Laravel logs and error monitoring: Sentry and Bugsnag integration
Integrating Sentry and Bugsnag in Laravel can improve application stability and performance. 1. Add Sentry SDK in composer.json. 2. Add Sentry service provider in config/app.php. 3. Configure Sentry DSN in the .env file. 4. Add Sentry error report in App\Exceptions\Handler.php. 5. Use Sentry to catch and report exceptions and add additional context information. 6. Add Bugsnag error report in App\Exceptions\Handler.php. 7. Use Bugsnag to monitor performance. Through these steps, you can effectively monitor and optimize errors and performance of your Laravel application.
introduction
In modern web development, logging and error monitoring are key to ensuring application stability and user experience. Today, we will dive into how to integrate Sentry and Bugsnag into the Laravel framework. With this article, you will learn how to easily integrate these tools into your Laravel project and gain valuable error insights from them, thereby improving application reliability and performance.
Review of basic knowledge
Before we begin, let's briefly review the basic concepts of logging and error monitoring. In Laravel, logs are implemented through the Monolog library, allowing developers to record various levels of information, such as debug, info, warning, error, etc. Error monitoring goes a step further, not only recording errors, but also providing real-time notifications, error trend analysis and performance monitoring. Sentry and Bugsnag are typical examples of such error monitoring tools that help developers quickly discover, diagnose and resolve problems in their applications.
Core concept or function analysis
Definition and function of Sentry and Bugsnag
Sentry and Bugsnag are tools specifically used for error monitoring. They can catch exceptions in applications, record detailed error information, and notify developers through email, Slack and other channels. Sentry is particularly good at providing detailed error stack traces and context information, while Bugsnag is known for its intuitive user interface and powerful performance monitoring capabilities. They all support multiple programming languages and frameworks, including Laravel.
Let's look at a simple Sentry integration example:
// Add Sentry SDK in your composer.json "require": { "sentry/sentry-laravel": "^2.0" } // Add Sentry service provider 'providers' => [ \Sentry\Laravel\ServiceProvider::class, ], // Configure Sentry DSN in .env file SENTRY_LARAVEL_DSN=your_sentry_dsn_here // Add Sentry error report in App\Exceptions\Handler.php public function report(Exception $exception) { if (app()->bound('sentry') && $this-> shouldReport($exception)) { app('sentry')->captureException($exception); } parent::report($exception); }
This example shows how to quickly integrate Sentry in Laravel. With a few lines of configuration, you can start monitoring errors in your application.
How it works
Sentry and Bugsnag work similarly, they catch exceptions by installing the SDK in the app. When an error occurs in the application, the SDK will automatically collect error information, including stack traces, user information, environment variables, etc., and send it to Sentry or Bugsnag's server for processing and storage. Developers can then view and analyze these error data through their web interface to quickly locate problems.
In terms of performance, Sentry and Bugsnag are both optimized to minimize the impact on application performance. They usually send error data asynchronously in the background to avoid blocking the normal operation of the application. However, it is worth noting that excessive error reporting may have some impact on application performance, so the threshold for error reporting needs to be properly configured in production environments.
Example of usage
Basic usage of Sentry
Let's see how to use Sentry in Laravel to log a manually thrown exception:
use Sentry\State\Scope; try { // Some code that may throw exception throw new \Exception('Something went wrong!'); } catch (\Exception $e) { //Catch exception and report it to Sentry app('sentry')->captureException($e); // Add extra context information app('sentry')->configureScope(function (Scope $scope): void { $scope->setUser(['id' => auth()->id()]); $scope->setExtra('custom', ['key' => 'value']); }); }
In this example, we manually catch an exception and report it to Sentry. At the same time, we have added some additional context information such as user ID and custom data, which helps analyze errors in more detail.
Advanced usage of Bugsnag
Bugsnag also provides a wealth of features, let's see how to use its advanced features to monitor the performance of Laravel applications:
use Bugsnag\Bugsnag; use Bugsnag\Handler; // Add Bugsnag error report in App\Exceptions\Handler.php public function report(Exception $exception) { if (app()->bound('bugsnag') && $this-> shouldReport($exception)) { app('bugsnag')->notifyException($exception); } parent::report($exception); } // Use Bugsnag to monitor performance in a controller public function index() { Bugsnag::startSpan('my_custom_span'); // Some time-consuming operations sleep(2); Bugsnag::finishSpan('my_custom_span'); return view('welcome'); }
In this example, we not only use Bugsnag to report errors, but also use its performance monitoring capabilities to track the execution time of an operation. This is very helpful in optimizing application performance.
Common Errors and Debugging Tips
There are some common problems you may encounter when using Sentry and Bugsnag. For example, error reports may fail due to network problems, or some sensitive information is accidentally sent to the monitoring platform. Here are some debugging tips:
- Network problem : Make sure your application server has access to Sentry or Bugsnag's servers. If you encounter network problems, you can consider cache error reports locally and wait until the network is restored before sending them.
- Sensitive information leakage : When configuring Sentry or Bugsnag, make sure to filter out sensitive information, such as user passwords, API keys, etc. You can set filtering rules in the configuration file to prevent this information from being sent.
- Too many bug reports : If your application produces a large number of bug reports, it may have a performance impact. You can set a threshold for error reporting, or use the sampling rate to reduce the number of reports.
Performance optimization and best practices
In practical applications, how to optimize the use of Sentry and Bugsnag? Here are some suggestions:
- Sampling of Error Reports : To reduce the impact on application performance, you can set the sampling rate of Error Reports. For example, only 10% of the errors are reported. This greatly reduces the number of error reports while still being able to catch most errors.
- Performance Monitoring : Use Sentry and Bugsnag's performance monitoring functions to regularly check the performance bottlenecks of applications. By analyzing performance data, you can discover which operations take longer and optimize them.
- Code readability : Make sure your code remains readable when using Sentry and Bugsnag. Add appropriate comments and documentation to help other developers understand the logic of bug reporting and performance monitoring.
- Error classification : Classify errors so that problems can be analyzed and resolved more easily. For example, errors can be classified according to dimensions such as error type, module, user, etc., so that problems can be located faster.
In short, Sentry and Bugsnag are powerful error monitoring tools that can help you better manage and optimize Laravel applications. With the introduction and examples of this article, you should have mastered how to integrate and use these tools in Laravel. I hope this knowledge can help you improve the stability and performance of your application in actual development.
The above is the detailed content of Laravel logs and error monitoring: Sentry and Bugsnag integration. For more information, please follow other related articles on the PHP Chinese website!

