In PHP, sometimes we need to deduplicate an array, that is, remove repeated elements. This process may seem simple, but different situations may require different approaches. Next, we will discuss some common array deduplication methods and their application scenarios.
1. Use the PHP built-in function array_unique()
PHP provides a built-in function array_unique(), which can directly perform deduplication operations on an array. The syntax for using this function is as follows:
array array_unique ( array $array [, int $sort_flags = SORT_STRING ] )
Among them, $array is the array to be duplicated, $sort_flags is an optional parameter, used to specify the comparison method of elements, and can take the following values:
- SORT_REGULAR - Compare elements in the usual way (without changing the data type of the elements)
- SORT_NUMERIC - Compare elements based on numeric size
- SORT_STRING - Compare elements based on strings
- SORT_LOCALE_STRING - Compares elements according to the current locale
- SORT_NATURAL - Compares elements according to their natural ordering
The sample code is as follows:
$arr = array(1, 2, 2, 3, 4, 4, 5); $new_arr = array_unique($arr); print_r($new_arr); // 输出:Array ( [0] => 1 [1] => 2 [3] => 3 [4] => 4 [6] => 5 )
Advantages of using array_unique() The reason is that it is simple and easy to use, and the array deduplication operation can be completed in one line of code. The disadvantage is that it can only handle one-dimensional arrays. If you want to handle multi-dimensional arrays, additional processing is required.
2. Use loop conditional judgment
If you want to process a multi-dimensional array, you can use loop conditional judgment to remove duplicates. The idea of this method is to traverse the array, compare each element in turn with the previous element in the array (except those that have been deduplicated), and skip if duplicates are found, otherwise add the element to the deduplicated array. The sample code is as follows:
$arr = array( array(1, 2, 2), array(3, 4, 4), array(5, 6, 7), array(1, 2, 2) ); $new_arr = array(); foreach ($arr as $value) { if (!in_array($value, $new_arr)) { $new_arr[] = $value; } } print_r($new_arr); // 输出:Array ( [0] => Array ( [0] => 1 [1] => 2 [2] => 2 ) [1] => Array ( [0] => 3 [1] => 4 [2] => 4 ) [2] => Array ( [0] => 5 [1] => 6 [2] => 7 ) )
Using loops The advantage of conditional judgment is that it is suitable for different forms of arrays, including multi-dimensional arrays, and can maintain good performance even when the amount of data is large. The disadvantage is that it requires writing more code, and it is easy to make mistakes if there are slight flaws.
3. Use PHP built-in function array_reduce()
In addition to array_unique(), PHP also provides a built-in function array_reduce(), which can be used to perform reduction operations on arrays. For array deduplication, we can convert the array into an associative array with array elements as keys and empty values, and then merge it with the original array. If the same elements are encountered, skip them, otherwise add them to the merge in the subsequent array. The sample code is as follows:
$arr = array(1, 2, 2, 3, 4, 4, 5); $new_arr = array_reduce($arr, function($carry, $item) { if(!array_key_exists($item, $carry)) { $carry[$item] = null; } return $carry; }, array()); print_r(array_keys($new_arr)); // 输出:Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 )
The advantage of using array_reduce() is that it can flexibly handle different forms of arrays and the code is concise. The disadvantage is that the performance may be reduced for arrays with large amounts of data.
To sum up, there are many ways to deduplicate arrays in PHP, and you can choose the appropriate method according to the specific situation. For one-dimensional arrays, it is recommended to use array_unique() first; for multi-dimensional arrays, it is recommended to use loop conditional judgment or array_reduce(). Program performance and data quality can be improved by deduplicating data.
The above is the detailed content of How to remove identical arrays in php. For more information, please follow other related articles on the PHP Chinese website!
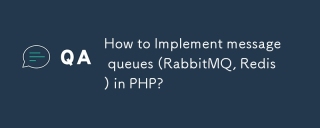
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
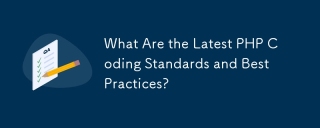
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
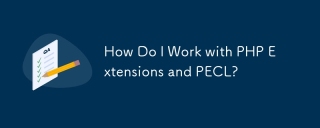
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
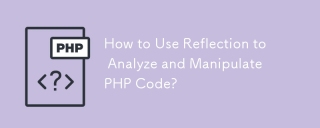
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
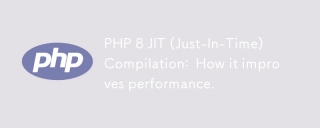
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
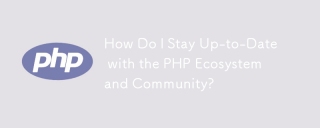
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
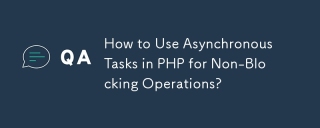
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
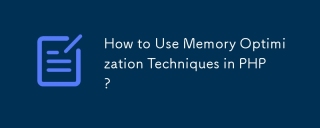
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
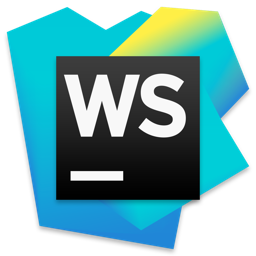
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
