4. Understand GOMAXPROCS to optimize performance. These practices are crucial for efficient and robust software development because they ensure effective management of resources, proper synchronization implementation, proper error handling, and performance optimization, thereby improving software efficiency and maintainability.
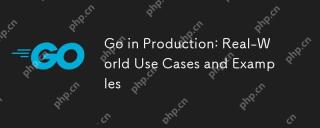
Goexcelsinproductionduetoitsperformanceandsimplicity,butrequirescarefulmanagementofscalability,errorhandling,andresources.1)DockerusesGoforefficientcontainermanagementthroughgoroutines.2)UberscalesmicroserviceswithGo,facingchallengesinservicemanageme
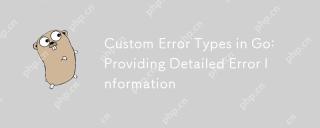
We need to customize the error type because the standard error interface provides limited information, and custom types can add more context and structured information. 1) Custom error types can contain error codes, locations, context data, etc., 2) Improve debugging efficiency and user experience, 3) But attention should be paid to its complexity and maintenance costs.
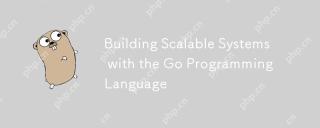
Goisidealforbuildingscalablesystemsduetoitssimplicity,efficiency,andbuilt-inconcurrencysupport.1)Go'scleansyntaxandminimalisticdesignenhanceproductivityandreduceerrors.2)Itsgoroutinesandchannelsenableefficientconcurrentprogramming,distributingworkloa
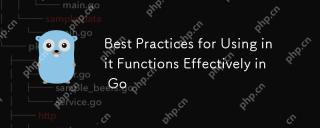
InitfunctionsinGorunautomaticallybeforemain()andareusefulforsettingupenvironmentsandinitializingvariables.Usethemforsimpletasks,avoidsideeffects,andbecautiouswithtestingandloggingtomaintaincodeclarityandtestability.
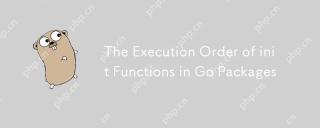
Goinitializespackagesintheordertheyareimported,thenexecutesinitfunctionswithinapackageintheirdefinitionorder,andfilenamesdeterminetheorderacrossmultiplefiles.Thisprocesscanbeinfluencedbydependenciesbetweenpackages,whichmayleadtocomplexinitializations


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
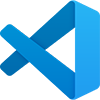
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
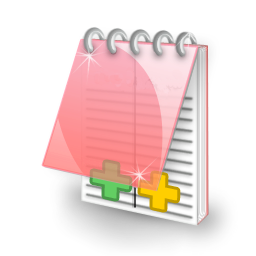
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
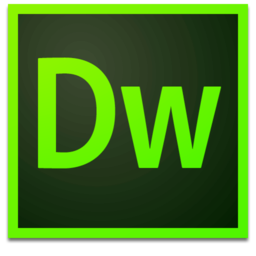
Dreamweaver Mac version
Visual web development tools
