Laravel is an open source web application framework based on PHP. It greatly simplifies common web development tasks such as routing, session management, authentication, and more. Laravel also provides a tool called Task Scheduler that allows users to easily execute program code at a specified time. This article will introduce Laravel's task scheduler and how to set up scheduled tasks.
What is the Laravel Task Scheduler
The task scheduler is a part of the Laravel framework that allows users to automatically execute program code at a specified time. This can be a one-time or recurring interval event. Task Scheduler provides several different ways to manage scheduled tasks, making it easy to use and debug. In Laravel, the task scheduler uses an object called a "scheduler" to manage scheduled tasks.
Task Scheduler in Laravel
Laravel task scheduler uses the following three main components:
Scheduler
Scheduler is the task scheduler Core components. It is an object responsible for scheduling and managing planned tasks. Schedulers provide a simple way to assign tasks to schedules and periods. Once a task is assigned, the scheduler is responsible for ensuring that it is executed at the designated time. The scheduler supports multiple task types, including commands, closures, and method calls.
Schedule
The schedule is an object used to specify the execution time of scheduled tasks. Timetables provide an intuitive way to specify when tasks should be performed. Schedulers can assign tasks to specific schedules to ensure tasks are executed within the scheduled time.
Listener
A listener is an object responsible for recording the execution results of scheduled tasks. If a task is abnormal or does not execute as planned, the listener will record relevant events. This helps users determine when task timing should be modified or other settings adjusted.
How to set up Laravel scheduled tasks
To set up scheduled tasks in Laravel, you need to complete the following steps:
Step 1: Create a controller and command
First, You need to create a controller and a command that will do the actual work. In this example, we will create a "TaskController" controller and assign a "TaskCommand" command to it.
php artisan make:controller TaskController php artisan make:command TaskCommand
Step 2: Edit the command
Open the TaskCommand.php file and update the "fire" method so that the actual task is performed. In this example, we set up the task to write the current timestamp to a file.
public function fire() { file_put_contents(storage_path().'/task.log', time(). "\n", FILE_APPEND); }
Step 3: Create Scheduler Task
Open the app/Console/Kernel.php file and add a new task. In this example, we set the task to execute every minute.
protected function schedule(Schedule $schedule) { $schedule->command('task')->everyMinute(); }
Step 4: Run the task
Finally, you can run the task scheduler using the following command:
php artisan schedule:run
This command will scan the Laravel task scheduler and execute the tasks that need to be executed .
Conclusion
By using the Laravel task scheduler, you can easily execute recurring tasks on a regular basis. Laravel provides a powerful task scheduler that makes setting up and managing scheduled tasks very simple. If you need to automate a task, Laravel Task Scheduler is probably your best choice.
The above is the detailed content of How to set up scheduled tasks in laravel. For more information, please follow other related articles on the PHP Chinese website!
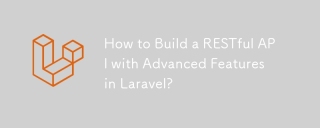
This article guides building robust Laravel RESTful APIs. It covers project setup, resource management, database interactions, serialization, authentication, authorization, testing, and crucial security best practices. Addressing scalability chall
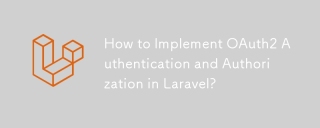
This article details implementing OAuth 2.0 authentication and authorization in Laravel. It covers using packages like league/oauth2-server or provider-specific solutions, emphasizing database setup, client registration, authorization server configu
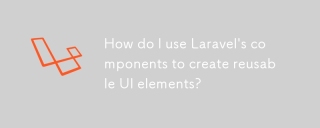
The article discusses creating and customizing reusable UI elements in Laravel using components, offering best practices for organization and suggesting enhancing packages.
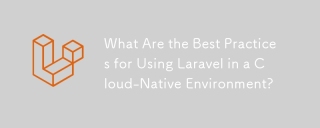
The article discusses best practices for deploying Laravel in cloud-native environments, focusing on scalability, reliability, and security. Key issues include containerization, microservices, stateless design, and optimization strategies.
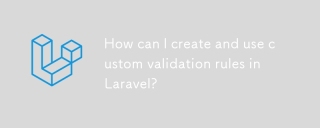
The article discusses creating and using custom validation rules in Laravel, offering steps to define and implement them. It highlights benefits like reusability and specificity, and provides methods to extend Laravel's validation system.
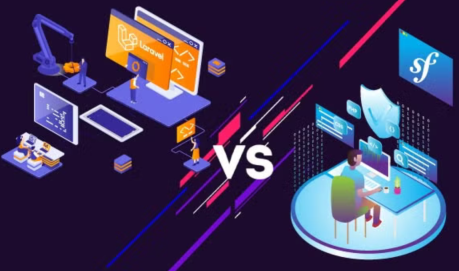
When it comes to choosing a PHP framework, Laravel and Symfony are among the most popular and widely used options. Each framework brings its own philosophy, features, and strengths to the table, making them suited for different projects and use cases. Understanding their differences and similarities is critical to selecting the right framework for your development needs.
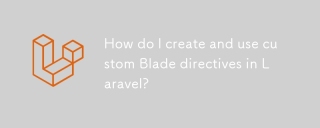
The article discusses creating and using custom Blade directives in Laravel to enhance templating. It covers defining directives, using them in templates, and managing them in large projects, highlighting benefits like improved code reusability and r
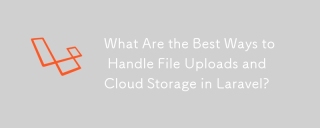
This article explores optimal file upload and cloud storage strategies in Laravel. It examines local storage vs. cloud providers (AWS S3, Google Cloud, Azure, DigitalOcean), emphasizing security (validation, sanitization, HTTPS) and performance opti


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
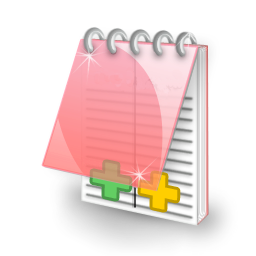
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
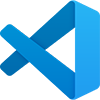
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
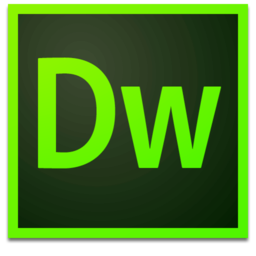
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool