In PHP programming, arrays are one of the frequently used data structures. In an array, sometimes we need to retrieve a certain row in order to perform related operations. So, how to retrieve a row in an array in PHP? This article will introduce it to you in detail.
1. What is an array
First of all, we need to understand what an array is. An array is an ordered collection that stores multiple values. In PHP, an array can be composed of multiple key-value pairs. A key-value pair refers to a key (key) and the corresponding value (value), and there is a certain relationship between them.
In PHP, arrays can be divided into two types: indexed arrays and associative arrays. Index array means that the keys in the array appear in the form of numbers (indexes), for example:
$nums = array(1,2,3,4,5);
Associative array means that the keys in the array appear in the form of strings, for example:
$person = array("name"=>"Tom","age"=>25,"gender"=>"male");
2. How to retrieve a row in an array
In PHP programming, we usually need to retrieve a certain row in the array to facilitate related operations. To retrieve a row in an array, you can use the PHP built-in function array_slice. The array_slice function can slice an array according to the specified parameters. It can intercept part of an array and return the intercepted part as a new array. The basic syntax of the array_slice function is as follows:
array array_slice(array $array, int $offset[, int $length[, bool $preserve_keys]])
Among them, the $array parameter is the array to be sliced, the $offset parameter specifies the starting position of interception, and the $length parameter specifies the number of elements to be intercepted. If the $length parameter is omitted, it means that all elements starting from $offset to the end of the array are intercepted. The $preserve_keys parameter is an optional parameter. If set to true, the key names of the original array will be retained.
The following is a simple example to demonstrate how to use the array_slice function to retrieve a row in an array. Suppose we have an index array containing 5 elements, named $nums, with the following content:
$nums = array(10,20,30,40,50);
If you need to retrieve the third row in the array, that is, intercept the third element from the array and its Subsequent elements can be implemented using the array_slice function. The specific code is as follows:
$third_row = array_slice($nums, 2); print_r($third_row);
The output result is:
Array ( [0] => 30 [1] => 40 [2] => 50 )
As can be seen from the output result, we successfully retrieved the third row in the array.
3. Practical Application
In addition to the above examples, the array_slice function can also be used in conjunction with other PHP functions to facilitate richer applications. Below we will use a practical case to demonstrate how to apply the array_slice function in practice.
Suppose we have an associative array containing multiple student information, named $students, with the following content:
$students = array(array("name"=>"Tom","age"=>20,"gender"=>"male"), array("name"=>"Jerry","age"=>22,"gender"=>"male"), array("name"=>"Lucy","age"=>21,"gender"=>"female"), array("name"=>"Mary","age"=>19,"gender"=>"female"), array("name"=>"John","age"=>23,"gender"=>"male"));
Each element in the $students array is an associative array, containing Basic information about a student, including name, age and gender. Now we are required to implement a function getStudents, whose function is to obtain student information within a specified range. The specific requirements are as follows:
- The function receives two parameters: $start and $end, which respectively represent the starting and ending lines of the student information to be obtained;
- The function returns a new Array containing information about all students in the specified range.
To achieve the above requirements, we can use the array_slice function to slice the $students array, and then combine it with the PHP built-in function array_values function to rearrange the key names. The specific implementation code is as follows:
function getStudents($students, $start, $end) { $new_students = array_slice($students, $start-1, $end-$start+1); return array_values($new_students); } $new_students = getStudents($students, 2, 4); print_r($new_students);
In the above code, we defined a function named getStudents and called the array_slice and array_values functions inside the function. First, we use the array_slice function to slice the $students array and assign the result to a new array $new_students. We then use the array_values function to rearrange the $new_students array to generate a new array with numeric indices as keys. Finally, we return the new array $new_students at the function return value.
After the getStudents function is called, we use the print_r function to output the return value of the function. The output result is:
Array ( [0] => Array ( [name] => Jerry [age] => 22 [gender] => male ) [1] => Array ( [name] => Lucy [age] => 21 [gender] => female ) [2] => Array ( [name] => Mary [age] => 19 [gender] => female ) )
As can be seen from the output result, we successfully obtained the student information from rows 2 to 4 in the $students array.
4. Summary
This article introduces in detail how to retrieve a row in an array in PHP. By using the PHP built-in function array_slice, we can easily implement array slicing operations. In practical applications, we can also combine other PHP functions to achieve more complex functions. When developing PHP, knowing how to retrieve a row in an array is a very important basic knowledge.
The above is the detailed content of How to retrieve a row in an array in php. For more information, please follow other related articles on the PHP Chinese website!
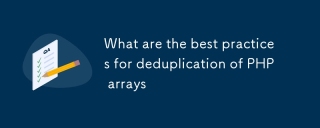
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
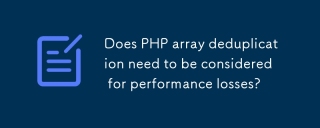
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
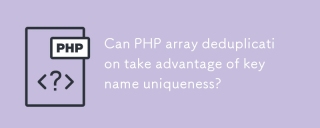
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
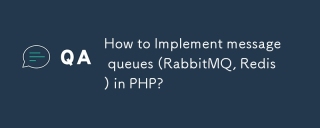
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
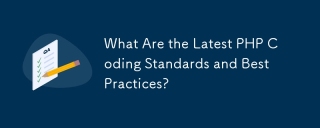
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
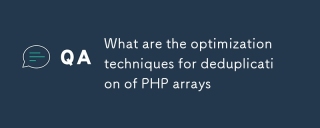
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
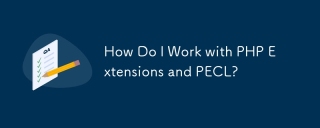
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
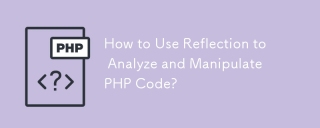
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
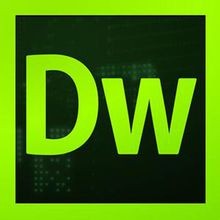
Dreamweaver CS6
Visual web development tools
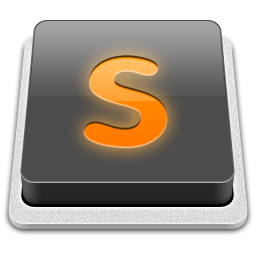
SublimeText3 Mac version
God-level code editing software (SublimeText3)
