Today we will briefly introduce how to convert JavaScript Map objects into PHP arrays.
First, we need to understand what a Map object is. The Map object is a key-value data structure that can store any type of value and object as a key, and the corresponding value can be easily accessed through the key.
In JavaScript, we can use the following code to create a Map object and add data to it:
let myMap = new Map(); myMap.set("key1", "value1"); myMap.set("key2", "value2");
The above code creates a new Map object and adds two data to it. key-value pairs. We can use the get method to get the corresponding value:
console.log(myMap.get("key1")); // 输出 "value1" console.log(myMap.get("key2")); // 输出 "value2"
Okay, now we have created a Map object and added some data to it. Next, we will convert this Map object into a PHP array.
PHP’s array is somewhat similar to JavaScript’s array. They are both data types that can hold multiple values. However, PHP arrays are more powerful than JavaScript arrays and can accommodate any type of data, including arrays, objects, etc.
We can use the following code to convert a Map object into a PHP array:
// 将Map对象转换成PHP数组 function mapToPhpArray($map) { $array = array(); foreach ($map as $key => $value) { if (is_object($value)) { $value = (array)$value; } elseif (is_array($value) && isset($value[0]) && is_object($value[0])) { $arr = array(); foreach ($value as $k => $v) { $arr[$k] = (array)$v; } $value = $arr; } $array[$key] = $value; } return $array; } // 示例代码 $myMap = new \Ds\Map(); $myMap->put("key1", "value1"); $myMap->put("key2", "value2"); $phpArray = mapToPhpArray($myMap); print_r($phpArray);
The above code defines a function called mapToPhpArray, which accepts a Map object as a parameter and converts it into a PHP array.
This function implements conversion by traversing each key-value pair in the Map object and checking the type of value. If value is an object, then it will convert it to an array; if value is an array, and the elements in the array are objects, then it will convert each element into an array.
Finally, the function adds all key-value pairs to a new PHP array and returns the array.
Using the above sample code, the JavaScript Map object can be successfully converted into a PHP array.
The above is the method to convert JavaScript Map object into PHP array. I hope to be helpful!
The above is the detailed content of map object to php array. For more information, please follow other related articles on the PHP Chinese website!
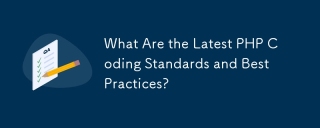
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
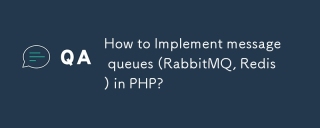
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
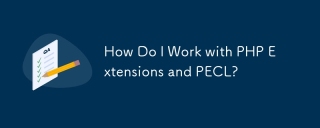
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
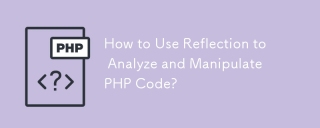
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
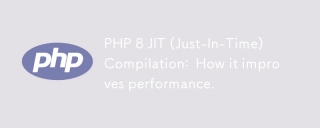
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
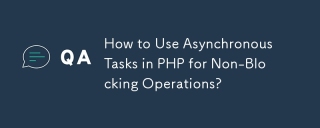
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
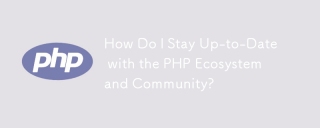
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
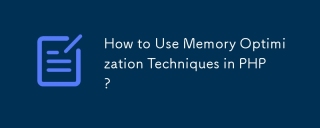
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
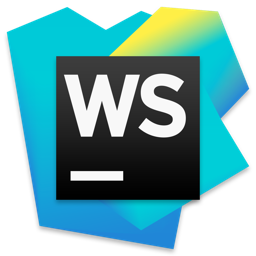
WebStorm Mac version
Useful JavaScript development tools
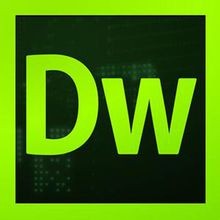
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
