When using Laravel queue, we may encounter task execution failure. At this time, Laravel provides a good solution, which is to use a failure queue.
When we execute queue tasks, we can push failed tasks into a queue specially designed to handle failed tasks, and then regularly check this queue and re-execute the failed tasks in it. In this way, we can avoid the queue tasks from being terminated due to some minor problems and continue to serve us.
Laravel's failure queue provides a variety of configurations and extensible interfaces, which we can configure according to our own needs.
First, we need to set the queue that failed tasks should enter in the configuration file config/queue.php
. The key of this configuration item is failed
, and its value is an array, which contains two configuration items: driver
and queue
. The driver
configuration item indicates which failure driver we want to use. Laravel provides two failure drivers by default, database
and redis
. And queue
indicates which queue the failed task will enter.
'failed' => [ 'driver' => 'database', 'queue' => 'failed', ],
If we want to use other failure drivers, we can do so by registering a custom failure driver and referencing it in the driver
configuration item.
The code to register a custom driver is as follows:
Queue::failing(function ($connection, $job, $data) { // 自定义处理逻辑 });
Next, we need to define the specific logic for handling failed tasks. We can directly push the failed task into the queue again, so that the task will be executed again during the next queue processing. Alternatively, we can also store some information about failed tasks for subsequent inspection and processing.
For the processing method of pushing the failed task into the queue, we can use the following code:
Queue::failing(function ($connection, $job, $data) { $queue = $job->getQueue(); $payload = $job->payload(); Queue::pushRaw($payload, $queue); });
This code pushes the failed task back to the original queue, waiting to be executed next time.
As for how to store failed task information, we can use the following code:
Queue::failing(function ($connection, $job, $data) { // 将失败任务信息存储到数据库中 DB::table('failed_jobs')->insert([ 'connection' => $connection, 'queue' => $job->getQueue(), 'payload' => $job->getRawBody(), 'exception' => $data['exception'], 'failed_at' => now(), ]); });
This code stores failed task information into the database table failed_jobs
, so that we can check and process it later.
In addition to the above two processing methods, Laravel also provides more processing methods for us to choose from. We can check out Laravel's documentation and source code for more details.
Finally, we need to periodically check the failure queue and retry the tasks in it. Laravel provides the queue:retry
command by default to retry tasks. This command accepts an optional parameter --queue
, which represents the task queue we want to retry. If this parameter is not specified, all queues will be retried by default.
php artisan queue:retry 5 --queue=my-queue
This command will re-execute the first 5 failed tasks from the my-queue
queue in the failure queue.
In addition, we can also use the operating system's scheduled task tool (such as crontab) to periodically execute the queue:retry
command to handle failed tasks regularly.
In short, Laravel's failure queue provides a very useful solution to handle the failure of queue tasks. We can configure it according to our own needs and handle failed tasks in many different ways. At the same time, we also need to regularly check the failure queue and retry the tasks in it to ensure that the queue tasks can run normally.
I hope this article will be helpful to everyone.
The above is the detailed content of What happens when laravel queue fails?. For more information, please follow other related articles on the PHP Chinese website!
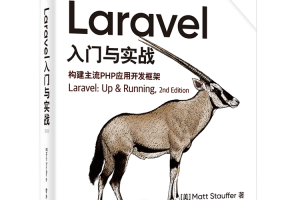
Integrating Sentry and Bugsnag in Laravel can improve application stability and performance. 1. Add SentrySDK in composer.json. 2. Add Sentry service provider in config/app.php. 3. Configure SentryDSN in the .env file. 4. Add Sentry error report in App\Exceptions\Handler.php. 5. Use Sentry to catch and report exceptions and add additional context information. 6. Add Bugsnag error report in App\Exceptions\Handler.php. 7. Use Bugsnag monitoring
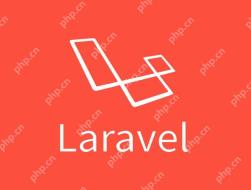
Laravel remains the preferred framework for PHP developers as it excels in development experience, community support and ecosystem. 1) Its elegant syntax and rich feature set, such as EloquentORM and Blade template engines, improve development efficiency and code readability. 2) The huge community provides rich resources and support. 3) Although the learning curve is steep and may lead to increased project complexity, Laravel can significantly improve application performance through reasonable configuration and optimization.
