PHP is a widely used and very popular open source programming language. In PHP, array is a very important data type used to store multiple values. This article will show you how to define an array and find its average.
Define Array
Defining an array in PHP is very simple. Here is a basic example:
<?php $number_array = array(2, 4, 6, 8, 10); ?>
The above code creates an array named $number_array
, which contains 5 elements: 2, 4, 6, 8, and 10.
Copy the above code into your local PHP environment, save it as a .php file and open it in the browser, you will see that there is no output on the page. This is because we haven't told PHP how to print this array. Here is a simple example:
<?php $number_array = array(2, 4, 6, 8, 10); print_r($number_array); ?>
In the above code, we have used the PHP built-in function print_r
to print the array. After running the above code, we will see the following output:
Array ( [0] => 2 [1] => 4 [2] => 6 [3] => 8 [4] => 10 )
The output here tells us that this array consists of 5 elements and each element has a unique index. In PHP, the index of an array can be a number or a string.
Find the average
Now that we have an array containing numbers, we can use a loop structure to iterate through it and calculate the average of these numbers. Here is a simple example:
<?php $number_array = array(2, 4, 6, 8, 10); $sum = 0; $count = count($number_array); for($i = 0; $i < $count; $i++) { $sum += $number_array[$i]; } $average = $sum / $count; echo "平均数是:" . $average; ?>
In the above code, we have used a loop to iterate through the array and add each number. We use the variable $sum
to store the sum of all numbers and the PHP built-in function count
to get the number of elements in the array. Once we have calculated the sum, we can calculate the average and then output it using the echo
statement.
After running the above code, we will see the following output:
平均数是:6
This tells us that the average of these numbers in the array is 6.
Conclusion
In this article, we have introduced how to define an array and calculate its average using PHP. Although this example is very simple, it can serve as the basis for more complex PHP applications. In real projects, arrays and loop structures are common tools in PHP programming, so it is very important to know how to operate on them.
The above is the detailed content of How to define an array and find the average in PHP. For more information, please follow other related articles on the PHP Chinese website!
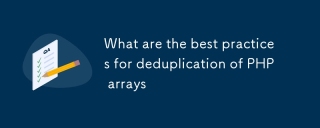
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
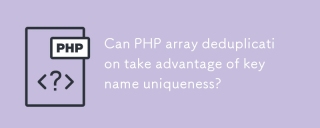
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
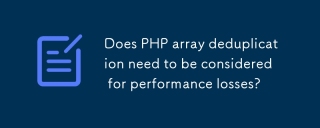
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
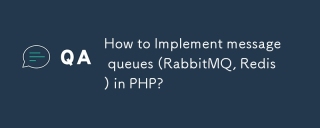
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
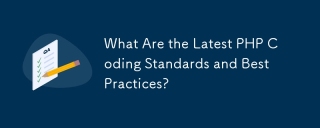
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
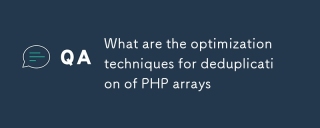
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
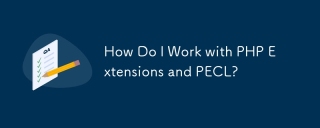
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
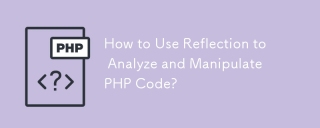
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
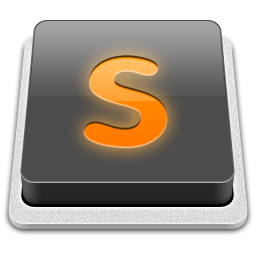
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
