In many web applications, XML is widely used as a common data transmission format. As a common web development language, PHP naturally needs to be able to process XML data easily. In PHP, converting XML data to array is a very common need. This article will explain how to convert XML data into an array using PHP.
First, to convert XML data into an array, you need to use simplexml_load_string(), a built-in function in PHP. This function loads an XML string into PHP and parses it, returning a SimpleXMLElement instance. SimpleXMLElement is a class in PHP specifically used to process XML data. It contains many useful methods to traverse and manipulate XML data.
Suppose we have the following XML data:
<?xml version="1.0" encoding="UTF-8"?> <bookstore> <book> <title>Harry Potter</title> <author>J.K. Rowling</author> <price>29.99</price> </book> <book> <title>The Hunger Games</title> <author>Suzanne Collins</author> <price>22.99</price> </book> </bookstore>
We can use the simplexml_load_string() function to load it into PHP:
$xml = '<?xml version="1.0" encoding="UTF-8"?> <bookstore> <book> <title>Harry Potter</title> <author>J.K. Rowling</author> <price>29.99</price> </book> <book> <title>The Hunger Games</title> <author>Suzanne Collins</author> <price>22.99</price> </book> </bookstore>'; $xmlObj = simplexml_load_string($xml);
Now $xmlObj is a SimpleXMLElement instance, we can use its methods to traverse and manipulate XML data. However, if we need to further process the XML data, such as filtering and sorting certain nodes, it is not convenient to use SimpleXMLElement. Therefore, we need to convert it to an array.
Converting SimpleXMLElement to an array is very simple, just add an (array) cast before the object:
$xmlArr = (array) $xmlObj; print_r($xmlArr);
The above code will output the following results:
Array ( [book] => Array ( [0] => Array ( [@attributes] => Array ( [category] => children ) [title] => Array ( [@attributes] => Array ( [lang] => en ) [0] => Harry Potter ) [author] => J.K. Rowling [price] => 29.99 ) [1] => Array ( [@attributes] => Array ( [category] => fiction ) [title] => Array ( [@attributes] => Array ( [lang] => en ) [0] => The Hunger Games ) [author] => Suzanne Collins [price] => 22.99 ) ) )
As you can see, we get a two-dimensional array, in which each node is converted into an associative array. If there are multiple nodes with the same name in the XML data, they will be converted into an array. For example, the two books here are converted into a book array.
It should be noted that in the converted array, each node has a key named @attributes, which corresponds to the attributes of the node. For example, the book node has a category attribute, and its value is converted to the value of the @attributes['category'] key.
In addition, since SimpleXMLElement is a recursive structure, it contains many nested child nodes. Therefore, the converted array is also a recursive structure, where each sub-array corresponds to a child node.
Of course, if there are some special nodes in the XML data that require special processing, such as CDATA nodes, empty nodes, etc., you need to use some special methods to handle them. But for ordinary XML data, using simplexml_load_string() and (array) cast is convenient enough to convert XML data into an array.
The above is the detailed content of How to convert xml to array in php. For more information, please follow other related articles on the PHP Chinese website!
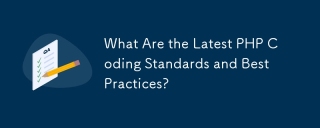
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
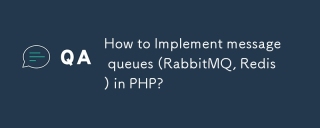
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
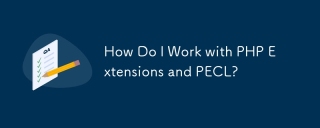
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
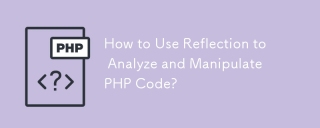
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
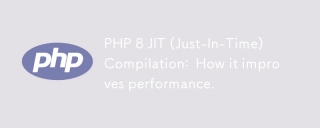
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
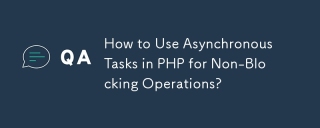
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
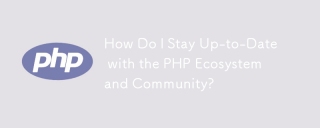
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
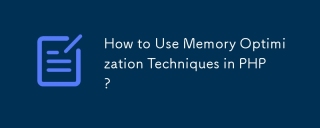
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
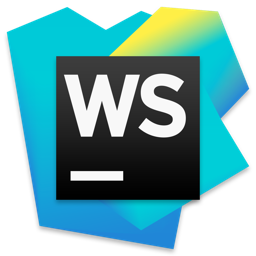
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment
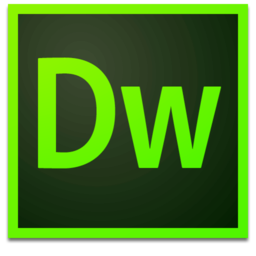
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
