PHP is a powerful server-side programming language that has various data types and functions that can be used to develop web applications. In PHP, array is a very important data type that can be used to store large amounts of data, such as numbers, strings, objects, etc. In PHP, we can use various methods to compare the size of two numbers, including using conditional statements, mathematical functions and operators, etc. This article will introduce how to use PHP arrays to determine the size between two numbers.
Conditional statements
In PHP, we can use conditional statements (if/else statements) to compare the size of two numbers. Here is a simple example of how to compare the size of two numbers using conditional statements:
$num1 = 10; $num2 = 20; if ($num1 $num2) { echo "num1比num2大"; } else { echo "num1和num2相等"; }
In the above example, we have declared two variables $num1 and $num2 and used if/else statements Compare the sizes between them. If $num1 is less than $num2, output "num2 is greater than num1", otherwise if $num1 is greater than $num2, output "num1 is greater than num2", otherwise output "num1 and num2 are equal".
Mathematical functions
In PHP, there are also some mathematical functions that can be used to compare the size of two numbers. For example, we can use the max() function to find the maximum value in a set of numbers and the min() function to find the minimum value. Here is an example:
$num1 = 10; $num2 = 20; $max = max($num1, $num2); $min = min($num1, $num2); echo "最大值是:" . $max . "<br>"; echo "最小值是:" . $min . "<br>";
In the above example, we declared two variables $num1 and $num2 and compared the size between them using the max() function and min() function. We pass $num1 and $num2 as arguments to these functions and assign their return values to the variables $max and $min. Then, we use the echo statement to print the values of $max and $min.
Operators
In addition to using conditional statements and mathematical functions, we can also use operators in PHP to compare the size of two numbers. Here are some operators for comparing two numbers:
- $a > $b: Returns true if $a is greater than $b, false otherwise.
- $a
- $a >= $b: If $a is greater than or equal to $b, return true, otherwise return false.
- $a
- $a == $b: If $a is equal to $b, return true, otherwise return false.
- $a != $b: If $a is not equal to $b, return true, otherwise return false.
We can use these operators to compare the size of two numbers. Here is a simple example:
$num1 = 10; $num2 = 20; if ($num1 > $num2) { echo "num1比num2大"; } else if ($num1 <p>In the above example, we have used the greater than operator and the less than operator to compare the size between $num1 and $num2. If $num1 is greater than $num2, output "num1 is greater than num2", otherwise if $num1 is less than $num2, output "num2 is greater than num1", otherwise output "num1 and num2 are equal". </p><p>Summary</p><p>In PHP, we can use various methods to compare the size of two numbers, including using conditional statements, mathematical functions and operators, etc. Using conditional statements makes your code easier to understand and maintain, mathematical functions make it easy to find the maximum and minimum values in a set of numbers, and operators make it faster to compare the size of two numbers. According to the actual situation, we can choose an appropriate method to compare the size of two numbers to achieve better results in actual development. </p>
The above is the detailed content of How to determine the size of two numbers in a php array. For more information, please follow other related articles on the PHP Chinese website!
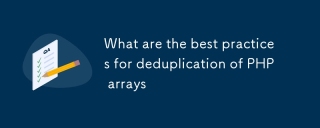
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
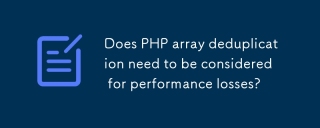
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
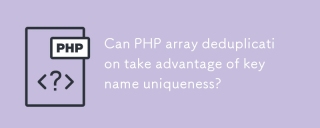
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
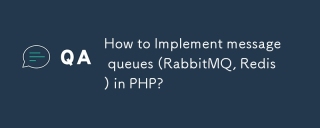
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
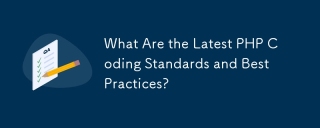
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
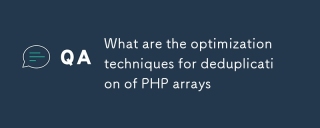
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
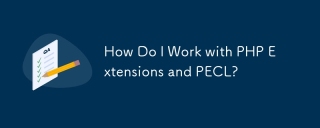
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
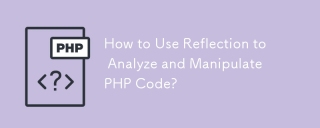
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
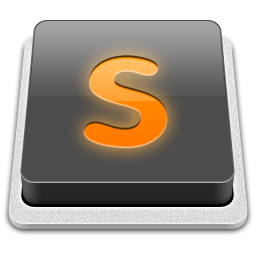
SublimeText3 Mac version
God-level code editing software (SublimeText3)
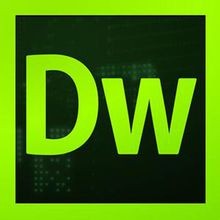
Dreamweaver CS6
Visual web development tools
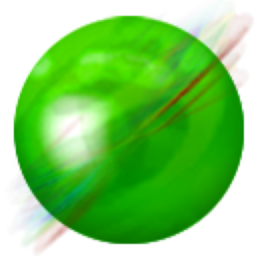
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
