Golang is an efficient programming language favored by many programmers for its simple syntax and good performance. The canvas is a very common requirement, especially in some graphics processing or game development. Today, we will explore how to implement a basic canvas using Golang.
First, we need to introduce some Golang libraries to implement our canvas. We will use the image
and image/color
libraries, image
provides the basic image processing functions we need, and image/color
Provides our treatment of color.
import ( "image" "image/color" )
Next, we need to define the basic properties of the canvas. Including width, height, background color, etc. Here we define a structure named canvas
and an initialization function to initialize the structure.
type canvas struct { width, height int bg color.Color img *image.RGBA } func newCanvas(width, height int, bg color.Color) *canvas { return &canvas{ width: width, height: height, bg: bg, img: image.NewRGBA(image.Rect(0, 0, width, height)), } }
canvas
The structure contains the width, height, background color and actual image of the canvas. In the newCanvas
function, we pass in the width, height and background color of the canvas, and initialize the img
property.
Next, we need to implement some drawing operations, such as drawing straight lines, drawing rectangles, etc. Here we can use the functions in the image/draw
library to achieve this. We define a method named line
to draw a straight line on the canvas.
func (c *canvas) line(x1, y1, x2, y2 int, color color.Color) { lineColor := &color drawLine(c.img, x1, y1, x2, y2, lineColor) } func drawLine(img *image.RGBA, x1, y1, x2, y2 int, color *color.Color) { dx := abs(x2 - x1) sx := 1 if x1 > x2 { sx = -1 } dy := abs(y2 - y1) sy := 1 if y1 > y2 { sy = -1 } err := dx - dy for { img.Set(x1, y1, *color) if x1 == x2 && y1 == y2 { break } e2 := 2 * err if e2 > -dy { err -= dy x1 += sx } if e2 <p>In the <code>line</code> method, we pass in the starting point coordinates, the end point coordinates, and the line color. Then, we call the <code>drawLine</code> function to draw a straight line. The <code>drawLine</code> function uses the Bresenham algorithm, which is a classic straight line drawing algorithm. </p><p>Similarly, we can also implement operations such as drawing rectangles, circles, etc. Here we only show the implementation of drawing a rectangle, other operations are similar. </p><pre class="brush:php;toolbar:false">func (c *canvas) rectangle(x1, y1, x2, y2 int, color color.Color) { rectColor := &color drawRectangle(c.img, x1, y1, x2, y2, rectColor) } func drawRectangle(img *image.RGBA, x1, y1, x2, y2 int, color *color.Color) { drawLine(img, x1, y1, x2, y1, color) drawLine(img, x2, y1, x2, y2, color) drawLine(img, x2, y2, x1, y2, color) drawLine(img, x1, y2, x1, y1, color) }
Finally, we need to implement an output function to output the canvas to a file or screen. Here we define a method named output
, which accepts a file name and outputs the canvas to the file.
func (c *canvas) output(filename string) error { file, err := os.Create(filename) if err != nil { return err } defer file.Close() err = png.Encode(file, c.img) if err != nil { return err } return nil }
In the output
method, we create the file through the os.Create
function, and then use the png.Encode
function to encode the image into PNG format and written to a file.
Now, we have implemented a basic canvas. We can create a canvas object and call its methods to draw lines, rectangles, circles, etc., and then call the output
method to output the image to a file. Here is a usage example:
func main() { c := newCanvas(200, 200, color.White) // 画一条红线 c.line(0, 0, 200, 200, color.RGBA{255, 0, 0, 255}) // 画一个蓝色矩形 c.rectangle(50, 50, 150, 150, color.RGBA{0, 0, 255, 255}) // 输出到文件 c.output("canvas.png") }
In this example, we create a 200x200 white canvas, then draw a red line and a blue rectangle on it, and output the image to "canvas.png" in the file. You can implement your own canvas by calling methods similarly.
To summarize, by using Golang’s image
and image/color
libraries, we can easily implement a basic canvas and perform various tasks on it Drawing operations. Of course, this is just a simple example and there is a lot of room for optimization and expansion. I hope this article can help you master basic Golang canvas programming skills.
The above is the detailed content of Explore how to use Golang to implement a basic canvas function. For more information, please follow other related articles on the PHP Chinese website!
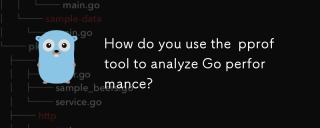
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
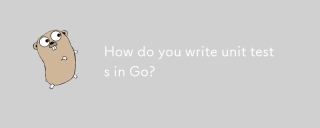
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
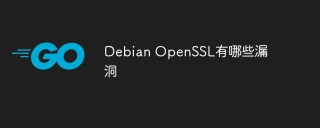
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
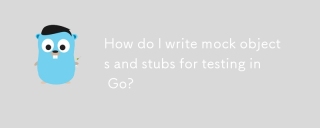
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
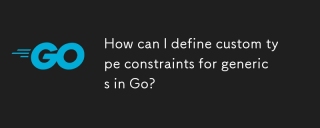
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
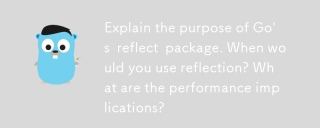
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
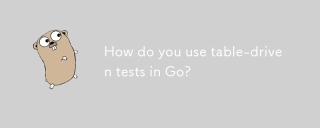
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
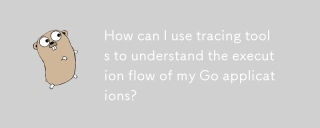
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
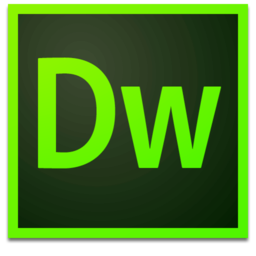
Dreamweaver Mac version
Visual web development tools