In PHP, an object is a special type of variable that can store properties and methods. Arrays can store multiple elements, and each element can be a variable. In some cases, we may need to store objects in an array for processing. This article will introduce how PHP arrays store objects.
1. Create an object
First, let us create a simple PHP object to demonstrate how to store objects in an array. Suppose we need to create an object with the following properties:
class Person { public $name; public $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } public function getDetails() { return "Name: " . $this->name . " Age: " . $this->age; } } $person1 = new Person("John Doe", 30); $person2 = new Person("Jane Smith", 25);
In the above code, we have created a class called "Person" and created two objects $person1 and $person2, these objects Has name and age attributes and getDetails() method.
2. Storing Objects
Next, we will learn how to store these objects in PHP arrays. In PHP, you can use the following two methods to store objects in arrays:
Method 1: Store objects directly in arrays
In the following ways, we can combine the above two Objects are stored in arrays:
$people = array($person1, $person2); // 访问数组中的对象属性和方法 echo $people[0]->name; // 输出 "John Doe" echo $people[1]->getDetails(); // 输出 "Name: Jane Smith Age: 25"
In the above code, we use the array() function to create a $people array, which contains $person1 and $person2 objects. The elements in this array can be accessed through subscript values. For example, $people[0]->name will output the name attribute value "John Doe" of the $person1 object.
Method 2: Use key-value pairs to store objects
You can also use key-value pairs to store objects in arrays. For example, to store the $person1 object in the $people array with the key "p1":
$people = array("p1"=>$person1);
This will create an associative array with the key name "p1" and the key value being the $person1 object. We can access the properties and methods of this object in the following ways:
echo $people["p1"]->name; // 输出 "John Doe" echo $people["p1"]->getDetails(); // 输出 "Name: John Doe Age: 30"
$people["p1"] is used here to access the $person1 object. Likewise, we can store the $person2 object in the $people array.
3. Delete objects
When the objects stored in the array are no longer needed, you can use the unset() function to delete them from the array. For example, we want to remove the $person2 object from the $people array:
unset($people[1]); // 从数组中删除第二个元素
This will remove the second element (i.e. the $person2 object) from the $people array. We can then use the count() function to view the number of elements in the $people array:
echo count($people); // 输出 1
Here the number of elements in the $people array will be output, which is 1.
Summary
The key to storing objects is to use the correct method. Whether you store objects directly in an array or use key-value pair storage, you need to know how to access objects in the array. When no longer needed, use the unset function to remove it from the array to ensure optimal performance. Hopefully this article helped you better understand the way PHP arrays store objects.
The above is the detailed content of How to store objects in php array. For more information, please follow other related articles on the PHP Chinese website!
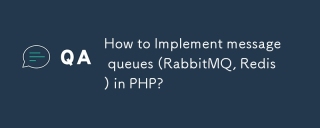
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
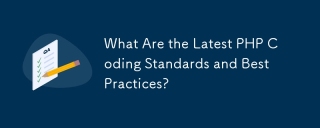
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
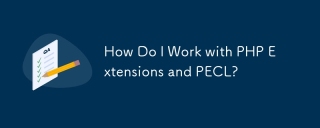
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
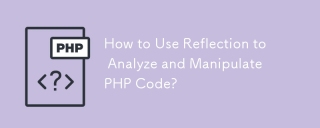
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
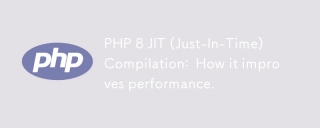
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
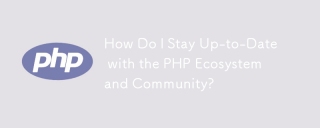
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
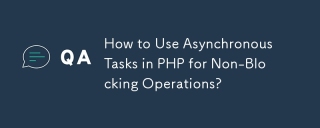
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
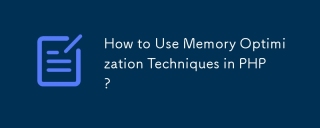
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
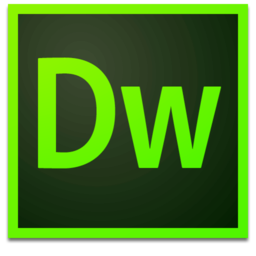
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
