In PHP development, array is a very important data type. It can hold an array of values and access them through array indexing. Sometimes, we need to get a specific value from a one-dimensional array. Below, we will explore how to get the values in a one-dimensional array in PHP.
- Using array index
The first method is to use array index. In a one-dimensional array, each value has a unique index. We can use these indexes to get the values in the array. For example, if we have an array $numbers, it contains the following values:
$numbers = array(1, 2, 3, 4, 5);
To get the third element ( For the value with index 2), we can use the following code:
$thirdElement = $numbers[2];
This code will set $thirdElement to 3. Likewise, we can use other indexes to get different values.
- Use the array_pop function
The second method is to use the PHP built-in function array_pop. This function removes an element from the end of the array and returns its value. For example, if we have the following array:
$colors = array("red", "green", "blue");
We can use array_pop to get the last color:
$lastColor = array_pop($colors);
In this example, $lastColor will be set to "blue" and the $colors array will contain "red" and "green".
- Use array_shift function
The third method is to use PHP's built-in function array_shift. This function removes an element from the beginning of the array and returns its value. Likewise, we can use this method to get the value of the first element. For example, if we have the following array:
$months = array("January", "February", "March");
We can use array_shift to get the first month:
$firstMonth = array_shift($months);
In this example, $firstMonth will be set to "January" and the $months array will contain "February" and "March".
- Use foreach loop
The fourth method is to use foreach loop. This method accesses each value in the array one by one and performs some operation. For example, if we have the following array:
$names = array("Peter", "John", "Mary");
We can use a foreach loop to output each name:
foreach($names as $name){
echo $name . "
";
}
This code will output:
Peter
John
Mary
If we only want to get the first element in the array, we can use the break statement to stop the loop. For example:
foreach($names as $name){
$first = $name;
break;
}
In this example, $first will be Set to "Peter".
Summary
In PHP, there are many ways to get the values in a one-dimensional array. We can use array indexing to access a specific value or we can use some built-in functions to get the first or last element of an array. In addition, using a foreach loop is also a very convenient method. Regardless of the method, you need to choose the most appropriate method based on the structure and data of the array.
The above is the detailed content of How to get values in one dimensional array in PHP. For more information, please follow other related articles on the PHP Chinese website!
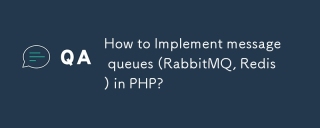
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
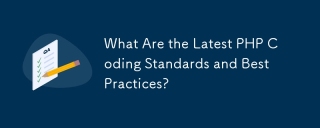
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
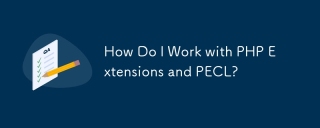
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
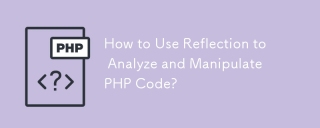
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
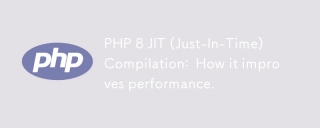
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
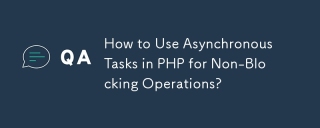
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
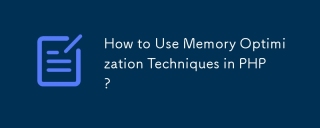
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
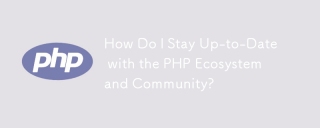
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
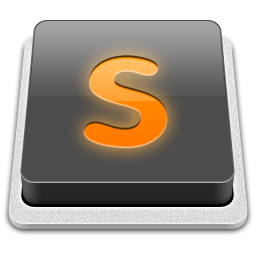
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.