In PHP development, the problem of determining whether data exists in an array is very common. The correct approach can avoid many unnecessary problems during development. This article will introduce the common methods in PHP to determine whether data exists in an array from the following aspects.
1. Use the count() function
A commonly used method in PHP to determine whether an array is empty is to use the count() function to determine whether the array is empty by counting the number of array elements. . If the number of array elements is 0, the array is empty.
Sample code:
$array = [1, 2, 3]; $count = count($array); if ($count > 0){ echo '数组里有数据'; }else { echo '数组里没有数据'; }
In the above code, we use the count() function to count the number of array elements. When the number of array elements is greater than 0, it outputs "there is data in the array", otherwise it outputs "there is no data in the array".
2. Use the empty() function
The empty() function can very conveniently determine whether an array is empty. The return value of the empty() function is of Boolean type. It returns true when the array is empty, otherwise it returns false.
Sample code:
$array = [1, 2, 3]; if (empty($array)){ echo '数组为空'; }else { echo '数组不为空'; }
In this example, we use the empty() function to determine whether the array is empty. Since the array is not empty, "array is not empty" is output.
3. Use the in_array() function
Array is one of the more commonly used data types in PHP. However, sometimes we need to determine whether a certain value exists in the array. You can use the in_array() function at this time.
Sample code:
$array = [1, 2, 3]; if (in_array(2, $array)){ echo '数组里有2'; }else { echo '数组里没有2'; }
In the above code, we use the in_array() function to determine whether the value 2 exists in the array. Since the value exists in the array, "2 in the array" is output.
4. Use the array_key_exists() function
If we need to determine whether a certain key exists in an array, we can use the array_key_exists() function. The return value of the array_key_exists() function is of Boolean type. It returns true when the key exists, otherwise it returns false.
Sample code:
$array = ['name' => '张三', 'age' => 18]; if (array_key_exists('name', $array)){ echo '数组中有名字'; }else { echo '数组中没有名字'; }
In this example, we use the array_key_exists() function to determine whether the name key exists in the array. Since the key exists, "There is a name in the array" is output.
5. Use the isset() function
Another commonly used method to determine whether an element exists in an array is to use the isset() function. The return value of the isset() function is of Boolean type. It returns true when the specified array element exists, otherwise it returns false.
Sample code:
$array = ['name' => '张三', 'age' => 18]; if (isset($array['name'])){ echo '数组中有名字'; }else { echo '数组中没有名字'; }
In the above code, we use the isset() function to determine whether the name element exists in the array. Since the element exists, "name in array" is output.
Summary:
In PHP development, it is a very common requirement to determine whether data exists in an array. This article introduces several common methods in PHP to determine whether data exists in an array. Including using the count() function, empty() function, in_array() function, array_key_exists() function and isset() function. By mastering these methods, we can ensure the correctness and efficiency of our code.
The above is the detailed content of How to determine whether there is data in an array in php. For more information, please follow other related articles on the PHP Chinese website!
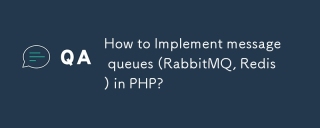
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
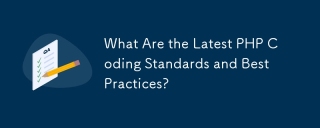
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
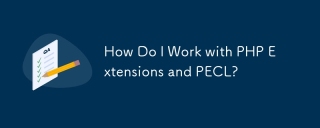
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
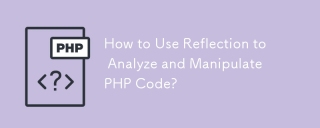
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
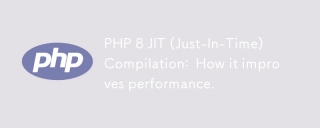
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
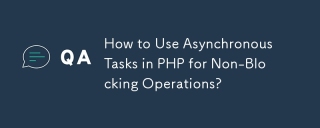
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
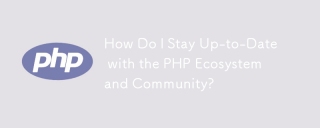
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
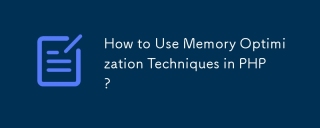
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
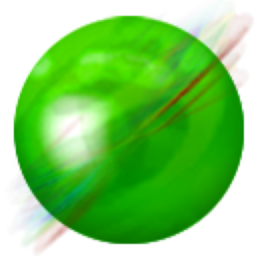
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
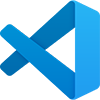
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