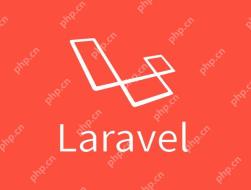
Building a live chat application in Laravel requires using WebSocket and Pusher. The specific steps include: 1) Configure Pusher information in the .env file; 2) Set the broadcasting driver in the broadcasting.php file to Pusher; 3) Subscribe to the Pusher channel and listen to events using LaravelEcho; 4) Send messages through Pusher API; 5) Implement private channel and user authentication; 6) Perform performance optimization and debugging.
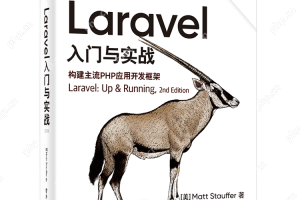
In Laravel, Redis and Memcached can be used to optimize caching policies. 1) To configure Redis or Memcached, you need to set connection parameters in the .env file. 2) Redis supports a variety of data structures and persistence, suitable for complex scenarios and scenarios with high risk of data loss; Memcached is suitable for quick access to simple data. 3) Use Cachefacade to perform unified cache operations, and the underlying layer will automatically select the configured cache backend.
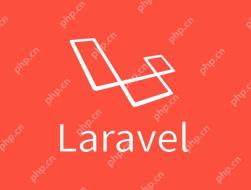
The steps to build a Laravel environment on different operating systems are as follows: 1.Windows: Use XAMPP to install PHP and Composer, configure environment variables, and install Laravel. 2.Mac: Use Homebrew to install PHP and Composer and install Laravel. 3.Linux: Use Ubuntu to update the system, install PHP and Composer, and install Laravel. The specific commands and paths of each system are different, but the core steps are consistent to ensure the smooth construction of the Laravel development environment.
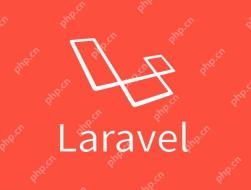
The main differences between Laravel and Yii are design concepts, functional characteristics and usage scenarios. 1.Laravel focuses on the simplicity and pleasure of development, and provides rich functions such as EloquentORM and Artisan tools, suitable for rapid development and beginners. 2.Yii emphasizes performance and efficiency, is suitable for high-load applications, and provides efficient ActiveRecord and cache systems, but has a steep learning curve.
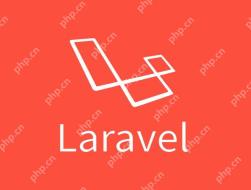
Laravel is suitable for developing e-commerce systems because it can quickly build efficient systems and provide an artistic development experience. 1) Product management realizes CRUD operation and classification association through EloquentORM. 2) Payment integration handles payment requests and exceptions through Stripe API to ensure the security and reliability of the payment process.
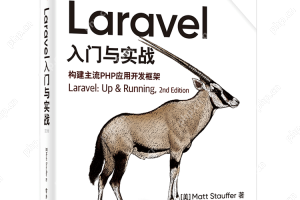
The essential Laravel extension packages for 2024 include: 1. LaravelDebugbar, used to monitor and debug code; 2. LaravelTelescope, providing detailed application monitoring; 3. LaravelHorizon, managing Redis queue tasks. These expansion packs can improve development efficiency and application performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
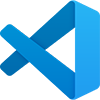
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
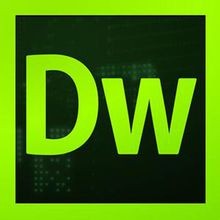
Dreamweaver CS6
Visual web development tools
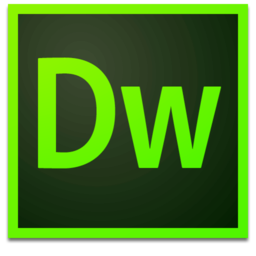
Dreamweaver Mac version
Visual web development tools
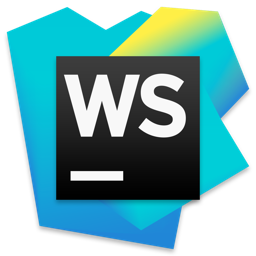
WebStorm Mac version
Useful JavaScript development tools
