Go language is an open source programming language that can be used to quickly develop simple and reliable programs. In the development process of Go language, hexadecimal conversion is often required. This article will explain how to perform base conversion in Go language.
Decimal to Binary
In Go language, you can use the strconv package to convert decimal numbers to binary numbers.
package main import ( "fmt" "strconv" ) func main() { num := 10 binary := strconv.FormatInt(int64(num), 2) fmt.Println(num, "的二进制表示为", binary) }
The above code will output:
10 的二进制表示为 1010
The strconv.FormatInt() function converts a decimal number into a binary string. The first parameter of this function is the value to be converted, and the second parameter is the target base, which is 2 in this example.
Binary to Decimal
If you need to convert a binary value to a decimal value, you can also use the strconv package.
package main import ( "fmt" "strconv" ) func main() { binary := "1010" num, _ := strconv.ParseInt(binary, 2, 64) fmt.Println(binary, "的十进制表示为", num) }
The above code will output:
1010 的十进制表示为 10
strconv.ParseInt() function parses a string into an integer. The first parameter of this function is the string to be parsed, the second parameter is the target base, in this case it is 2, and the third parameter is the number of digits in the result type, in this case it is a 64-bit integer.
Decimal to hexadecimal
In the Go language, the strconv package also provides a function for converting decimal numbers to hexadecimal numbers:
package main import ( "fmt" "strconv" ) func main() { num := 33752069 hex := strconv.FormatInt(int64(num), 16) fmt.Println(num, "的十六进制表示为", hex) }
The above code Will output:
33752069 的十六进制表示为 2030405
strconv.FormatInt() function converts a decimal number into a hexadecimal string. The first parameter of this function is the value to be converted, and the second parameter is the target base, which is 16 in this example.
Hexadecimal to Decimal
If you need to convert a hexadecimal value to a decimal value, you can also use the strconv package.
package main import ( "fmt" "strconv" ) func main() { hex := "2030405" num, _ := strconv.ParseInt(hex, 16, 64) fmt.Println(hex, "的十进制表示为", num) }
The above code will output:
2030405 的十进制表示为 33752069
strconv.ParseInt() function parses a string into an integer. The first parameter of this function is the string to be parsed, the second parameter is the target base, in this case it is 16, and the third parameter is the number of digits in the result type, in this case it is a 64-bit integer.
Summary
This article introduces how to perform base conversion in Go language. Through the strconv package, we can easily convert decimal numbers to other base numbers, or other base numbers to decimal numbers. This is one of the very common skills in Go language programming.
The above is the detailed content of How to perform hexadecimal conversion in Go language. For more information, please follow other related articles on the PHP Chinese website!
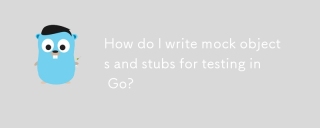
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
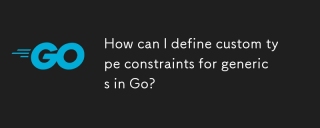
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
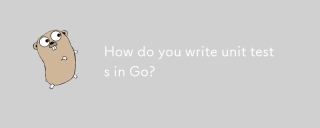
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
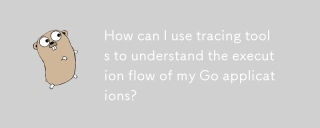
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
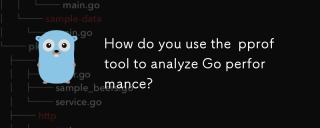
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
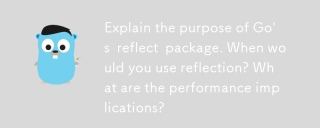
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
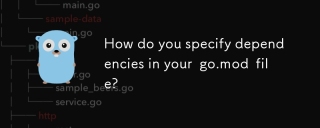
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
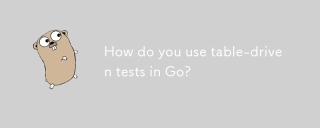
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
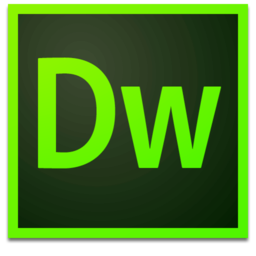
Dreamweaver Mac version
Visual web development tools
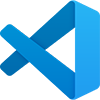
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