Taskmanagementtoolsareessentialforeffectiveremoteprojectmanagementbyprioritizingtasksandtrackingprogress.1)UsetoolslikeTrelloandAsanatosetprioritieswithlabelsortags.2)EmploytoolslikeJiraandMonday.comforvisualtrackingwithGanttchartsandprogressbars.3)K

Laravel10enhancesperformancethroughseveralkeyfeatures.1)Itintroducesquerybuildercachingtoreducedatabaseload.2)ItoptimizesEloquentmodelloadingwithlazyloadingproxies.3)Itimprovesroutingwithanewcachingsystem.4)ItenhancesBladetemplatingwithviewcaching,al

The best full-stack Laravel application deployment strategies include: 1. Zero downtime deployment, 2. Blue-green deployment, 3. Continuous deployment, and 4. Canary release. 1. Zero downtime deployment uses Envoy or Deployer to automate the deployment process to ensure that applications remain available when updated. 2. Blue and green deployment enables downtime deployment by maintaining two environments and allows for rapid rollback. 3. Continuous deployment Automate the entire deployment process through GitHubActions or GitLabCI/CD. 4. Canary releases through Nginx configuration, gradually promoting the new version to users to ensure performance optimization and rapid rollback.

ToscaleaLaravelapplicationeffectively,focusondatabasesharding,caching,loadbalancing,andmicroservices.1)Implementdatabaseshardingtodistributedataacrossmultipledatabasesforimprovedperformance.2)UseLaravel'scachingsystemwithRedisorMemcachedtoreducedatab

Toovercomecommunicationbarriersindistributedteams,use:1)videocallsforface-to-faceinteraction,2)setclearresponsetimeexpectations,3)chooseappropriatecommunicationtools,4)createateamcommunicationguide,and5)establishpersonalboundariestopreventburnout.The

LaravelBladeenhancesfrontendtemplatinginfull-stackprojectsbyofferingcleansyntaxandpowerfulfeatures.1)Itallowsforeasyvariabledisplayandcontrolstructures.2)Bladesupportscreatingandreusingcomponents,aidinginmanagingcomplexUIs.3)Itefficientlyhandleslayou

Laravelisidealforfull-stackapplicationsduetoitselegantsyntax,comprehensiveecosystem,andpowerfulfeatures.1)UseEloquentORMforintuitivebackenddatamanipulation,butavoidN 1queryissues.2)EmployBladetemplatingforcleanfrontendviews,beingcautiousofoverusing@i

Forremotework,IuseZoomforvideocalls,Slackformessaging,Trelloforprojectmanagement,andGitHubforcodecollaboration.1)Zoomisreliableforlargemeetingsbuthastimelimitsonthefreeversion.2)Slackintegrateswellwithothertoolsbutcanleadtonotificationoverload.3)Trel


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use
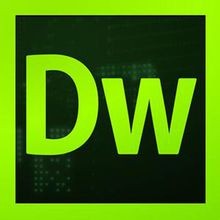
Dreamweaver CS6
Visual web development tools
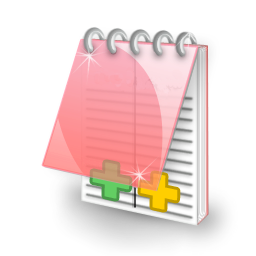
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
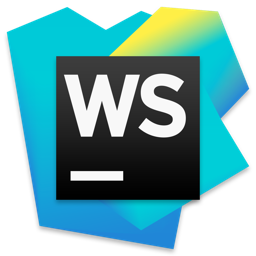
WebStorm Mac version
Useful JavaScript development tools
