JavaScript is a scripting language widely used in front-end development. In web applications, JavaScript can help us achieve a variety of interactive effects and functions. Among them, hidden fields and arrays are two features commonly used in JavaScript development.
1. Hidden Field
A hidden field is a field hidden in an HTML form. It will not be displayed on the user interface, but it can be accessed through JavaScript code. , plays a very important role in data transmission and processing.
First of all, the syntax for defining hidden fields in HTML forms is as follows:
<input type="hidden" name="fieldName" value="fieldValue">
Here, type="hidden"
means that this is a hidden field; name
represents the name of the field; value
represents the value of the field. When the user submits the form, the value of this field is submitted to the server for processing. If you need to get or modify the value of this field through JavaScript code, you can use the following syntax:
var fieldValue = document.getElementsByName("fieldName")[0].value; // 获取隐藏字段的值 document.getElementsByName("fieldName")[0].value = "newFieldValue"; // 修改隐藏字段的值
Here, document.getElementsByName
can return all elements with the specified name in the page, [ 0]
means getting the first element, because there is usually only one hidden field in the form, so we can use [0]
to get it.
2. Array
An array is a list composed of multiple elements. Each element has a corresponding subscript. You can access and process the elements in the array through the subscript. element. In JavaScript, array is a very basic and important data type. It can be used to store and process data, which greatly facilitates developers to manage and operate data.
The syntax for creating an array is as follows:
var arr = []; // 创建一个空数组 var arr = [1, 2, 3]; // 创建一个包含 1、2、3 三个元素的数组
The syntax for accessing array elements is as follows:
var arr = [1, 2, 3]; var firstElement = arr[0]; // 获取第一个元素,即 1 arr[2] = 4; // 修改第三个元素的值为 4
Arrays also have many advanced operations, such as array sorting, filtering, and traversal , interception, etc., developers need to have an in-depth understanding of them.
3. Implementation of storing array values in hidden fields
Next, we will introduce a more practical method, which is to store array values in hidden fields. This method can conveniently submit the value of an array to the server when the form is submitted, saving a lot of trouble.
The specific implementation is divided into two steps:
The first step: convert the value of the array into a string. Because the value of the hidden field can only be of string type, we need to convert the value of the array into a string and store it in the hidden field, such as:
var arr = [1, 2, 3]; var arrStr = arr.join(","); // 将数组转换成字符串,用逗号隔开。arrStr 的值为 "1,2,3" document.getElementsByName("fieldName")[0].value = arrStr; // 将字符串存储到隐藏字段中
Step 2: Get the value stored in the hidden field string and convert it to an array. When the page loads, we need to take out the string stored in the hidden field and convert it into an array. The sample code is as follows:
window.onload = function() { var arrStr = document.getElementsByName("fieldName")[0].value; // 获取隐藏字段的值 var arr = arrStr.split(","); // 将字符串转换成数组,使用 "," 作为分隔符 console.log(arr[0]); // 输出数组的第一个元素,即 1 }
The split
function is used here to split the string into an array, and the separator is comma. In this way, we successfully retrieved the array value stored in the hidden field and successfully converted it into an array.
Summary
JavaScript’s hidden fields and arrays are two very commonly used features that can provide developers with very convenient operations and solutions. When we need to submit the value of an array to the server when the form is submitted, the value of the array can be stored in a hidden field, which greatly simplifies the development process. At the same time, array operations also require an in-depth understanding of its basic syntax and advanced operations, which is very helpful for developers to improve their JavaScript skills.
The above is the detailed content of javascript hidden field array value. For more information, please follow other related articles on the PHP Chinese website!

HTML and React can be seamlessly integrated through JSX to build an efficient user interface. 1) Embed HTML elements using JSX, 2) Optimize rendering performance using virtual DOM, 3) Manage and render HTML structures through componentization. This integration method is not only intuitive, but also improves application performance.

React efficiently renders data through state and props, and handles user events through the synthesis event system. 1) Use useState to manage state, such as the counter example. 2) Event processing is implemented by adding functions in JSX, such as button clicks. 3) The key attribute is required to render the list, such as the TodoList component. 4) For form processing, useState and e.preventDefault(), such as Form components.

React interacts with the server through HTTP requests to obtain, send, update and delete data. 1) User operation triggers events, 2) Initiate HTTP requests, 3) Process server responses, 4) Update component status and re-render.

React is a JavaScript library for building user interfaces that improves efficiency through component development and virtual DOM. 1. Components and JSX: Use JSX syntax to define components to enhance code intuitiveness and quality. 2. Virtual DOM and Rendering: Optimize rendering performance through virtual DOM and diff algorithms. 3. State management and Hooks: Hooks such as useState and useEffect simplify state management and side effects handling. 4. Example of usage: From basic forms to advanced global state management, use the ContextAPI. 5. Common errors and debugging: Avoid improper state management and component update problems, and use ReactDevTools to debug. 6. Performance optimization and optimality

Reactisafrontendlibrary,focusedonbuildinguserinterfaces.ItmanagesUIstateandupdatesefficientlyusingavirtualDOM,andinteractswithbackendservicesviaAPIsfordatahandling,butdoesnotprocessorstoredataitself.

React can be embedded in HTML to enhance or completely rewrite traditional HTML pages. 1) The basic steps to using React include adding a root div in HTML and rendering the React component via ReactDOM.render(). 2) More advanced applications include using useState to manage state and implement complex UI interactions such as counters and to-do lists. 3) Optimization and best practices include code segmentation, lazy loading and using React.memo and useMemo to improve performance. Through these methods, developers can leverage the power of React to build dynamic and responsive user interfaces.

React is a JavaScript library for building modern front-end applications. 1. It uses componentized and virtual DOM to optimize performance. 2. Components use JSX to define, state and attributes to manage data. 3. Hooks simplify life cycle management. 4. Use ContextAPI to manage global status. 5. Common errors require debugging status updates and life cycles. 6. Optimization techniques include Memoization, code splitting and virtual scrolling.

React's future will focus on the ultimate in component development, performance optimization and deep integration with other technology stacks. 1) React will further simplify the creation and management of components and promote the ultimate in component development. 2) Performance optimization will become the focus, especially in large applications. 3) React will be deeply integrated with technologies such as GraphQL and TypeScript to improve the development experience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
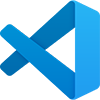
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.