When developing and using golang, you will generally encounter situations where you need to shut down or stop running. For example, if the program ends or an exception occurs, it needs to be shut down gracefully.
Graceful shutdown can stop the program and at the same time allow the started tasks to be processed, avoiding problems such as data loss and resource leakage, and not causing unnecessary losses to the client. This article discusses the graceful shutdown method of golang.
Elegant shutdown method
In golang, it is not difficult to implement graceful shutdown. We can do it by setting a semaphore or channel. The specific implementation method is as follows:
Implemented through semaphores
package main import ( "log" "os" "os/signal" "syscall" "time" ) func main() { stop := make(chan os.Signal, 1) signal.Notify(stop, syscall.SIGINT, syscall.SIGTERM) log.Println("Starting...") go func() { <p>In this code, a signal buffer channel of type os.Signal and length 1 is created through make, and then the operating system is told through the Notify function in the signal package that we want to monitor The signals are SIGINT and SIGTERM, both of which can represent the program's shutdown request. </p><p>Next, we started a goroutine and listened to the stop channel. Once the SIGINT or SIGTERM signal is received, the execution of the goroutine will be triggered. During the execution process, we process the shutdown logic. Time.Sleep is used here to simulate a time-consuming operation to better test whether our program ends normally. Finally, the operating system is notified through the os.Exit function that the program shutdown request has been processed. </p><p>In the main goroutine, we process the business logic and print "Running..." every second. Here is just a simple example; usually, when we process the business logic, we need to save it regularly. data and release resources, etc. </p><h3 id="Through-channel">Through channel</h3><pre class="brush:php;toolbar:false">package main import ( "log" "os" "time" ) func main() { done := make(chan bool) log.Println("Starting...") go func() { osSignals := make(chan os.Signal, 1) signal.Notify(osSignals, syscall.SIGINT, syscall.SIGTERM) select { case <p>In this code, we create a done variable of bool type to indicate whether our program has been completed, and then process the business logic in the main goroutine and print it regularly. "Running..." and listen to done through the select function. If the done variable is set to true, the program ends and returns to the main function to complete the program exit. </p><p>In another goroutine, we create a signal buffer channel of type os.Signal and length 1 through make, and then tell the operating system through the Notify function in the signal package what signal we want to monitor. It's SIGINT and SIGTERM. Next, listen to osSignals through the select function. If a SIGINT or SIGTERM signal is received, a specific operation will be triggered. </p><h2 id="Signals-monitored-by-the-program">Signals monitored by the program</h2><p>In Linux systems, there are generally the following signals: </p>
Signal name | Signal description |
---|---|
Terminal interrupt character, usually issued through Control C | |
Termination signal, usually used to request the program to stop running on its own | |
Suspend signal, usually indicates that a terminal connection has been interrupted and needs Re-read the configuration file, etc. | |
Custom signal | |
Kill The dead process signal cannot be caught or ignored. The process can only be violently killed. | |
The stop process signal cannot be caught or ignored. The process can only be violently stopped. | |
Continue running the process | |
Pipe rupture signal, usually indicating reading data The other party has closed the connection | |
Alarm clock signal, used to trigger an operation regularly | |
Subprocess end signal |
The role of graceful shutdown
Through graceful shutdown, we can avoid data loss, resource leakage and other problems caused by failed requests and operations. For long-running programs and Applications that provide services are especially important. Especially in distributed systems and microservice architectures, graceful shutdown can effectively ensure system stability and performance. If there is no targeted problem handling function, the program may end arbitrarily or trigger a crash after receiving a stop signal, causing serious problems. Causing confusion and even unnecessary losses to the client.
Summary
Through this article, we learned how to use golang's channel and os/signal packages for graceful shutdown, and how to complete graceful shutdown by listening to signals. In actual development, the normal shutdown of an application is a very important task. Graceful shutdown can effectively improve the stability of the program and avoid problems such as resource waste and data loss.
The above is the detailed content of Let's talk about the elegant shutdown method in golang. For more information, please follow other related articles on the PHP Chinese website!
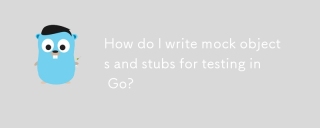
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
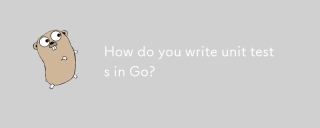
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
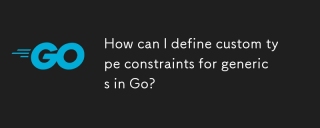
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
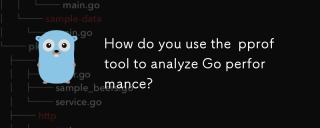
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
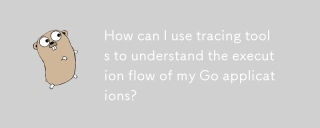
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
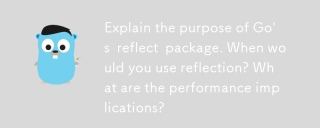
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
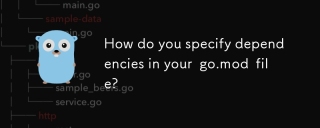
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
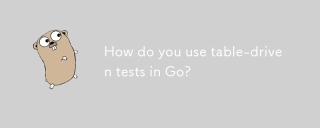
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
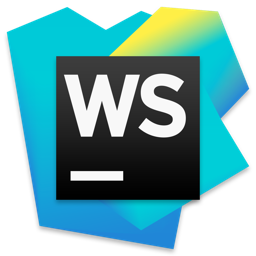
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
