Golang is a modern programming language that is particularly suitable for building high-performance, high-concurrency, and distributed applications. MySQL is a widely used open source relational database that is often used in conjunction with Golang to build reliable and efficient web applications. In this article, we will introduce in detail how to use Golang to set up and connect to MySQL.
- Install MySQL database
Before you start using the MySQL database, you need to complete the installation first. Usually, you need to obtain the available MySQL binary package and install the corresponding version according to the operating system and hardware architecture. There is nothing complicated about this process, just follow the instructions of the installation wizard.
After the installation is complete, start the MySQL service and check whether the service is running normally. On Linux or MacOS systems, you can check whether the MySQL service is available by running the following command:
$ service mysql status
If you are using a Windows operating system, you can find the installed MySQL service in the control panel and check whether normal operation.
- Create MySQL database and table
Before using Golang to connect to the MySQL database, you need to create a database and a table. You can do this by following the steps below:
- Connect to the MySQL command line:
On Linux or MacOS systems, you can connect to the MySQL command line through a terminal window , as follows:
$ mysql -u root -p
You need to enter the user name and password of the MySQL administrator to enter the MySQL command line.
- Create a MySQL database:
In the MySQL command line, execute the following command to create a new database:
$ CREATE DATABASE mydb;
This command will create A MySQL database named "mydb".
- Use the created database:
In the MySQL command line, you can use the following command to use the database you just created:
$ USE mydb;
This The command will switch the current operation object to the database named "mydb".
- Create a MySQL table:
In the MySQL command line, you can use the following command to create a new table:
$ CREATE TABLE mytable ( id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY, first_name VARCHAR(30) NOT NULL, last_name VARCHAR(30) NOT NULL, email VARCHAR(50) );
This command will A MySQL table named "mytable" will be created and four fields will be set for it.
- Golang connects to MySQL
After completing the creation of the MySQL database and table, we can use Golang to connect and perform related operations. The following is the operation process for connecting to MySQL in Golang:
- Import the Golang mysql package:
In your Golang project, you need to import the mysql package first.
import ( "database/sql" "fmt" _ "github.com/go-sql-driver/mysql" )
- Create a MySQL connection:
When connecting to a MySQL database in Golang, you need to create a db object to represent the status of the MySQL connection. Here is how to create a MySQL connection:
func main() { //连接MySQL数据库 db, err := sql.Open("mysql", "user:password@tcp(127.0.0.1:3306)/mydb") if err != nil { fmt.Println(err.Error()) return } defer db.Close() }
In the example, we use user and password to represent the username and password of the MySQL administrator respectively, 127.0.0.1 and 3306 represent the address and port number of the MySQL service, mydb is the name of the MySQL database we just created.
- Execute MySQL query:
After successfully connecting to the MySQL database, we can execute SQL statements to perform various operations. The following is an example of using Golang to insert data into MySQL:
func main() { //连接MySQL数据库 db, err := sql.Open("mysql", "user:password@tcp(127.0.0.1:3306)/mydb") if err != nil { fmt.Println(err.Error()) return } defer db.Close() //插入数据 stmt, err := db.Prepare("INSERT INTO mytable (first_name, last_name, email) VALUES (?, ?, ?)") if err != nil { fmt.Println(err.Error()) return } defer stmt.Close() res, err := stmt.Exec("John", "Doe", "john@doe.com") if err != nil { fmt.Println(err.Error()) return } id, err := res.LastInsertId() if err != nil { fmt.Println(err.Error()) return } fmt.Printf("Inserted a new row with ID: %d ", id) }
The above code uses Golang's database/sql package and the MySQL driver, opens a connection, and then executes an INSERT statement to insert data into our in the table. In this process, we use the Prepare function to prepare the SQL statement and return an stmt object. We then use the Exec function to insert the data into the MySQL database. If the execution is successful, we will be able to get the ID of the row we inserted.
- Summary
This article details how to use Golang to connect to a MySQL database, create databases and tables in MySQL, and use Golang to perform MySQL operations. When you understand this connection method, you can easily develop high-level web applications using Golang with correct MySQL operations and unbeatable performance.
The above is the detailed content of How to set up mysql in golang. For more information, please follow other related articles on the PHP Chinese website!
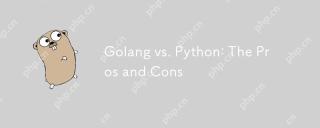
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
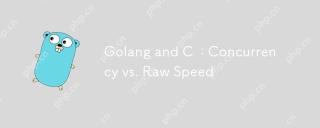
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
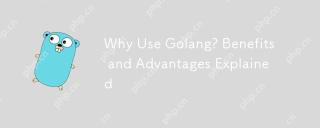
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
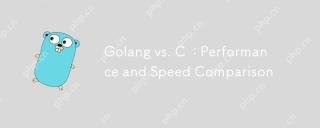
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
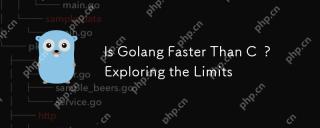
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
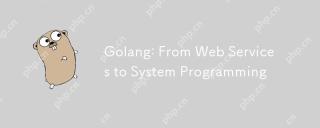
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
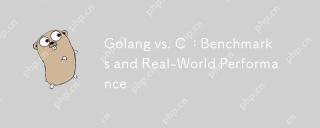
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
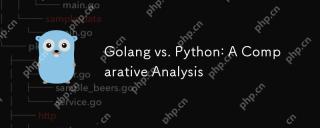
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
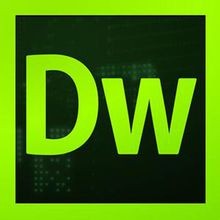
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
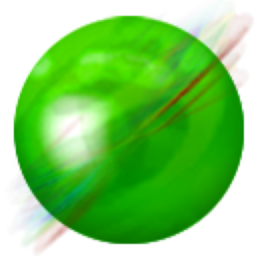
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment