With the continuous development and popularity of Web applications, the database has become the most critical part for developers. As a language widely used in web development, PHP also has very rich and powerful support for database operations. In PHP, we can add and delete folders in the database by writing corresponding code. This article will introduce the specific implementation process.
1. Preparation
Before starting to write PHP code, we need to install the necessary software and prepare the relevant environment. The specific steps are as follows:
- Install PHP and related extensions
First, we need to install PHP locally or on the server. Taking Windows as an example here, just download the corresponding PHP installation package and install it.
Next, you need to install some necessary PHP extensions, such as mysqli, PDO and other extensions. These extensions can be enabled by setting them in the php.ini configuration file. Under the Windows operating system, the php.ini file is usually located in \php\php.ini in the PHP installation directory.
- Install the database
Next, we need to install the database. For MySQL, you can download the corresponding installation package from the official website and install it.
After installing the database, you need to ensure that you can connect to the database through PHP code. The code example is as follows:
// Database connection configuration
$host = "localhost";
$username = "root";
$password = "" ;
$database = "test_db";
//Connect to the database
$conn = mysqli_connect($host, $username, $password, $database);
if (!$conn ) {
die("连接数据库失败: " . mysqli_connect_error());
} else {
echo "成功连接到数据库";
}
?>
In the above code, we can see that the MySQL database is connected through the mysqli_connect() function, And detect whether the connection is successful. If the connection is successful, "Successfully connected to the database" will be output. Otherwise, a specific error message will be output.
2. Adding folders
Next, we will introduce how to add folders in the database in PHP.
- Create a form to add a folder
First, we need to create an HTML form to add a folder to the database. The code example is as follows:
<meta> <title>添加文件夹</title>
In the above code, we created a form and specified the form submission address through the action attribute, which is add_folder.php page. The form contains an input box to accept the folder name entered by the user.
- Write PHP script
Next, we need to write a PHP script to add the folder name submitted by the user to the database. The code example is as follows:
// Database connection configuration
$host = "localhost";
$username = "root";
$password = "" ;
$database = "test_db";
//Accept data submitted by users
$folder_name = $_POST['folder_name'];
//Execute SQL statements
$sql = "INSERT INTO folders (name) VALUES ('$folder_name')";
if (mysqli_query($conn, $sql)) {
echo "新文件夹添加成功";
} else {
echo "添加新文件夹失败: " . mysqli_error($conn);
}
//Close the database connection
mysqli_close($conn);
?>
In the above code, we first pass $_POST['folder_name'] Get the folder name submitted by the user and then use the INSERT statement to add the folder name to the database. If the addition is successful, "New folder added successfully" is output, otherwise a specific error message is output. Finally, close the database connection.
3. Deleting folders
In addition to adding folders, we also need to delete folders in the database. The specific steps are as follows:
- Create a link to delete the folder
First, we need to create a link to delete the folder in the database. The code example is as follows:
In the above code, we create a link and pass The GET method passes the folder ID to be deleted to the delete_folder.php page.
- Write a PHP script
Next, we need to write a PHP script to delete the folder with the specified ID from the database. The code example is as follows:
// Database connection configuration
$host = "localhost";
$username = "root";
$password = "" ;
$database = "test_db";
//Accept passed parameters
$id = $_GET['id'];
//Execute SQL statement
$sql2 = "DELETE FROM folders WHERE id=$id";
if (mysqli_query($conn, $sql2)) {
echo "文件夹删除成功";
} else {
echo "文件夹删除失败: " . mysqli_error($conn);
}
// Close the database connection
mysqli_close($conn);
?>
In the above code, we obtain the passed folder ID through $_GET['id'], and use the DELETE statement to delete the folder from the database. If the deletion is successful, "Folder deletion successful" is output, otherwise a specific error message is output.
4. Summary
Through the introduction of this article, we have implemented the adding and deleting operations of folders in the database in PHP. Of course, this is just a simple example. In actual development, many aspects need to be considered, such as SQL injection, verification of input data, etc., which require in-depth understanding and practice by developers.
The above is the detailed content of How to add and delete folders in the database in php. For more information, please follow other related articles on the PHP Chinese website!
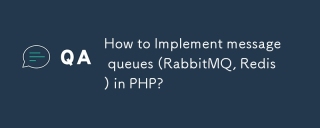
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
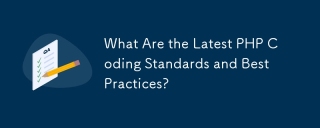
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
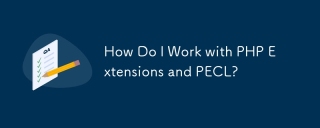
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
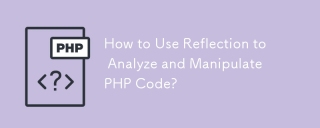
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
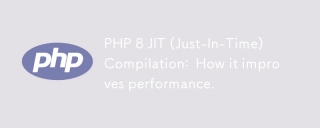
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
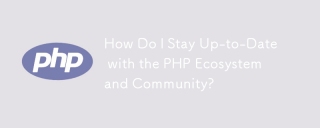
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
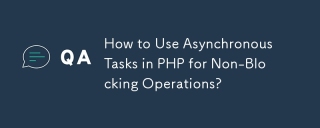
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
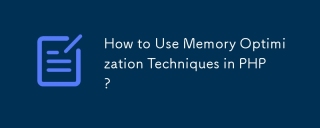
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
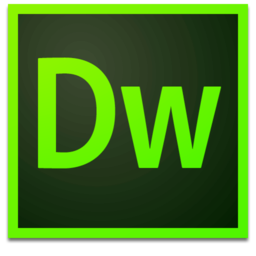
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor
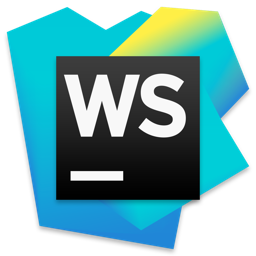
WebStorm Mac version
Useful JavaScript development tools
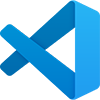
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
