Golang is a very efficient and easy-to-learn programming language, which has been widely used in actual project development. Slices are a very important data structure in golang, which allow us to operate arrays and slices more conveniently. This article will introduce how to use slices in golang.
1. Define and initialize slices
In golang, slices can be used to dynamically adjust the size of an array. A slice is represented by a pointer to the underlying array, a length, and a capacity. The length of a slice refers to the number of elements it contains, and the capacity refers to the number of elements it can hold. Therefore, we can define and initialize a slice in the following way:
var slice []int // 通过 var 关键词声明一个空切片 slice1 := make([]int, 5) // 通过 make 函数创建一个长度为 5 的切片 slice2 := []int{1, 2, 3} // 直接指定切片中的元素 slice3 := []int{0: 1, 2: 3} // 通过索引初始化切片中的元素
2. Add elements to the slice
Use the append() function to add elements to the slice, which will automatically Slices are expanded (if necessary). An example is as follows:
slice = append(slice, 1) // 在 slice 的末尾添加一个元素 slice = append(slice, 2, 3) // 在 slice 的末尾添加两个元素
It should be noted that if the length of the slice exceeds the capacity when adding elements, the append() function will automatically expand the capacity. When expanding, golang will create a new underlying array and copy the original elements to the new array. Therefore, it is not recommended to add a large number of elements to a slice in multiple loops, as this will lead to frequent expansion operations and affect performance.
3. Delete elements from the slice
In golang, deleting elements in the slice is usually achieved by reassigning values. The following is a common implementation:
slice = append(slice[:i], slice[i+1:]...) // 从切片中删除第 i 个元素
This code will split the original slice into two parts and then merge them together. Among them, slice[:i] means from the beginning of the slice to the i-th element (excluding the i-th element), and slice[i 1:] means from the i-th element to the end of the slice. Of the two slices, one contains all elements that need to be retained, and the other does not contain elements that need to be deleted. Finally, combine them together using the ... operator.
In addition, we can also use the copy() function to delete elements. An example is as follows:
copy(slice[i:], slice[i+1:]) // 从切片中删除第 i 个元素 slice = slice[:len(slice)-1] // 删除切片中的最后一个元素
In the above code, the copy() function will move all the elements after the element that needs to be deleted one position forward in sequence, and finally reduce the length of the slice by one to reach the length of the deleted element. Purpose.
4. Copying and intercepting slices
Golang provides two built-in functions to copy and intercept slices: copy() and append(). Below we introduce the usage of these two functions respectively:
- copy() function
var slice1 []int // 定义一个空切片 slice2 := make([]int, 5) // 创建一个长度为 5 的切片 copy(slice1, slice2) // 将 slice2 复制到 slice1 中
It should be noted that the copy() function will only copy the two slices Same elements. If the length of the source slice is greater than the length of the target slice, only the elements that can be accommodated in the target slice will be copied, and the excess elements will be truncated. If the length of the destination slice is greater than the length of the source slice, the excess elements in the destination slice are set to their default values.
- append() function
var slice1 []int // 定义一个空切片 slice2 := []int{1, 2, 3} // 创建一个切片并初始化 slice1 = append(slice1, slice2...) // 将 slice2 追加到 slice1 中
It should be noted that when using the append() function, if you append a slice to another slice, you need to use ... operator to expand it. In this way, the source slice will be expanded into the target slice, rather than the source slice being appended to the target slice as a whole.
5. Slice traversal
You can use the for loop in golang to traverse the elements in the slice. An example is as follows:
for i, val := range slice { fmt.Printf("Index: %d, Value: %d\n", i, val) }
In the above code, a range form of for loop is used to iterate over the elements in the slice. Among them, i represents the index of the element, and val represents the value of the element. As you can see, using range allows us to traverse the elements in the slice more conveniently, which is also the recommended traversal method in golang.
Summary:
This article introduces the definition and initialization of slices in golang, adding elements to slices, deleting elements from slices, copying and intercepting slices, and traversing slices. Slices are a common data structure that can greatly simplify array operations. Therefore, it is very important for Golang developers to master the use of slices.
The above is the detailed content of How to use slices in golang. For more information, please follow other related articles on the PHP Chinese website!
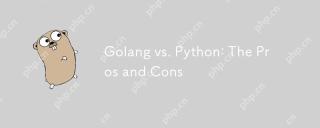
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
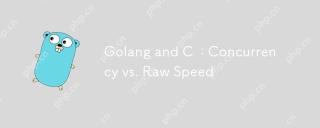
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
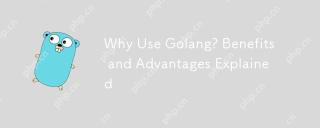
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
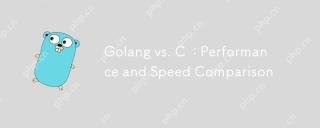
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
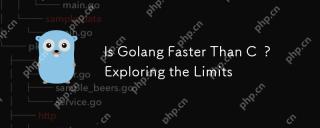
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
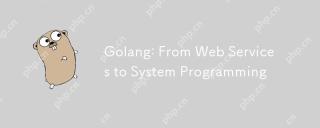
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
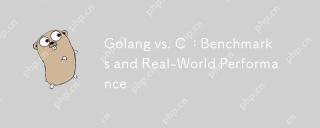
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
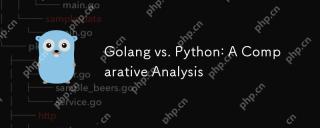
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
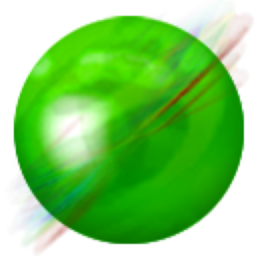
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
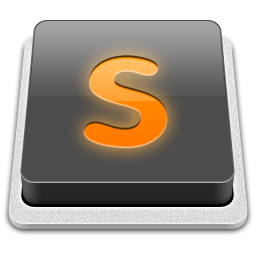
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
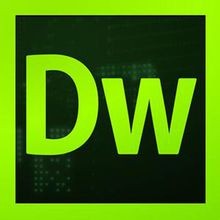
Dreamweaver CS6
Visual web development tools