Vue.js has become a very popular framework in today's front-end development. As Vue.js continues to evolve, unit testing is becoming more and more important. Today we’ll explore how to write unit tests in Vue.js 3 and provide some best practices and common problems and solutions.
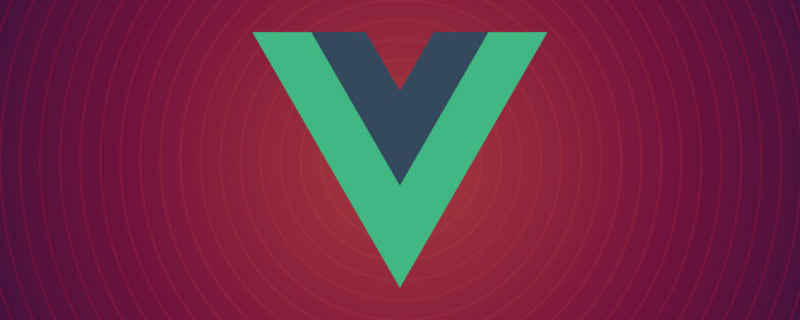
1. Why unit testing is necessary
1.1 The concept of unit testing
Unit testing is a testing method for testing The smallest testable unit in software, usually a single function or method. The purpose of unit testing is to verify that the unit behaves as expected.
1.2 Advantages of unit testing
- Improve the quality of code: By writing unit tests, you can ensure the correctness of the code and reduce the time to fix errors later. [Related recommendations: vuejs video tutorial, web front-end development]
- Improve the maintainability of the code: By writing unit tests, the code can be more easily maintained and Ensure that future modifications do not destabilize the code.
- Improve the readability of the code: By writing unit tests, the logic and functionality of the code can be understood more easily, thereby improving the readability of the code.
1.3 Applicable scenarios for unit testing
- When writing new code, unit tests need to be written to verify the correctness of the code.
- When modifying existing code, unit tests need to be written to verify the correctness of the modification.
- When code refactoring is required, unit tests need to be written to verify the correctness of the refactoring.
2. Unit testing tools in Vue 3
2.1 Overview of Jest
Jest is a popular JavaScript unit testing framework. It supports multiple test formats, including spec, faker, xdescribe, it, etc. Jest provides a series of built-in test functions and assertion functions to make writing unit tests easy.
2.2 Overview of Vue Test Utils
Vue Test Utils is a new testing tool provided in Vue.js 3. It provides some built-in functions, such as ##q、http etc., making it easier to use the plug-ins provided by Vue.js 3 when writing unit tests. 2.3 Configuration of Vue 3 unit tests
In Vue.js 3, the configuration of unit tests requires the use of the Vue.config.js file. You can configure unit testing related settings by setting the test option in the Vue.config.js file. For example, you can set the test path, set the switch of white box testing and black box testing, etc.
3. Vue 3 unit test example
3.1 Test the rendering result of the component
To test the rendering result of the component, you can use the describe function and it function provided by Vue Test Utils . Here is an example:
import { createTestComponent } from 'vue-test-utils';
import MyComponent from '@/components/MyComponent';describe('MyComponent', () => {
const component = createTestComponent(MyComponent); it('renders correct content', () => {
// 设置测试数据
const data = { content: 'Hello Vue!' }; // 运行测试用例
component.$render(); // 获取渲染结果
const renderedContent = component.$el.textContent; // 验证渲染结果是否正确
expect(renderedContent).toBe('Hello Vue!');
});
});
复制代码
In this example, we create a test component MyComponent using the createTestComponent() function and write a test case using the it function. In the test case, we set up the test data and ran the component's $render() method. Finally, we get the rendering result of the component and verify that it is correct.
3.2 Test the interactive behavior of the component
To test the interactive behavior of the component, you can use the describe function and it function provided by Vue Test Utils, as well as the interactive events and life cycle hooks provided by Vue. Here is an example:
import { createTestComponent } from 'vue-test-utils';
import MyComponent from '@/components/MyComponent';describe('MyComponent', () => {
const component = createTestComponent(MyComponent); // 定义一个按钮事件
beforeEach(() => {
component.$el.querySelector('button').addEventListener('click', () => {
// 触发事件
console.log('Button clicked!');
});
}); // 编写测试用例
it('emits an event when clicked', () => {
// 触发按钮事件
component.$el.querySelector('button').click(); // 获取事件响应
const eventHandler = component.$el.addEventListener('click', event => {
// 验证事件响应是否正确
expect(event.preventDefault).toBeFalsy();
expect(event.target).toBe(component.$el);
});
});
});
复制代码
In this example, we define a button event using the beforeEach() function and trigger the event in the test case. Finally, we get the event response using the component.$el.addEventListener() method and verify that it is correct.
