Golang is a language that supports object-oriented programming, and pointers and methods are often used in actual development. A pointer is a variable that stores the memory address of a variable. A method is a function that specifies a type. There is a difference between pointer methods and non-pointer methods in Golang. This article will explore the differences and uses of these two methods.
1. Pointer method
The pointer method is a method bound to a structure pointer. Pointer methods have the following characteristics:
- The receiver type of a pointer method is a pointer to a certain type.
- Pointer methods can modify the value pointed to by the receiver.
- If a type's method needs to modify its receiver itself, it must use pointer methods.
For example, we define a structure type:
type Rectangle struct { width float64 height float64 }
We can use pointer methods to modify instances of this structure:
func (r *Rectangle) SetProperty(width, height float64) { r.width = width r.height = height }
In the above method, Receiver (r *Rectangle)
is a pointer to a Rectangle structure. This method is used to set the properties of Rectangle.
2. Non-pointer method
The non-pointer method is a method bound to a structure. It accepts a copy of the structure as the receiver parameter. The non-pointer method has the following characteristics :
- The receiver type of a non-pointer method is a copy of the value of the type.
- Non-pointer methods cannot modify the value of the receiver.
For example, we define a structure type:
type Rectangle struct { width float64 height float64 }
We can use non-pointer methods to calculate the area of the structure:
func (r Rectangle) GetArea() float64 { return r.width * r.height }
In the above method , the receiver r Rectangle
is a copy of the value of the Rectangle structure. This method returns the area of the rectangle.
3. The difference between pointer methods and non-pointer methods
- Pointer methods can modify the properties in the receiver, but non-pointer methods cannot.
- Pointer methods can avoid copying the object when called because they can directly access the address of the object. This feature is particularly useful when working with large structures.
- Non-pointer methods must copy the entire object when called, which may cause performance issues.
- When defining a method, you can use non-pointer methods if you do not need to modify the receiver itself, otherwise you must use pointer methods.
For example, we can use pointer methods to modify the structure:
func main() { rect := Rectangle{width: 10, height: 5} fmt.Println("Width:", rect.width, "Height:", rect.height) rect.SetProperty(20, 10) fmt.Println("Width:", rect.width, "Height:", rect.height) }
Output result:
Width: 10 Height: 5 Width: 20 Height: 10
4. Summary
In Golang Among them, pointer methods and non-pointer methods have their own advantages, disadvantages and usage scenarios. When defining methods, you need to consider the usage of the object and business needs, and choose an appropriate method to write code. Proper use of both methods can improve code readability and performance.
The above is the detailed content of The difference between golang pointer methods. For more information, please follow other related articles on the PHP Chinese website!
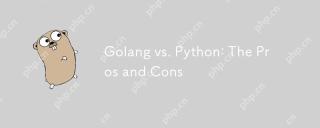
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
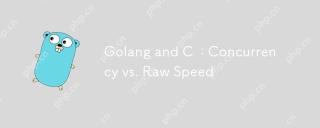
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
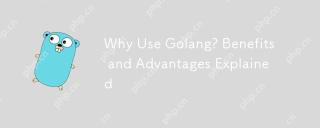
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
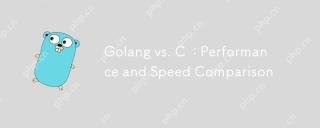
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
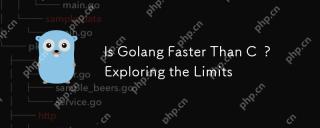
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
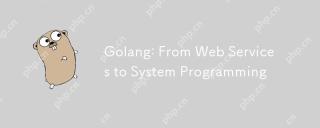
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
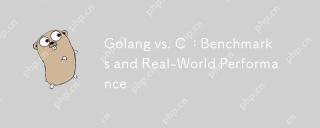
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
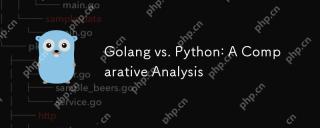
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
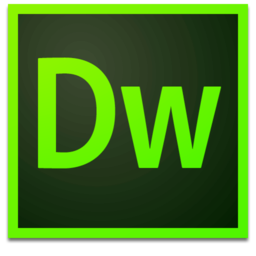
Dreamweaver Mac version
Visual web development tools
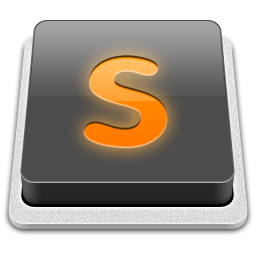
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
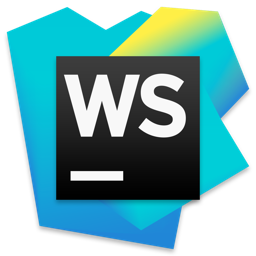
WebStorm Mac version
Useful JavaScript development tools