PHP is an open source server-side scripting language that can run on a web server. As a powerful and free programming language, PHP has become an important part of web development. The PHP array is also one of the most commonly used data structures in PHP.
So, how to call an array in PHP?
- Create an array
In PHP, you can use the array() function to create an array. The function can accept any number of parameters, which will become elements of the array.
$colors = array("Red", "Blue", "Green");
The above code creates an array named $colors and uses three colors as elements of the array. You can use the print_r() function to output the contents of the array.
print_r($colors);
The output result is:
Array ( [0] => Red [1] => Blue [2] => Green )
- Accessing array elements
In PHP, you can use square brackets [] to access array elements.
echo $colors[0]; // 输出 Red echo $colors[1]; // 输出 Blue echo $colors[2]; // 输出 Green
In PHP, array indexing starts from 0, so $colors[0] is the first element of the array.
- Modify array elements
You can modify array elements in the same way.
$colors[1] = "Yellow"; print_r($colors);
The output result is:
Array ( [0] => Red [1] => Yellow [2] => Green )
The above code changes the second element of the $colors array from "Blue" to "Yellow".
- Traverse an array
In PHP, you can use a loop to traverse an array. Common methods include for loop and foreach loop.
// for循环 for ($i = 0; $i "; } // foreach循环 foreach ($colors as $color) { echo $color . "<br>"; }
The above code will iterate through all elements in the $colors array and output their values.
- Associative array
In addition to index arrays, PHP also supports associative arrays. An associative array is an array that maps keys to values. When creating an associative array, you can specify key names and key values.
$person = array("name" => "Tom", "age" => 26, "city" => "New York"); print_r($person);
The output result is:
Array ( [name] => Tom [age] => 26 [city] => New York )
Associative array elements can be accessed using key names.
echo $person["name"]; // 输出 Tom echo $person["age"]; // 输出 26 echo $person["city"]; // 输出 New York
Associative arrays can be traversed using a foreach loop.
foreach ($person as $key => $value) { echo $key . ": " . $value . "<br>"; }
The above code will traverse all elements in the $person array and output their key names and values.
In PHP, arrays are a flexible and powerful tool that can help developers organize and process data easily. Mastering the calling methods of PHP arrays is an important step in web development. Hope this article helps you!
The above is the detailed content of How to call array in PHP. For more information, please follow other related articles on the PHP Chinese website!
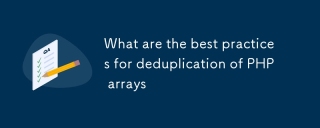
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
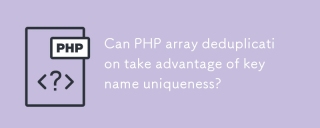
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
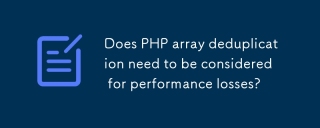
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
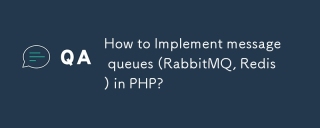
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
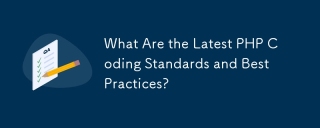
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
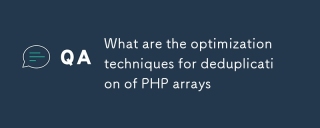
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
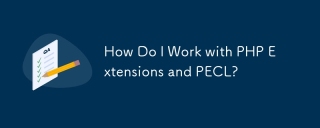
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
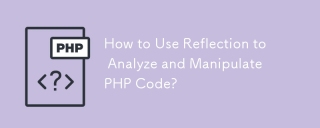
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
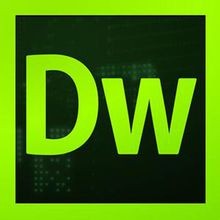
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
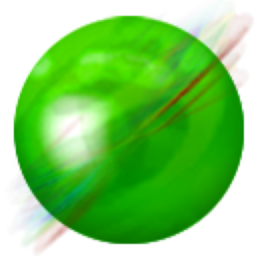
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
