PHP is a very popular open source server-side scripting language that is widely used to develop web applications. In PHP, sorting is a very important operation, which can help us process data quickly and accurately.
In PHP, we usually use built-in functions or array methods to sort arrays. However, in this article, I want to discuss another way of sorting without using PHP’s built-in functions and array methods.
In PHP, we can use comparison-based sorting algorithm to sort. These algorithms rely on comparing the size relationship between two elements and then placing them in a certain order. This sorting method is very effective, but it requires the use of functions or array methods to implement.
However, we also have an algorithm called non-comparison sorting. These algorithms can sort without comparing the size relationship between two elements, so they are faster and more memory-efficient than comparison-based sorting algorithms.
One of the non-comparison sorting algorithms is counting sort. This sorting algorithm determines the position of each element in the sort based on its value. The value of each element is how many elements before it are smaller than it in the sorting. We can then use a temporary array to store the number of times each value occurs, and then determine the position of each element based on the count array.
Here is a sample code:
function countingSort($arr) { $maxVal = max($arr); $count = array_fill(0, $maxVal + 1, 0); $output = array_fill(0, count($arr), 0); foreach ($arr as $val) { $count[$val]++; } for ($i = 1; $i = 0; $i--) { $output[$count[$arr[$i]] - 1] = $arr[$i]; $count[$arr[$i]]--; } return $output; }
In counting sort, we first find the largest element in the array and then create a counting array. Next, we loop through the entire array and record the number of occurrences of each element in the count array. Then create an output array and fill it with the values in the count array. Finally, the output array is returned as the sorted result.
Using counting sorting is much faster than sorting methods using PHP built-in functions and array methods. The time complexity of this method is O(n k), where n is the number of elements and k is the maximum value of the elements.
In short, although PHP provides many built-in functions and array methods for sorting operations, using a non-comparative sorting algorithm can be more efficient and save memory. Counting sort is one of the very useful algorithms that can help us sort an array quickly.
The above is the detailed content of How to sort array in php without using function. For more information, please follow other related articles on the PHP Chinese website!
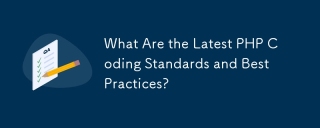
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
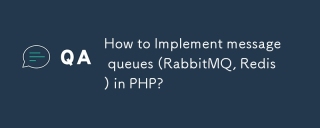
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
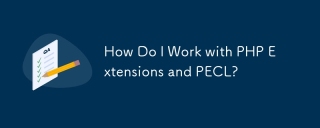
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
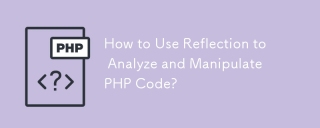
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
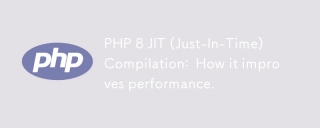
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
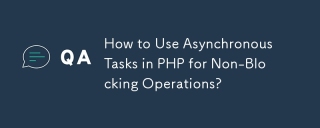
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
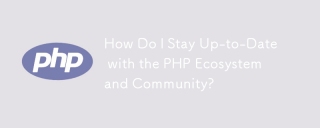
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
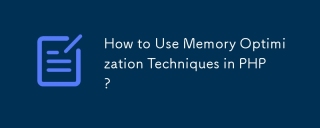
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
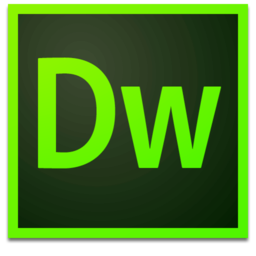
Dreamweaver Mac version
Visual web development tools
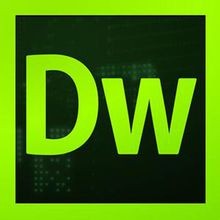
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
