With the rapid development of the Internet, message boards have become an essential part of many websites. Among many message boards, the reply function is particularly important because it allows message writers to interact with website administrators, making communication smoother. This article will introduce how to use php to implement the reply function of the message board.
1. Database design
Before implementing the message board reply function, you first need to design a data table to store the message and reply content. This article uses the MySQL database. The following is the design of the message table and reply table:
Message table (message)
Column name | Data type | Explanation |
---|---|---|
int(11) | Message ID | |
varchar(20) | Name of the commenter | |
varchar( 255) | Message content | |
datetime | Message time | |
datetime | Modification time |
Data type | Explanation | |
---|---|---|
int(11) | Reply ID | |
int(11) | Message ID | |
varchar(20) | Replyer name | |
varchar(255) | Reply content | |
datetime | Reply time | |
datetime | Modification time |
2. Page layout
After the database design is completed, the next step is page layout. For message boards, it is important that the interface is simple, beautiful and easy to use. This article uses the Bootstrap framework to design the message board page. The following is the code of the message board page:
nbsp;html> <meta> <title>留言板</title> <link> <script></script> <script></script> <div> <h2 id="留言板">留言板</h2> <form> <div> <label>姓名:</label> <div> <input> </div> </div> <div> <label>留言内容:</label> <div> <textarea></textarea> </div> </div> <div> <div> <button>提交</button> </div> </div> </form> <?php // 查询留言列表 $sql = "SELECT * FROM message ORDER BY created_at DESC"; $result = mysqli_query($conn, $sql); while ($row = mysqli_fetch_assoc($result)) { ?> <div> <div> <?php echo $row['username']; ?>(<?php echo $row['created_at']; ?>) </div> <div> <?php echo $row['content']; ?> </div> </div> <?php // 查询回复列表 $sql = "SELECT * FROM reply WHERE message_id = {$row['id']} ORDER BY created_at ASC"; $result2 = mysqli_query($conn, $sql); while ($row2 = mysqli_fetch_assoc($result2)) { ?> <div> <div> <?php echo $row2['username']; ?>(<?php echo $row2['created_at']; ?>) </div> <div> <?php echo $row2['content']; ?> </div> </div> <?php } ?> <form> <div> <div> <input>"> <input> </div> </div> <div> <div> <textarea></textarea> </div> </div> <div> <div> <button>回复</button> </div> </div> </form> <?php } ?> </div>
In the above code, the message board page is divided into two parts, the upper part is the message box, and the lower part is the message list. and reply form. Both the message list and the reply form are dynamically generated. The reply form of each message has a hidden message_id field to facilitate server-side processing.
3. Back-end implementation
After the page layout is completed, the next step is back-end implementation. In this article, the object-oriented PHP programming method is adopted to facilitate code expansion and maintenance. The following is the PHP code:
<?php // 建立数据库连接 $conn = new mysqli("localhost", "root", "password", "test"); if ($conn->connect_error) { die("数据库连接失败:" . $conn->connect_error); } // 定义Message类 class Message { public $id; public $username; public $content; public $created_at; public $updated_at; // 构造函数 function __construct($id, $username, $content, $created_at, $updated_at) { $this->id = $id; $this->username = $username; $this->content = $content; $this->created_at = $created_at; $this->updated_at = $updated_at; } // 静态方法:获取留言列表 static function get_list($conn) { $messages = array(); $sql = "SELECT * FROM message ORDER BY created_at DESC"; $result = mysqli_query($conn, $sql); while ($row = mysqli_fetch_assoc($result)) { $message = new Message($row['id'], $row['username'], $row['content'], $row['created_at'], $row['updated_at']); array_push($messages, $message); } return $messages; } } // 定义Reply类 class Reply { public $id; public $message_id; public $username; public $content; public $created_at; public $updated_at; // 构造函数 function __construct($id, $message_id, $username, $content, $created_at, $updated_at) { $this->id = $id; $this->message_id = $message_id; $this->username = $username; $this->content = $content; $this->created_at = $created_at; $this->updated_at = $updated_at; } // 静态方法:根据留言ID获取回复列表 static function get_list_by_message_id($conn, $message_id) { $replies = array(); $sql = "SELECT * FROM reply WHERE message_id = {$message_id} ORDER BY created_at ASC"; $result = mysqli_query($conn, $sql); while ($row = mysqli_fetch_assoc($result)) { $reply = new Reply($row['id'], $row['message_id'], $row['username'], $row['content'], $row['created_at'], $row['updated_at']); array_push($replies, $reply); } return $replies; } } // 处理留言提交 if ($_SERVER['REQUEST_METHOD'] == 'POST' && !empty($_POST['username']) && !empty($_POST['content'])) { $sql = "INSERT INTO message (username, content) VALUES ('{$_POST['username']}', '{$_POST['content']}')"; mysqli_query($conn, $sql); } // 处理回复提交 if ($_SERVER['REQUEST_METHOD'] == 'POST' && !empty($_POST['message_id']) && !empty($_POST['username']) && !empty($_POST['content'])) { $sql = "INSERT INTO reply (message_id, username, content) VALUES ({$_POST['message_id']}, '{$_POST['username']}', '{$_POST['content']}')"; mysqli_query($conn, $sql); } // 获取留言列表 $messages = Message::get_list($conn); ?>
In the above code, the Message class and Reply class are defined, corresponding to messages and replies. In the Message class, the static method get_list is defined to obtain the message list; in the Reply class, the static method get_list_by_message_id is defined to obtain the reply list. At the same time, PHP's built-in $_POST array is used to process form submission data, and message and reply data are inserted into the corresponding data table.
At this point, the entire process of implementing the message board reply function in PHP has been completed. Through the design and implementation of the above code, we can easily implement the message board reply function, which improves the user experience and facilitates website management.
The above is the detailed content of How to use php to implement the reply function of message board. For more information, please follow other related articles on the PHP Chinese website!
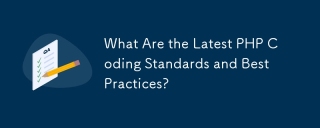
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
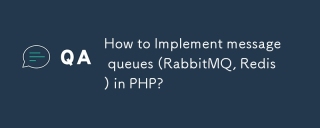
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
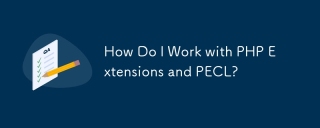
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
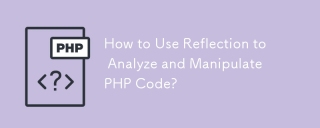
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
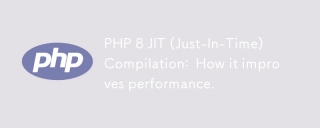
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
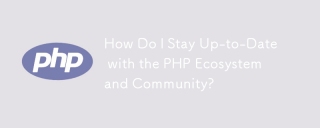
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
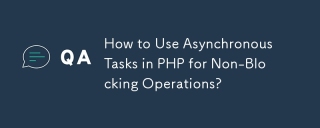
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
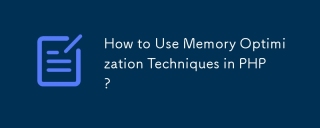
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
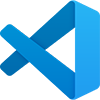
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
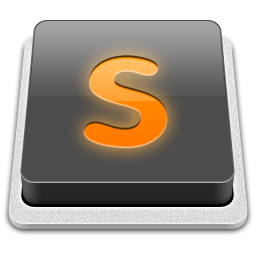
SublimeText3 Mac version
God-level code editing software (SublimeText3)
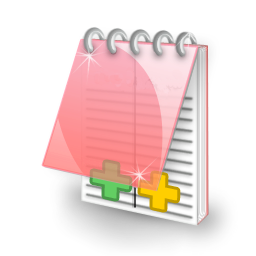
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
