Golang is a modern C-like programming language developed by Google and first released in 2009. It has the characteristics of simplicity, efficiency, and security, and is widely used in servers, network programming, cloud computing and other fields. In Golang, conversion between integers and strings is a very basic operation. This article introduces how to convert integer types to their corresponding string types in Golang.
1. Integer types in Golang
In Golang, integer types include signed integers and unsigned integers. Among them, signed integer types include int8, int16, int32, int64, etc.; unsigned integer types include uint8, uint16, uint32, uint64, etc.
The following is a simple example showing how to use integer types in Golang:
package main import ( "fmt" ) func main() { var x int = -10 var y uint = 20 fmt.Println(x) fmt.Println(y) }
In the above code, we define a variable x of type int with a value of -10. At the same time, we define a uint type variable y with a value of 20. In Golang, the int type defaults to a signed integer type, and the uint type defaults to an unsigned integer type. The types of x and y can be verified by printing their values.
2. Integer and string type conversion
In Golang, converting the integer type to the string type and converting the string type to the integer type are very common operations. Here, we focus on the process of converting an integer type to a string type.
In Golang, the easiest way to convert an integer type to a string type is to use the strconv.Itoa() function. Itoa represents converting the Int type into the Aci code (ASCII) character type. This function can convert signed integer types such as int, int8, int16, int32, etc. into string types. The following is an example of using the strconv.Itoa() function to convert the int type to the string type:
package main import ( "fmt" "strconv" ) func main() { var age int = 20 var ageStr string = strconv.Itoa(age) fmt.Println(age, ageStr) }
In the above code, we define a variable age of type int with a value of 20. We then use the strconv.Itoa() function to convert age to string type and store the result in the string variable ageStr. Finally, we print the values of age and ageStr.
Run the above code, the output result is:
20 20
Among them, the first 20 represents the age of the integer type, and the second 20 represents the ageStr of the string type. We can see that using the strconv.Itoa() function can easily convert the integer type to the string type.
3. Convert int64 type to string
In Golang, the int64 type is a very commonly used integer type because it can represent very large numbers. If you need to convert the int64 type to a string type, you can use the strconv.FormatInt() function. FormatInt is used for int64 and returns the string representation of the number in the specified base (2 to 36). For example:
package main import ( "fmt" "strconv" ) func main() { var num int64 = 1234567890 var numStr string = strconv.FormatInt(num, 10) fmt.Println(num, numStr) }
In the above code, we define a variable num of type int64 with a value of 1234567890. Then we convert num to string type using strconv.FormatInt() function and store the result in string variable numStr. Finally, we print the values of num and numStr.
Run the above code, the output result is:
1234567890 1234567890
Among them, the first 1234567890 represents the integer type num, and the second 1234567890 represents the string type numStr. We can see that the int64 type can be easily converted to a string type using the strconv.FormatInt() function.
4. Convert uint64 type to string
In Golang, the uint64 type is an unsigned integer type and is also a very commonly used integer type. If you need to convert the uint64 type to a string type, you can use the strconv.FormatUint() function. FormatUint is used for uint64 and returns the string representation of the number in the specified base (2 to 36). For example:
package main import ( "fmt" "strconv" ) func main() { var num uint64 = 1234567890 var numStr string = strconv.FormatUint(num, 10) fmt.Println(num, numStr) }
In the above code, we define a uint64 type variable num with a value of 1234567890. Then we convert num to string type using strconv.FormatUint() function and store the result in string variable numStr. Finally, we print the values of num and numStr.
Run the above code, the output result is:
1234567890 1234567890
Among them, the first 1234567890 represents the integer type num, and the second 1234567890 represents the string type numStr. We can see that the uint64 type can be easily converted to a string type using the strconv.FormatUint() function.
Summary
In Golang, converting the integer type to the string type is a very basic operation. In this article, we have introduced how to convert integer type to string type using functions such as strconv.Itoa(), strconv.FormatInt(), strconv.FormatUint(), etc. Whether in servers, network programming, cloud computing and other fields, these operations have a wide range of applications. I hope this article can help you better understand and apply integer and string type conversion in Golang.
The above is the detailed content of How to convert integer to string type in golang. For more information, please follow other related articles on the PHP Chinese website!
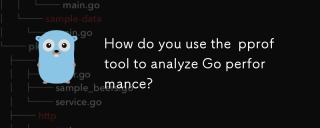
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
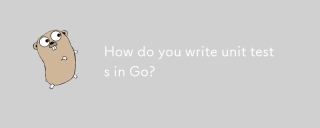
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
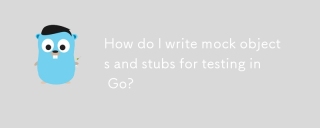
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
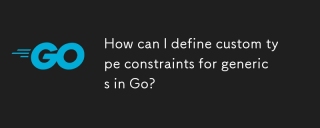
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
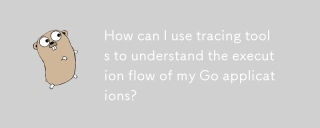
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
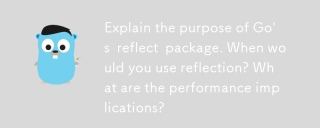
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
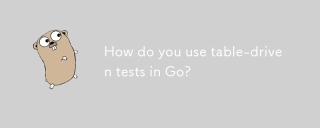
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
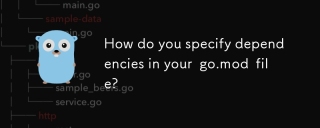
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
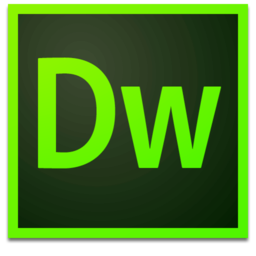
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
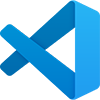
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