3.3 Test the status changes of Vuex Store
In Vue 3, you can use the describe function and it function provided by Vue Test Utils to test the status changes of Vuex Store, as well as the Store and action functions provided by Vuex . Here is an example:
import { createTestStore, createTestReducer } from 'vuex-test-utils';
import MyReducer from '@/reducers/MyReducer';describe('MyReducer', () => {
const store = createTestStore({
reducer: MyReducer,
}); // 定义一个 action
const action = { type: 'ADD_TODO' }; // 编写测试用例
it('adds a new TODO to the store when the action is dispatched', () => {
// 发送 action
store.dispatch(action); // 获取 store 中的状态
const todos = store.state.todos; // 验证状态是否正确
expect(todos.length).toBe(1);
});
});
复制代码
In this example, we create a test Vuex Store using the createTestStore() function and create a test Reducer using the createTestReducer() function. Then, we define an action that adds a new TODO to the store. Finally, we wrote a test case using the it function and verified that the action successfully added a new TODO to the store.
3.4 测试异步请求
在 Vue 3 中,测试异步请求可以使用 Vue Test Utils 提供的 describe 函数和 it 函数,以及 Vue 提供的 Tick 机制。下面是一个示例:
import { createTestComponent } from 'vue-test-utils';
import MyComponent from '@/components/MyComponent';describe('MyComponent', () => {
const component = createTestComponent(MyComponent); // 定义一个异步请求
beforeEach(() => {
component.$nextTick(() => {
// 发送异步请求
axios.get('/api/data').then(response => {
// 验证异步请求是否正确
expect(response.data).toBeDefined();
});
});
}); // 编写测试用例
it('emits an event when clicked', () => {
// 触发按钮事件
component.$el.querySelector('button').click(); // 获取事件响应
const eventHandler = component.$el.addEventListener('click', event => {
// 验证事件响应是否正确
expect(event.preventDefault).toBeFalsy();
expect(event.target).toBe(component.$el);
});
});
});
复制代码
在这个示例中,我们使用 beforeEach() 函数定义了一个异步请求,并在测试用例中触发了该请求。在测试用例中,我们使用了 Vue 的 Tick 机制来确保异步请求在测试用例之间隔离。最后,我们使用 it 函数编写了测试用例,并验证异步请求是否正确。
四、Vue 3 单元测试最佳实践
4.1 编写可测试的组件
编写可测试的组件是单元测试的最佳实践之一。可测试的组件具有以下特点:
- 组件拥有清晰的 API: 组件的 API 应该清晰明了,易于使用。这有助于编写测试用例,并且使组件更容易被复用。
- 组件有单元测试:单元测试是组件编写的一部分。单元测试可以验证组件的输入和输出,并确保组件的行为符合预期。
- 组件有集成测试:集成测试是组件与其他组件或系统之间的交互测试。集成测试可以帮助发现组件与其他组件或系统的兼容性问题。
编写可测试的组件可以提高组件的可读性、可维护性和可扩展性,同时也可以帮助团队更好地管理代码。
4.2 如何编写高质量的测试用例
编写高质量的测试用例是单元测试的另一个最佳实践。以下是一些编写高质量测试用例的建议:
- 确保测试用例覆盖所有可能的情况:测试用例应该覆盖组件的所有输入和输出,以确保组件的行为符合预期。
- 编写简洁、易于理解的测试用例:测试用例应该简洁、易于理解。这有助于编写和维护测试用例,并且可以提高测试用例的可读性。
- 使用断言函数:断言函数可以使测试用例更加健壮。断言函数可以验证组件的输入和输出,并确保组件的行为符合预期。
- 编写单元测试和集成测试:单元测试和集成测试应该分别编写。单元测试应该验证组件的输入和输出,而集成测试应该验证组件与其他组件或系统的兼容性。
4.3 如何优化测试速度
优化测试速度是单元测试的另一个重要最佳实践。以下是一些优化测试速度的建议:
- 使用异步测试:异步测试可以使测试速度更快。异步测试可以让组件在渲染完成之前进行测试,从而提高测试速度。
- 使用 Vue Test Utils 的缓存机制:Vue Test Utils 提供了缓存机制,可以加快测试执行速度。
- 避免在测试中使用全局变量:在测试中使用全局变量会使测试速度变慢。如果必须在测试中使用全局变量,可以考虑在测试用例之间共享变量。
- 避免在测试中使用 Vuex 或其他状态管理工具:在测试中使用 Vuex 或其他状态管理工具会使测试速度变慢。如果必须在测试中使用状态管理工具,可以考虑在其他测试中使用该工具。
五、常见的 Vue 3 单元测试问题及解决方案
5.1 如何测试具有副作用的代码
在测试具有副作用的代码时,我们需要考虑如何防止测试用例之间的干扰,以及如何确保测试环境的稳定性。以下是一些解决方案:
- 隔离测试环境:通过将测试环境与其他代码隔离开来,可以避免测试用例之间的干扰。可以使用 test.config.js 文件中的 setup 和 teardown 方法来编写测试环境初始化和清理代码。
- 使用副作用追踪工具:Vue 3 引入了副作用追踪工具 Vuex Transactions,它可以跟踪代码中的副作用,并在测试过程中自动执行。可以在 test/unit/index.js 文件中引入 Vuex Transactions 并将其实例化,然后将其注入到测试组件中。
- 编写单元测试:对于具有副作用的代码,我们可以编写单元测试来测试其副作用。例如,我们可以编写一个测试用例来测试修改数据的影响,或者编写一个测试用例来测试组件更新的影响。
5.2 如何处理异步测试
异步测试是 Vue 3 单元测试中的一个重要部分。在异步测试中,测试用例可能会等待某个异步操作完成才能执行,因此在测试过程中需要确保测试环境的稳定性。以下是一些处理异步测试的问题和解决方案:
- Handling asynchronous operations: Asynchronous operations are usually managed using the Tick mechanism in Vue 3. In tests, we can use Tick managers to ensure that asynchronous operations are isolated between test cases.
- Handling waiting time: In asynchronous testing, the test case may need to wait for an asynchronous operation to complete before executing. This may lead to inconsistent wait times between test cases, affecting the stability of test results. You can simulate the execution time of an asynchronous operation by using the wait dialog in Vue 3.
- Handling the nesting of asynchronous tests: When asynchronous tests are nested, we need to ensure the stability of the test environment. Asynchronous operations in nested test cases can be managed by using the Tick mechanism in Vue 3.
5.3 How to mock global objects
In testing, we may need to mock global objects. Here are some ways to mock global objects:
- Using the Context API in Vue 3: The Context API in Vue 3 allows us to mock global objects. Mock global objects can be created using the Context API in the test/unit/index.js file.
- Use global variables: Simulate global objects by defining global variables in code. Global variables can be defined using the environment object in the src/config/test.config.js file.
- Using Vuex Store:Vuex Store allows us to simulate global objects. You can use Vuex Store in the test/unit/index.js file to create mock global objects.
5.4 How to simulate routing
In testing, we may need to simulate routing. Here are some ways to simulate routing:
- Using the Context API in Vue 3: The Context API in Vue 3 allows us to simulate routing. Mock routes can be created using the Context API in the test/unit/index.js file.
- Using Vue Router: Vue Router allows us to simulate routing. Mock routes can be created using Vue Router in the test/unit/router/index.js file.
- Use external API: You can use third-party tools or libraries to simulate routing, such as using Redux or Webpack plug-ins to simulate routing.
5.5 How to test the interactive behavior of components
When testing the interactive behavior of components, we need to consider how to simulate user operations and how to ensure stability between test cases. Here are some ways to test the interactive behavior of components:
- Use tools that simulate user operations: You can use tools that simulate user operations to simulate user operations, such as use cases, API Call, Selenium, etc.
- Use the Context API in Vue 3: The Context API in Vue 3 allows us to simulate user operations. You can use the Context API in the test/unit/index.js file to simulate user operations.
- Using Vuex Store:Vuex Store allows us to simulate user operations. You can use Vuex Store in the test/unit/index.js file to simulate user operations.
- Using Vue Router: Vue Router allows us to simulate user operations. You can use Vue Router in the test/unit/router/index.js file to simulate user operations.
(Learning video sharing: vuejs introductory tutorial, Basic programming video)
The above is the detailed content of Explore how to write unit tests in Vue3. For more information, please follow other related articles on the PHP Chinese website!