In network programming, requesting cookies is a very important function, especially when you need to request a web page from a web server, this function becomes very useful. Now when writing programs in Golang, requesting cookies is also one of the essential operations. In this article, we will introduce how to use Golang to request cookies.
- What is a cookie
First of all, we need to know what a cookie is. A cookie is a small data file stored on the user's computer. It can pass data between web server and client. When we request a website, the website sends a cookie containing some information to our browser, which the browser stores locally and sends back to the server on subsequent requests.
- Request cookie in Golang
In Golang, we can use the Client
structure in the net/http
package The body sends an HTTP request. An HTTP request can be created by using the http.NewRequest()
function. By setting the "Cookie" field in the request header, we can send cookie information.
The following is a sample code for requesting cookies using Golang:
package main import ( "fmt" "net/http" ) func main() { // 创建一个HTTP客户端 client := &http.Client{} // 创建一个GET请求 req, err := http.NewRequest("GET", "http://example.com", nil) if err != nil { fmt.Println(err) return } // 设置请求头中的Cookie信息 cookie := &http.Cookie{Name: "name", Value: "value"} req.AddCookie(cookie) // 发送请求 resp, err := client.Do(req) if err != nil { fmt.Println(err) return } // 打印响应 fmt.Println(resp) }
In the above sample code, we created an HTTP client and used http.NewRequest()
The function creates a GET request. Then, we use the req.AddCookie()
function to set the Cookie information in the request header. Finally, we use the client.Do()
function to send the request and print the returned response result.
- Request multiple cookies
Some websites may store multiple pieces of information in one cookie. In this case, we need to set multiple cookie information in the request header.
The following is a Golang sample code that sets multiple cookie information in the request header:
package main import ( "fmt" "net/http" ) func main() { // 创建一个HTTP客户端 client := &http.Client{} // 创建一个GET请求 req, err := http.NewRequest("GET", "http://example.com", nil) if err != nil { fmt.Println(err) return } // 设置请求头中的多个Cookie信息 cookies := []*http.Cookie{ &http.Cookie{Name: "name1", Value: "value1"}, &http.Cookie{Name: "name2", Value: "value2"}, } for _, c := range cookies { req.AddCookie(c) } // 发送请求 resp, err := client.Do(req) if err != nil { fmt.Println(err) return } // 打印响应 fmt.Println(resp) }
In the above sample code, we use req.AddCookie()
The function sets two cookie information in the request header, then sends the request and prints the returned response result.
- Conclusion
In this article, we introduced how to use Golang to request cookies. By setting the "Cookie" field in the request header, we can send cookie information to the web server to achieve more efficient communication. By studying the content of this article, you can master the skills of using Golang to request cookies and apply them to your actual projects.
The above is the detailed content of How to use Golang to request cookies. For more information, please follow other related articles on the PHP Chinese website!
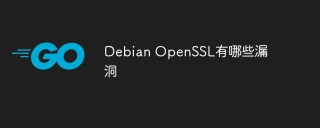
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
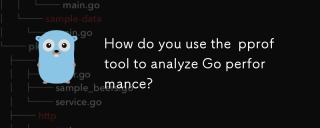
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
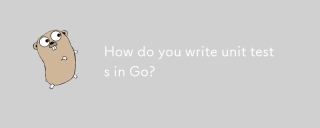
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
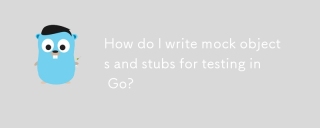
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
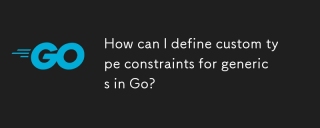
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
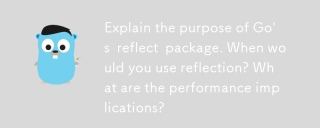
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
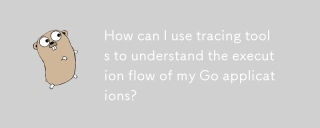
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
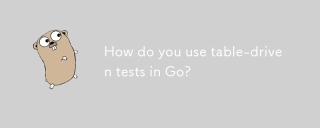
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Zend Studio 13.0.1
Powerful PHP integrated development environment
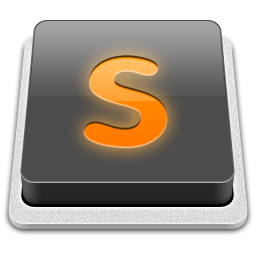
SublimeText3 Mac version
God-level code editing software (SublimeText3)
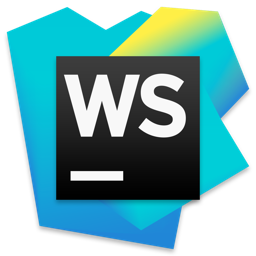
WebStorm Mac version
Useful JavaScript development tools