What Are the Best Ways to Handle File Uploads and Cloud Storage in Laravel?
Laravel offers several excellent ways to manage file uploads and integrate with cloud storage services, significantly simplifying the process and improving application scalability. The best approach often depends on your specific needs and project scale.
For smaller applications, using Laravel's built-in file system features can suffice. This involves storing files locally and using the Storage
facade for file manipulation. However, for larger applications or those requiring high availability and scalability, integrating with a cloud storage provider is strongly recommended. This offloads storage management and allows for easier scaling.
The process typically involves:
- Handling the Upload: Using the built-in request validation and file handling capabilities in Laravel, you can easily validate file types, sizes, and names before storing them. This prevents errors and security vulnerabilities.
-
Storing the File: You can choose between storing files locally (using the
Storage
facade) or directly to a cloud storage provider (using a suitable package like Flysystem). Local storage is simple for small projects, but cloud storage is better for scalability and redundancy. - Database Integration: Store relevant file metadata (like filename, path, size, and MIME type) in your database. This allows for easy retrieval and management of files within your application.
-
Using a Package: Packages like
spatie/laravel-medialibrary
provide a more structured and robust approach to managing files and their associated metadata. They offer features like file transformations and multiple file associations with models.
How can I optimize file upload speed and efficiency in a Laravel application?
Optimizing file upload speed and efficiency in a Laravel application involves several strategies targeting both the client-side and server-side processes.
Client-Side Optimizations:
-
Chunking Large Files: For very large files, break them into smaller chunks for upload. This allows for resuming uploads if interrupted and improves user experience. Libraries like
Tus
provide excellent support for this. -
Progress Bars: Display a progress bar to provide feedback to the user during the upload process, enhancing the user experience. JavaScript libraries like
Dropzone.js
can handle this effectively.
Server-Side Optimizations:
- Using a Fast Storage Solution: Choose a fast storage solution (SSD drives for local storage or a high-performance cloud storage provider) to minimize storage latency.
-
Efficient File Handling: Use optimized file handling functions and avoid unnecessary operations. Laravel's
Storage
facade already provides a reasonably efficient interface. - Asynchronous Processing: Process uploaded files asynchronously using queues (like Laravel's queue system). This prevents blocking the main request thread, maintaining responsiveness.
- Caching: If you process uploaded files (like image resizing or conversion), caching processed files can significantly reduce processing time for subsequent requests.
- Load Balancing: For high-traffic applications, consider using load balancing to distribute the upload load across multiple servers.
- Optimize Database Interactions: Minimize database interactions during the upload process. Batch inserts are more efficient than individual inserts.
What are the security considerations when implementing file uploads and cloud storage in Laravel?
Security is paramount when handling file uploads and cloud storage. Several crucial considerations must be addressed:
- Input Validation: Rigorously validate all uploaded files to prevent malicious files from being uploaded. Check file types, sizes, and contents to prevent script injections and other attacks. Laravel's validation features are essential here.
-
File Type Validation: Restrict uploaded file types to only those your application needs. Avoid allowing potentially dangerous file types (like
.exe
,.bat
,.sh
). - File Size Limits: Implement file size limits to prevent denial-of-service (DoS) attacks where large files consume server resources.
- Secure Storage: Use secure storage mechanisms. For cloud storage, ensure proper access control and encryption. For local storage, use appropriate file permissions to restrict access.
- Sanitize Filenames: Sanitize filenames to remove potentially harmful characters before storing them. This prevents directory traversal attacks.
- HTTPS: Always use HTTPS to encrypt communication between the client and server, protecting sensitive data during upload.
- Regular Security Audits: Conduct regular security audits and penetration testing to identify and address potential vulnerabilities.
- Cloud Provider Security: If using a cloud storage provider, carefully review their security practices and ensure they meet your security requirements.
What cloud storage providers integrate best with Laravel for file uploads?
Several cloud storage providers offer excellent integration with Laravel, each with its own strengths and weaknesses:
- Amazon S3: A highly popular and robust solution, offering excellent scalability, reliability, and features. Laravel's built-in support for Flysystem makes integration straightforward.
- Google Cloud Storage: Another powerful and scalable option with good integration through Flysystem.
- Azure Blob Storage: Microsoft's cloud storage solution also integrates well with Laravel via Flysystem.
- DigitalOcean Spaces: A more affordable option that is simple to use and integrates well with Laravel.
The "best" provider depends on your specific needs and preferences. Consider factors such as cost, scalability, geographic location, and existing infrastructure when making your choice. All mentioned providers generally offer seamless integration with Laravel via Flysystem, simplifying the configuration and usage. Remember to choose a provider that aligns with your application's security and compliance requirements.
The above is the detailed content of What Are the Best Ways to Handle File Uploads and Cloud Storage in Laravel?. For more information, please follow other related articles on the PHP Chinese website!

Laravel stands out by simplifying the web development process and delivering powerful features. Its advantages include: 1) concise syntax and powerful ORM system, 2) efficient routing and authentication system, 3) rich third-party library support, allowing developers to focus on writing elegant code and improve development efficiency.

Laravelispredominantlyabackendframework,designedforserver-sidelogic,databasemanagement,andAPIdevelopment,thoughitalsosupportsfrontenddevelopmentwithBladetemplates.

Laravel and Python have their own advantages and disadvantages in terms of performance and scalability. Laravel improves performance through asynchronous processing and queueing systems, but due to PHP limitations, there may be bottlenecks when high concurrency is present; Python performs well with the asynchronous framework and a powerful library ecosystem, but is affected by GIL in a multi-threaded environment.

Laravel is suitable for projects that teams are familiar with PHP and require rich features, while Python frameworks depend on project requirements. 1.Laravel provides elegant syntax and rich features, suitable for projects that require rapid development and flexibility. 2. Django is suitable for complex applications because of its "battery inclusion" concept. 3.Flask is suitable for fast prototypes and small projects, providing great flexibility.

Laravel can be used for front-end development. 1) Use the Blade template engine to generate HTML. 2) Integrate Vite to manage front-end resources. 3) Build SPA, PWA or static website. 4) Combine routing, middleware and EloquentORM to create a complete web application.

PHP and Laravel can be used to build efficient server-side applications. 1.PHP is an open source scripting language suitable for web development. 2.Laravel provides routing, controller, EloquentORM, Blade template engine and other functions to simplify development. 3. Improve application performance and security through caching, code optimization and security measures. 4. Test and deployment strategies to ensure stable operation of applications.

Laravel and Python have their own advantages and disadvantages in terms of learning curve and ease of use. Laravel is suitable for rapid development of web applications. The learning curve is relatively flat, but it takes time to master advanced functions. Python's grammar is concise and the learning curve is flat, but dynamic type systems need to be cautious.

Laravel's advantages in back-end development include: 1) elegant syntax and EloquentORM simplify the development process; 2) rich ecosystem and active community support; 3) improved development efficiency and code quality. Laravel's design allows developers to develop more efficiently and improve code quality through its powerful features and tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
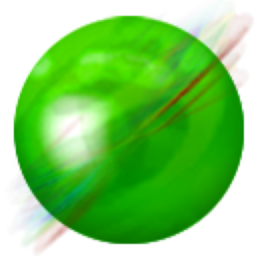
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment