In PHP, arrays are a very common data type, and the values in them are often used for calculations and operations. One common operation is to add values in an array. This article will introduce how to add values using PHP arrays.
- foreach loop
Use foreach loop to traverse the array, then accumulate all the values in the array, and finally return the added result. The code example is as follows:
<?php $numbers = array(1, 2, 3, 4, 5); $sum = 0; foreach ($numbers as $number) { $sum += $number; } echo $sum; ?>
The output result is: 15.
- array_sum function
You can use the PHP built-in function array_sum, which can calculate the sum of all values in an array. The code example is as follows:
<?php $numbers = array(1, 2, 3, 4, 5); echo array_sum($numbers); ?>
The output result is: 15.
- array_reduce function
Use the PHP built-in function array_reduce, which can accumulate the values in the array through the callback function. The code example is as follows:
<?php $numbers = array(1, 2, 3, 4, 5); $sum = array_reduce($numbers, function($carry, $item) { $carry += $item; return $carry; }); echo $sum; ?>
The output result is: 15.
- array_walk function
Use the PHP built-in function array_walk, which can apply a user-defined function to each element in the array. You can use this function to add arrays. The code example is as follows:
<?php $numbers = array(1, 2, 3, 4, 5); $sum = 0; array_walk($numbers, function($item) use(&$sum) { $sum += $item; }); echo $sum; ?>
The output result is: 15.
- for loop
Use the for loop to traverse the elements in the array, accumulate all the values in the array, and finally return the added result. The code example is as follows:
<?php $numbers = array(1, 2, 3, 4, 5); $sum = 0; for ($i = 0; $i < count($numbers); $i++) { $sum += $numbers[$i]; } echo $sum; ?>
The output result is: 15.
Summary
The above five methods can all use PHP arrays to add values. Depending on the actual usage, you can choose different methods. In actual development, the most suitable method can be selected to implement value addition based on the size of the array and the performance requirements of the calculation.
The above is the detailed content of How to add values in php arrays. For more information, please follow other related articles on the PHP Chinese website!
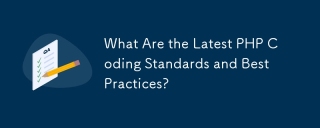
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
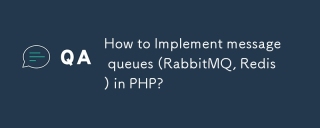
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
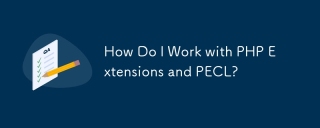
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
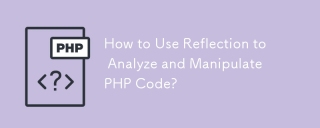
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
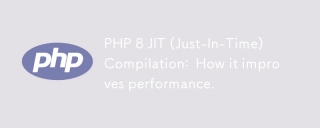
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
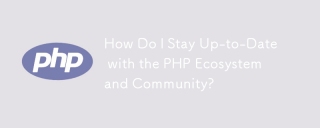
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
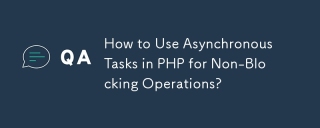
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
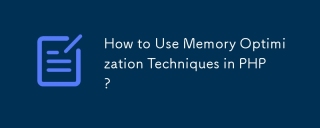
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
