In PHP, array is a very common and important data structure that can be used to store a set of related data. In the actual development process, we often encounter situations where we need to convert a multi-dimensional array into a one-dimensional array. At this time, we need to use the array function in PHP to achieve this.
PHP provides a variety of methods to convert multi-dimensional arrays into one-dimensional arrays. Below we introduce these methods respectively.
Method 1: array_walk_recursive() function
The array_walk_recursive() function can traverse all elements in a multi-dimensional array and add them to a new one-dimensional array. The following is a sample code using this function:
function array_flatten($array) { $result = array(); array_walk_recursive($array, function($value) use (&$result) { array_push($result, $value); }); return $result; }
In this sample code, we define a function called array_flatten(), which accepts a multi-dimensional array as a parameter and returns a one-dimensional array. This function first creates an empty array $result, and then uses the array_walk_recursive() function to walk through all elements in the multidimensional array and add them to the $result array. Finally, the function returns the $result array.
Method 2: RecursiveIteratorIterator class
The RecursiveIteratorIterator class in PHP can also be used to traverse all elements in a multi-dimensional array and add them to a new one-dimensional array. The following is a sample code using this class:
function array_flatten($array) { $result = array(); $iterator = new RecursiveIteratorIterator(new RecursiveArrayIterator($array)); foreach ($iterator as $value) { array_push($result, $value); } return $result; }
In this sample code, we define a function called array_flatten(), which accepts a multi-dimensional array as a parameter and returns a one-dimensional array. The function first creates an empty array $result, and then uses the RecursiveIteratorIterator class and the RecursiveArrayIterator class to iterate through all elements in the multidimensional array and add them to the $result array. Finally, the function returns the $result array.
Method 3: Recursive function
Finally, we can also use recursive functions to convert multi-dimensional arrays into one-dimensional arrays. The following is a sample code using a recursive function:
function array_flatten($array) { $result = array(); foreach ($array as $value) { if (is_array($value)) { $result = array_merge($result, array_flatten($value)); } else { array_push($result, $value); } } return $result; }
In this sample code, we define a function called array_flatten(), which accepts a multi-dimensional array as a parameter and returns a one-dimensional array. This function first creates an empty array $result and iterates through all elements in the multidimensional array. If the current element is an array, the array_flatten() function is called recursively to process the array and the returned result is combined with the $result array. Otherwise, add the current element to the $result array. Finally, the function returns the $result array.
The above three methods can convert multi-dimensional arrays into one-dimensional arrays. Developers can choose a suitable method according to their own needs. In actual development, we also need to pay attention to the possibility that key names in multi-dimensional arrays have the same key name. In this case, we must handle it according to the specific situation.
The above is the detailed content of How to convert multi-dimensional to one-dimensional array in php. For more information, please follow other related articles on the PHP Chinese website!
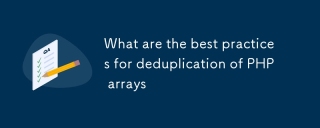
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
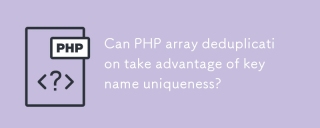
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
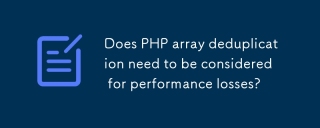
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
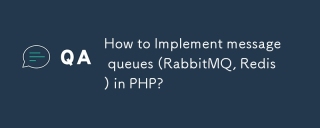
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
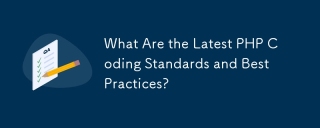
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
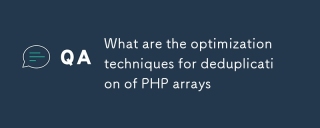
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
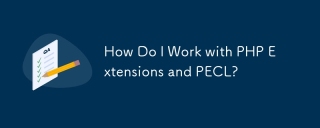
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
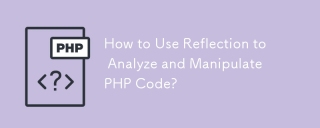
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
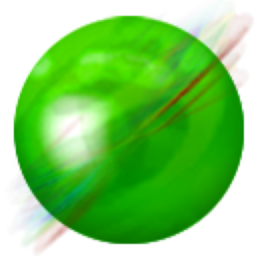
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
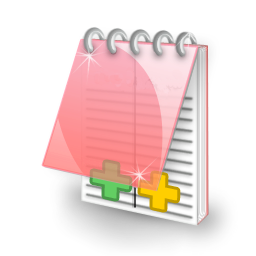
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
