


In PHP, array is a very important data structure that can be used in many scenarios. Two-dimensional array is one of them, usually used to store data such as tables or matrices. In actual development, you may encounter situations where you need to merge two or more two-dimensional arrays into a new two-dimensional array. However, if you directly use PHP's own array_merge
function to merge, a problem will arise: the merged key values will change. So, let’s discuss how to merge two-dimensional arrays without changing the key values.
Understand the structure of two-dimensional arrays
In PHP, a two-dimensional array is actually an array containing multiple one-dimensional arrays. Each one-dimensional array is a subarray in which multiple key-value pairs can be stored. For example, the following is a two-dimensional array containing two sub-arrays:
$array = [ [ 'name' => 'John', 'age' => 25, ], [ 'name' => 'Mary', 'age' => 30, ], ];
It can be seen that each sub-array represents a person's information, where name
and age
is the key, and the corresponding values are the person's name and age. In actual development, many operations need to be performed on data of this structure, such as sorting, filtering, merging, etc. of arrays.
Use the array_merge function to merge two-dimensional arrays
In PHP, there are many ways to merge arrays, among which using the array_merge
function is a common method. This function can merge multiple arrays into a new array, and the merged key values will be automatically reordered. The following is an example of using the array_merge
function to merge two two-dimensional arrays:
$array1 = [ [ 'name' => 'John', 'age' => 25, ], ]; $array2 = [ [ 'name' => 'Mary', 'age' => 30, ], ]; $array = array_merge($array1, $array2); var_dump($array);
The output result is:
array(2) { [0]=> array(2) { ["name"]=> string(4) "John" ["age"]=> int(25) } [1]=> array(2) { ["name"]=> string(4) "Mary" ["age"]=> int(30) } }
It can be seen that the merged array follows the original The order is reordered and the key values are reassigned because the array_merge
function will use the key values of all arrays as the keys of the new array, and the values of the new keys are the values in the original array. If there are duplicate key values in the original array, the subsequent array will overwrite the values in the previous array. This behavior is undesirable in many scenarios, because the key values of the original array may contain important information, and reordering or overwriting may lead to data errors. So, is there a way to preserve the key values of the original array?
Use the array_replace_recursive function to merge two-dimensional arrays
array_replace_recursive
function is another array merging method that comes with PHP, and is different from the array_merge
function Yes, it preserves the key values of the original array rather than reordering or overwriting. In addition, the array_replace_recursive
function also supports recursive merging of arrays and can handle the merging of multi-dimensional arrays. The following is an example of using the array_replace_recursive
function to merge two two-dimensional arrays:
$array1 = [ [ 'name' => 'John', 'age' => 25, ], ]; $array2 = [ [ 'name' => 'Mary', 'age' => 30, ], ]; $array = array_replace_recursive($array1, $array2); var_dump($array);
The output result is:
array(1) { [0]=> array(2) { ["name"]=> string(4) "Mary" ["age"]=> int(30) } }
As you can see, the merged array retains the original array keys and order, rather than changing their order or overwriting their values. In addition, the array_replace_recursive
function also supports merging multi-dimensional arrays, making processing more convenient. However, it should be noted that when merging arrays recursively, if the structures of the arrays are inconsistent, the results may be abnormal, so special attention is required.
Summary
In PHP, two-dimensional array is one of the common data structures. In actual development, you may encounter situations where you need to merge two or more two-dimensional arrays into a new two-dimensional array. If you directly use PHP's own array_merge
function to merge, the array may be reordered or the key values may be overwritten. In order to maintain the key values and order of the original array, you can use the array_replace_recursive
function to merge arrays. In addition, the array_replace_recursive
function also supports recursive merging of arrays, which is very convenient. However, it should be noted that when merging arrays recursively, special attention needs to be paid to the structure of the array to avoid abnormal situations.
The above is the detailed content of How to merge two-dimensional arrays in php without changing key values. For more information, please follow other related articles on the PHP Chinese website!
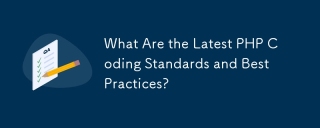
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
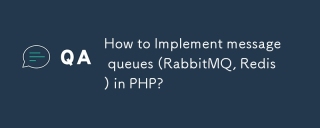
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
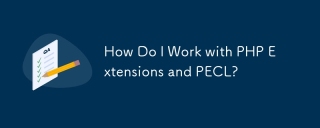
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
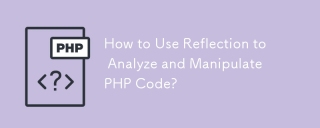
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
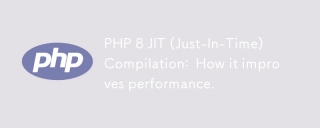
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
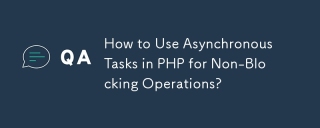
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
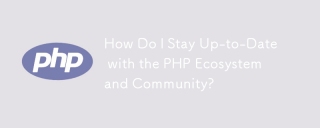
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
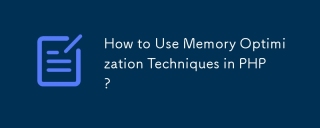
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
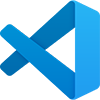
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
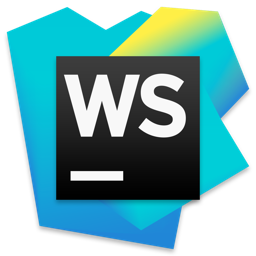
WebStorm Mac version
Useful JavaScript development tools
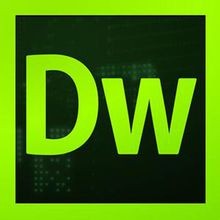
Dreamweaver CS6
Visual web development tools
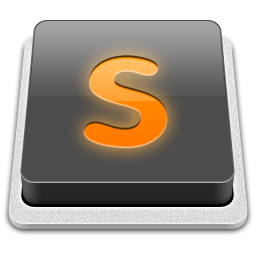
SublimeText3 Mac version
God-level code editing software (SublimeText3)
