PHP is a widely used programming language that is easy to use, open and efficient. In PHP, arrays are a very important data structure, and looping through arrays is also one of the basic operations in daily development. In this article, we will explore how to loop through an array in PHP.
Arrays in PHP
In PHP, an array is a collection used to store the same type of data. Arrays can store various types of data, including integers, floating point numbers, strings, Boolean values, etc. In PHP, arrays can be declared in the following ways:
//使用array()函数声明数组 $arr = array(1,2,3); //使用[]操作符声明数组 $arr = [1,2,3];
There are three types of arrays in PHP: indexed arrays, associative arrays, and multidimensional arrays.
1. Index array
Index array is the most commonly used array type. It is an array type that uses numeric indexing to access array elements. In PHP, you can declare an indexed array in the following way:
//使用array()函数声明索引数组 $arr = array(1,2,3); //使用[]操作符声明索引数组 $arr = [1,2,3];
2. Associative array
Associative array is an array type that uses key-value pairs to access array elements. In PHP, you can declare an associative array in the following way:
//使用array()函数声明关联数组 $arr = array("name"=>"Tom", "age"=>18); //使用[]操作符声明关联数组 $arr = ["name"=>"Tom", "age"=>18];
The keys and values in an associative array can be any type of data, and the keys must be unique. Elements in an associative array can be accessed using keys as follows:
echo $arr["name"]; //输出Tom echo $arr["age"]; //输出18
3. Multidimensional Array
A multidimensional array is an array type that contains one or more arrays. In PHP, multidimensional arrays can be declared using:
//使用array()函数声明多维数组 $arr = array( array(1,2,3), array(4,5,6), array(7,8,9) ); //使用[]操作符声明多维数组 $arr = [ [1,2,3], [4,5,6], [7,8,9] ];
In a multidimensional array, each array can be an array of any type or an array of the same type.
Looping through arrays in PHP
In PHP, looping through arrays is a common operation. Looping through an array allows us to easily obtain each element in the array and process it.
The following are several commonly used ways to loop through arrays in PHP:
1. Use a for loop to traverse the index array
If the array is an index array, you can use The for loop iterates through the array. The for loop needs to specify the number of loops to traverse the array, and the loop variable can obtain the array elements by index. The code is as follows:
$arr = array(1,2,3,4,5); $len = count($arr); for ($i=0; $i<p>2. Use foreach loop to traverse index arrays and associative arrays</p><p>If the array is an index array or associative array, then you can use foreach loop to traverse the array, this is in PHP One of the most commonly used ways to traverse an array. The foreach loop can automatically obtain array elements without specifying the number of loops, which is very convenient. The code is as follows: </p><pre class="brush:php;toolbar:false">//遍历索引数组 $arr = array(1,2,3,4,5); foreach ($arr as $value) { echo $value; } //遍历关联数组 $arr = array("name"=>"Tom", "age"=>18); foreach ($arr as $key=>$value) { echo $key.":".$value; }
3. Use a while loop to traverse the index array
Using a while loop to traverse an array requires obtaining the array length in advance, and then using the loop variable as an index to obtain the array elements. The code is as follows:
$arr = array(1,2,3,4,5); $len = count($arr); $i = 0; while ($i <p>4. Use do-while loop to traverse the index array</p><p>Using do-while loop to traverse the array is similar to using while loop, except that the loop body is executed first and then the condition is checked. The code is as follows: </p><pre class="brush:php;toolbar:false">$arr = array(1,2,3,4,5); $len = count($arr); $i = 0; do { echo $arr[$i]; $i++; } while ($i <p>5. Use the list() function to traverse the associative array</p><p>Using the list() function can easily assign the value of the associative array to a variable. The code is as follows: </p><pre class="brush:php;toolbar:false">$arr = array("name"=>"Tom", "age"=>18); list($name, $age) = $arr; echo $name; echo $age;
Summary
This article introduces the concept of arrays in PHP and how to use for loops, foreach loops, while loops, do-while loops and list() functions to traverse arrays. Depending on the type of array, different looping methods can be selected. Proficient in the skill of looping through arrays can greatly improve the efficiency of the program and speed up development.
The above is the detailed content of How to loop through an array in php. For more information, please follow other related articles on the PHP Chinese website!
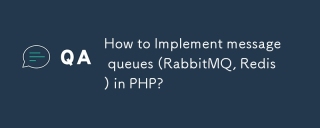
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
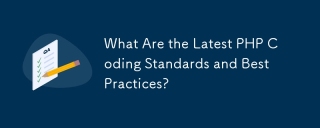
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
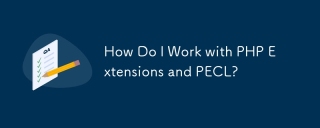
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
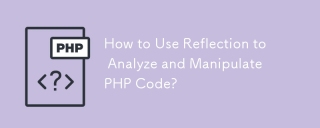
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
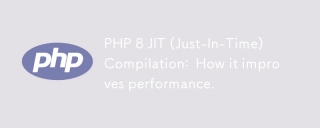
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
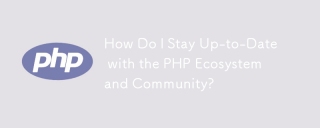
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
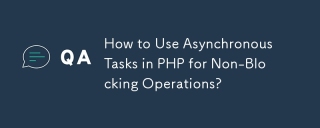
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
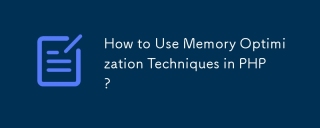
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
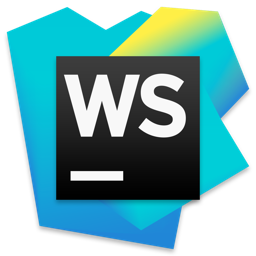
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
