Recently, when I was using uniapp to develop a small program, I encountered a problem: calling the subcomponent method failed. After some research and debugging, I found a solution and now share it with you.
First, let’s take a look at the scenario where this problem occurs. A custom component is introduced into a page, and there is a method in the component that needs to be called externally. We can achieve this by defining the method in the methods of the component object and calling the method inside the component. However, when actually calling, we found that this method always returns a null value, as shown below:
// 引入组件 <template> <custom-component></custom-component> </template> <script> import customComponent from '@/components/custom-component.vue' export default { components: { customComponent }, mounted() { // 调用子组件方法 const data = this.$refs.customComponent.customMethod() console.log(data) // 输出:undefined } } </script> // custom-component.vue <template> <div>这是一个自定义组件</div> </template> <script> export default { methods: { customMethod() { return '这是从子组件返回的数据' } } } </script>
Here we try to call the customMethod method of the child component in the parent component and print the return value of the method . However, in the console we find that the return value is undefined.
After investigation, it was found that this is because the communication method of components in uniapp is different from the communication method of Vue's native components. In uniapp, we need to use uni.$emit to send events and listen to the event in the component. The following is the correct example:
// 引入组件 <template> <custom-component></custom-component> </template> <script> import customComponent from '@/components/custom-component.vue' export default { components: { customComponent }, methods: { onCustomEvent(data) { console.log(data) // 输出:这是从子组件返回的数据 } } } </script> // custom-component.vue <template> <div>这是一个自定义组件</div> </template> <script> export default { methods: { customMethod() { // 向父组件发送事件 this.$emit('customEvent', '这是从子组件返回的数据') } } } </script>
In this example, we use @customEvent to listen to the event of the subcomponent, and process the data passed from the subcomponent in the onCustomEvent method. It should be noted that the method of sending events needs to be done in the child component, rather than calling the method of the child component in the parent component.
This is because in uniapp, the parent component cannot directly call the child component's method. Instead, we need to use events for data transfer and component communication.
To sum up, the component communication methods of uniapp and native Vue are slightly different. In uniapp, we need to communicate between components through events. Especially when calling the method of the subcomponent, we need to use $emit to send the event and listen to the event in the subcomponent to achieve this.
I hope this article is helpful to everyone, thank you for reading!
The above is the detailed content of What should I do if uniapp fails to call a subcomponent method?. For more information, please follow other related articles on the PHP Chinese website!
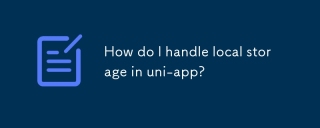
This article details uni-app's local storage APIs (uni.setStorageSync(), uni.getStorageSync(), and their async counterparts), emphasizing best practices like using descriptive keys, limiting data size, and handling JSON parsing. It stresses that lo
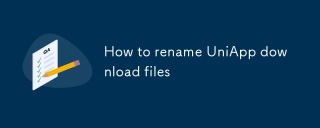
This article details workarounds for renaming downloaded files in UniApp, lacking direct API support. Android/iOS require native plugins for post-download renaming, while H5 solutions are limited to suggesting filenames. The process involves tempor

This article addresses file encoding issues in UniApp downloads. It emphasizes the importance of server-side Content-Type headers and using JavaScript's TextDecoder for client-side decoding based on these headers. Solutions for common encoding prob
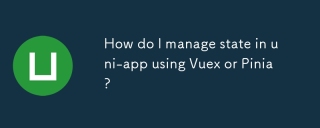
This article compares Vuex and Pinia for state management in uni-app. It details their features, implementation, and best practices, highlighting Pinia's simplicity versus Vuex's structure. The choice depends on project complexity, with Pinia suita

This article details uni-app's geolocation APIs, focusing on uni.getLocation(). It addresses common pitfalls like incorrect coordinate systems (gcj02 vs. wgs84) and permission issues. Improving location accuracy via averaging readings and handling
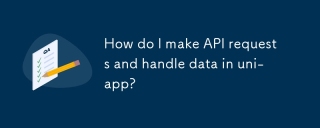
This article details making and securing API requests within uni-app using uni.request or Axios. It covers handling JSON responses, best security practices (HTTPS, authentication, input validation), troubleshooting failures (network issues, CORS, s
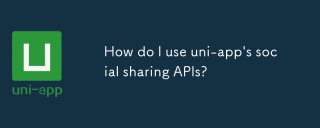
The article details how to integrate social sharing into uni-app projects using uni.share API, covering setup, configuration, and testing across platforms like WeChat and Weibo.
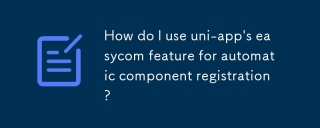
This article explains uni-app's easycom feature, automating component registration. It details configuration, including autoscan and custom component mapping, highlighting benefits like reduced boilerplate, improved speed, and enhanced readability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
