PHP is a server-side scripting language. In PHP, an array is a set of data types that represent a sequence of ordered values. For an array, we may need to know its length, that is, the number of elements it contains. In this article, we will discuss how to determine the length of an array in PHP.
In PHP, there are several ways to determine the length of an array. Here are some of them:
Method 1: count() function
The count() function can be used to determine the number of elements in an array. This function accepts an array as argument and returns an integer containing the number of elements in the array.
The following is an example:
$myArray = array("apple", "banana", "pear"); $count = count($myArray); echo "The count of my array is " . $count;
In this example, we define an array containing three elements. We use the count() function to get the length of the array and print the result to the screen. Running this code will output:
The count of my array is 3
It is worth noting that the count() function is slower because it needs to traverse the entire array to determine its length.
Method 2: sizeof() function
The sizeof() function is an alias of the count() function. It has the same function as the count() function, which is used to return the number of elements in the array.
The following is an example:
$myArray = array("apple", "banana", "pear"); $size = sizeof($myArray); echo "The size of my array is " . $size;
In this example, we define an array containing three elements. We use the sizeof() function to get the length of the array and print the result to the screen. Running this code will output:
The size of my array is 3
Method 3: Use the count() or sizeof() function to replace the count keyword
In PHP, you can also use the count keyword to get the length of the array. The count keyword returns an integer representing the number of elements in the array.
The following is an example:
$myArray = array("apple", "banana", "pear"); $count = count($myArray); echo "The count of my array is " . $count;
In this example, we define an array containing three elements. We use the count() function to get the length of the array and print the result to the screen. Running this code will output:
The count of my array is 3
Unlike the count() function and the sizeof() function, the count keyword is faster because it does not need to traverse the entire array to determine its length. Of course, the count keyword can only be used to get the length of an array.
Summary
There are three ways to determine the length of a PHP array: count() function, sizeof() function and count keyword. Each method has its own advantages and disadvantages. Which method to use depends on your specific needs.
Hope this article is helpful to you!
The above is the detailed content of How to determine the length of an array in php. For more information, please follow other related articles on the PHP Chinese website!
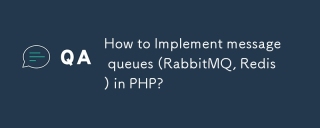
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
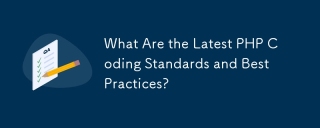
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
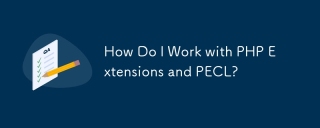
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
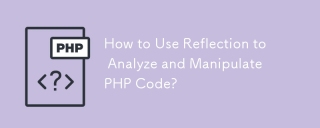
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
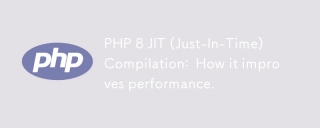
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
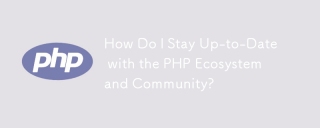
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
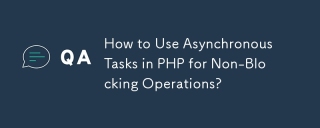
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
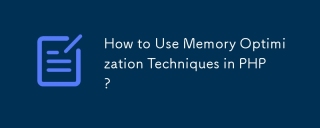
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
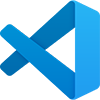
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
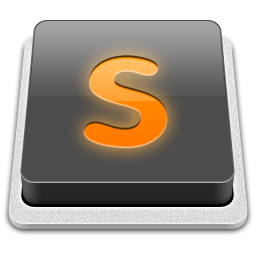
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
