Associative array is a very important data type in PHP and one of the data types that is used relatively frequently. Associative arrays can store a series of key-value pairs in an array, where the keys and values can be of any data type, including numbers, strings, arrays, etc. In PHP, there are many ways to store associative arrays. This article will introduce one of the common methods: storing them one by one.
1. Create an associative array
In PHP, creating an associative array is very simple, just use the array() or [] operator. Among them, array() is a function, and the [] operator is an array literal. Key-value pairs in an associative array can be separated by a colon (:) or an equal sign (=), as shown below:
// 使用 array() 函数创建How to store associative array in php $arr1 = array("name" => "张三", "age" => 18, "gender" => "男"); // 使用数组字面量 [] 创建How to store associative array in php $arr2 = ["name": "李四", "age": 20, "gender": "女"];
In the above code, $arr1 and $arr2 are both associative arrays, and they are represented by It consists of three key-value pairs. Each key-value pair consists of a key and a value. The keys of $arr1 are "name", "age" and "gender", and the corresponding values are "Zhang San", 18 and "male", while the keys of $arr2 are "name", "age" and "gender" respectively, and the corresponding values are "John Doe", 20 and "female" respectively.
2. Store associative arrays one by one
In PHP, you can add key-value pairs to associative arrays by storing them one by one. This method is suitable for situations where associative arrays need to be dynamically created or there are many key-value pairs. Let’s take a look at the specific process.
- Create an empty associative array
First create an empty associative array $employees, and then add employee information one by one, where each employee information includes name, gender ( Four attributes: gender), age (age) and position (position). The code is as follows:
$employees = [];
- Add employee information
Next we add employee information to the associative array $employees one by one. As shown below:
$employees['person1']['name'] = '张三'; $employees['person1']['gender'] = '男'; $employees['person1']['age'] = 28; $employees['person1']['position'] = '项目经理'; $employees['person2']['name'] = '李四'; $employees['person2']['gender'] = '女'; $employees['person2']['age'] = 25; $employees['person2']['position'] = 'UI设计师'; $employees['person3']['name'] = '王五'; $employees['person3']['gender'] = '男'; $employees['person3']['age'] = 32; $employees['person3']['position'] = '技术总监';
In the above code, we first assign a number to each employee, such as person1, person2 and person3, as a key. Then, for each employee, we use the form $employees['person1'] to specify the key to which the employee's key-value pair belongs, and add the name, gender, age and position attributes to the employee by assigning values one by one, such as $employees'person1' = 'Zhang San'.
- Output employee information
Finally, we can output the employee information stored in the $employees associative array through a loop. The code is as follows:
foreach ($employees as $personKey => $personValue) { echo "<strong>员工编号:$personKey</strong><br>"; foreach ($personValue as $detailKey => $detailValue) { echo "$detailKey:$detailValue<br>"; } echo '<br>'; }
The output result is as shown in the figure below:
As can be seen from the above code and results, we use the method of storing one by one to associate Adding key-value pairs to the array finally outputs the data stored in the associative array in a more intuitive way.
Summary
Associative array is a very powerful data type in PHP. It can store multiple key-value pairs in an array. Each key-value pair can be composed of a key and a Composed of values, which can be of any data type. In PHP, you can create and operate associative arrays through a variety of methods. The one-by-one storage method is suitable for situations where you need to dynamically create an associative array, or when there are many key-value pairs. No matter which method is used, mastering the use of associative arrays is an essential basic skill in PHP development.
The above is the detailed content of How to store associative array in php. For more information, please follow other related articles on the PHP Chinese website!
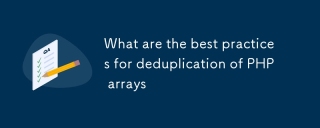
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
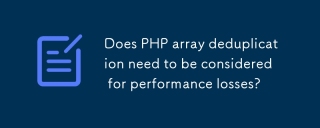
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
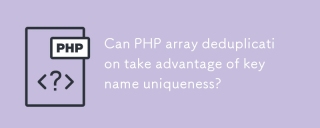
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
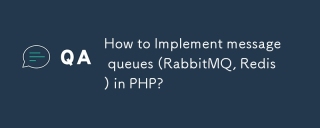
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
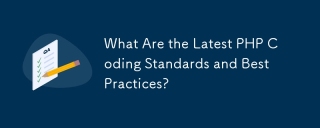
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
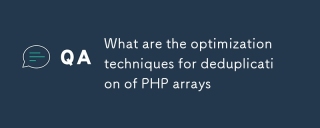
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
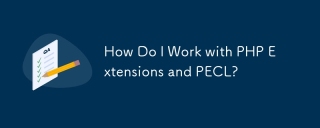
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
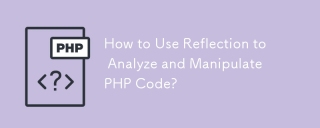
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
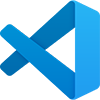
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
