In PHP development, an object array usually refers to a collection of objects containing different attributes and data. These objects can be instances defined as classes, or result sets returned from database query statements. In some cases, we need to convert these object arrays into ordinary arrays for easier operation and transmission. This article will introduce in detail how PHP implements the conversion of object arrays.
1. Object array in PHP
In PHP, the implementation of object array can be diversified. Let’s give an example.
- Array object
Array object is an object type variable defined as an array. There is no class relationship between these objects. For example, below we define an array object:
$user1 = array( 'id' => 1, 'username' => 'user1', 'email' => 'user1@example.com' ); $user2 = array( 'id' => 2, 'username' => 'user2', 'email' => 'user2@example.com' ); $users = array($user1, $user2);
In the above code, we define two array objects $user1 and $user2 respectively, and use the array() function to include them in an array $users middle.
- Class object
Class object is an object that defines a class. These objects have an inheritance relationship with the class and can use the properties and methods of the class. For example, below we define a User class and create several objects of this class:
class User { public $id; public $username; public $email; public function __construct($id, $username, $email) { $this->id = $id; $this->username = $username; $this->email = $email; } } $user1 = new User(1, 'user1', 'user1@example.com'); $user2 = new User(2, 'user2', 'user2@example.com'); $users = array($user1, $user2);
In the above code, we define a User class and use the __construct() function to create $user1 and $user2 two objects of this class and use the array() function to include them in an array $users.
- Database result set object
The database result set object is the data result obtained from the database using a query statement. The result is an array of similar objects. For example, below we use the mysqli method to query the database and obtain the result set object:
$conn = new mysqli($servername, $username, $password, $dbname); $sql = "SELECT * FROM users"; $result = $conn->query($sql); $users = array(); if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { $user = new User($row["id"], $row["username"], $row["email"]); array_push($users, $user); } }
In the above code, we use the mysqli method to query the database and assign the returned result set object to the $result variable , and then we use a while loop to convert the query results into an object array of User class.
2. Convert object array to ordinary array
In some cases, we need to convert object array to ordinary array to facilitate operation and transmission. PHP comes with many functions to implement this process, we will introduce them one by one.
- Use the json_encode function to convert
The json_encode function is a function that comes with PHP. It can convert arrays, objects and other data types into JSON format data. Here, we can get the ordinary array we want by converting the object array into JSON format data, and then converting the JSON data into an array. The following is an example:
$json_users = json_encode($users); //将对象数组转换为JSON格式数据 $users_arr = json_decode($json_users, true); //将JSON格式数据转换为数组
In the above code, we use the json_encode function to convert the object array $users into JSON format data and assign it to the $json_users variable. Next, we use the json_decode function to convert the JSON format data in the $json_users variable into an associative array and assign it to the $users_arr variable. In this way, the $users_arr variable holds the ordinary array form of the object array $users.
- Use foreach loop conversion
The foreach loop can help us operate on each element in the array, and it can also help us convert an object array into a normal array.
$users_arr = array(); foreach($users as $user) { $user_arr = array( 'id' => $user->id, 'username' => $user->username, 'email' => $user->email ); array_push($users_arr, $user_arr); }
In the above code, we traverse each element in the $users object array through a foreach loop, save its attributes and data in an associative array $user_arr, and use the array_push function to push each $user_arr The array is added to the $users_arr array. Ultimately, the $users_arr array is an ordinary array that contains all the data in the $users object array.
- Use the array_map function to convert
The array_map function is a function that comes with PHP. It will apply the specified function to each element of the array and return a new array. The following is an example:
function obj_to_arr($obj) { $result = array(); foreach ($obj as $key => $val) { if (is_object($val)) { $result[$key] = obj_to_arr($val); } else { $result[$key] = $val; } } return $result; } $users_arr = array_map('obj_to_arr', $users);
In the above code, we first define a function obj_to_arr to convert the object into an array. This function applies the specified object to each element in the array and returns a new array. Finally, we use the array_map function to apply the obj_to_arr function to each element in the $users object array and assign the result to the $users_arr array.
3. Summary
In PHP development, object array is one of the common data types, and processing and operating it is an indispensable part of the development process. In this article, we introduce in detail how to define object arrays in PHP and provide three ways to convert them into ordinary arrays. The above methods are very simple and easy to understand, and I hope they can be helpful to PHP developers.
The above is the detailed content of How to convert object array in PHP. For more information, please follow other related articles on the PHP Chinese website!
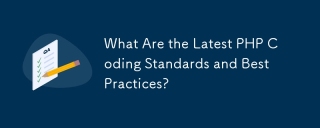
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
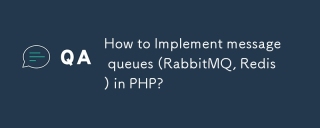
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
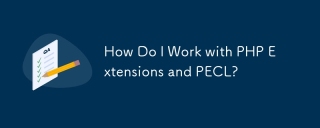
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
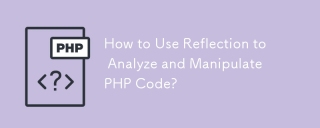
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
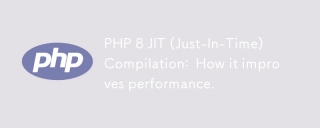
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
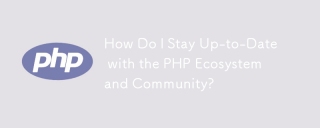
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
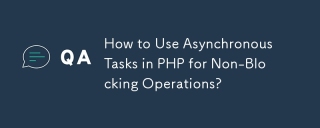
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
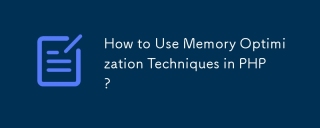
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
