In PHP, array is a very commonly used data structure. In actual development, we often need to loop through the array and modify the array. This article will explain how to use PHP to loop through an array and modify it.
- for loop traverses the array
The for loop is the most common loop statement. We can use a for loop to traverse the array and modify it.
The following is a simple example:
<?php $numbers = array(1, 2, 3, 4, 5); for($i = 0; $i < count($numbers); $i++) { $numbers[$i] = $numbers[$i] * 2; } print_r($numbers); ?>
The output result is:
Array ( [0] => 2 [1] => 4 [2] => 6 [3] => 8 [4] => 10 )
In the above code, we define an array $numbers containing five elements, and then Use a for loop to iterate through the array. In the loop, we multiply each element in the array by 2.
- foreach loop traverses the array
In addition to the for loop, we can also use the foreach loop to traverse the array. Unlike the for loop, the foreach loop can directly traverse each element of the array without defining a loop variable.
The following is an example of using a foreach loop to traverse an array:
<?php $numbers = array(1, 2, 3, 4, 5); foreach($numbers as &$value) { $value = $value * 2; } unset($value); print_r($numbers); ?>
The output result is:
Array ( [0] => 2 [1] => 4 [2] => 6 [3] => 8 [4] => 10 )
In the above code, we use a foreach loop to traverse the array $numbers, and Each element in the array is multiplied by 2. It should be noted that we use &$value in the foreach loop to refer to the address of the array element so that the array element can be modified.
- While loop traverses the array
In addition to the for loop and foreach loop, we can also use the while loop to traverse the array. In fact, using a while loop to iterate over an array is very similar to using a for loop to iterate over an array.
The following is an example of using a while loop to traverse an array:
<?php $numbers = array(1, 2, 3, 4, 5); $count = count($numbers); $i = 0; while($i < $count) { $numbers[$i] = $numbers[$i] * 2; $i++; } print_r($numbers); ?>
The output result is:
Array ( [0] => 2 [1] => 4 [2] => 6 [3] => 8 [4] => 10 )
In the above code, we defined a variable $count to store the array number of elements, and then use a while loop to traverse the array. In the loop, we multiply each element in the array by 2.
- Summary
In PHP, looping through an array and modifying it is a very common operation. We can use for loop, foreach loop, while loop and other methods to traverse the array and modify the array. It should be noted that during the process of traversing the array and making modifications, we need to ensure that we do not miss any array element, otherwise it will cause a logic error.
The above is the detailed content of How to loop through an array and modify it using PHP. For more information, please follow other related articles on the PHP Chinese website!
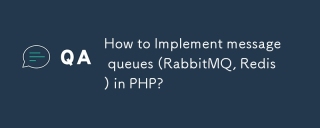
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
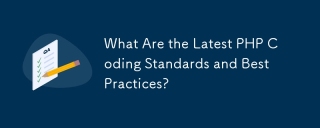
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
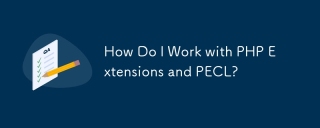
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
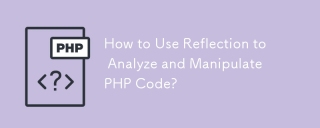
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
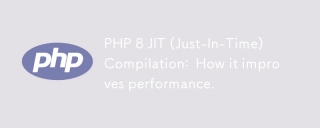
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
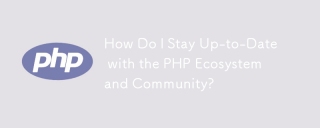
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
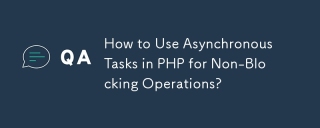
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
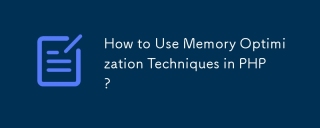
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
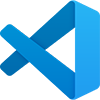
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
