With the rapid development of the mobile Internet industry, the chat function has become one of the regular functions of many APPs, and conversation is the basis for chat. When I was learning uniapp technology recently, I found that uniapp also provides a rich API that can easily implement session functions. Here, combined with the author's learning experience, I will share how uniapp implements conversation.
1. Basic concepts
Before we start to implement the session function, let’s understand the basic concepts of session. A session is a series of interactive processes between a session object from the beginning to the end. In chat applications, a conversation object usually includes chat records between two or more people, arranged in chronological order.
2. Project Construction
This article will use the uniapp framework and the uniCloud environment as an example to introduce the session implementation process. First, we need to build a uniapp project. The specific steps are as follows:
- Create a new uniapp project in HBuilderX and select uniCloud as the server environment.
- In the manifest.json file, configure the network request permissions and navigation bar style.
- In the pages folder, create a new chat folder to store chat-related pages and components.
3. Function implementation
- Login authentication
Before implementing the session function, we need to perform the login authentication operation first. uniCloud provides two methods of login with account and password and WeChat login. We can make corresponding selections on the login page and call uniCloud's API to complete the login operation. After successful login, the member information will be stored locally or in uniCloud.
- Get the chat list
Getting the chat list is an important step to implement the conversation function. In this article, we will use the cloud functions provided by uniCloud to implement it. First, create a new cloud function in the uniCloud platform and name it "getChatList". In the cloud function, we can query the user's chat list information and return data in JSON format to the front end.
Cloud function code example:
'use strict'; const db = uniCloud.database() exports.main = async (event, context) => { const collection = db.collection('chatList') const res = await collection.where({ openid: event.openid }).get() return res.data };
In the front-end page, we can call uniCloud's cloud function API to obtain chat list data. In the chat folder, create a new chatlist.vue file to display the user's chat list. Use the uni-list component to render the chat list.
- Implementing the chat page
When clicking on a chat record in the chat list, we need to enter the chat page and display the chat content. In the chat folder, we create a new chat.vue file to implement the chat interaction function. The specific implementation steps are as follows:
(1) By passing in user information and chat object information, call the cloud function to obtain the chat record and display it.
(2) Use the uni-input component to implement the message input box.
(3) Use uni-list component and uni-message component to realize message list display.
(4) Implement the message sending function by calling cloud functions, and display the message in the chat page in real time.
Chat page code example:
<template> <view> <view> <view> <view> <image></image> </view> <view> <view> <template>{{message.content}}</template> </view> </view> <view> <image></image> </view> </view> </view> <view> <uni-input></uni-input> </view> </view> </template> <script> import { uniCloud } from 'wx-resource' import { mapState } from 'vuex' import socket from '@/utils/socket.js' export default { data() { return { messages: [], inputContent: '' } }, computed: { ...mapState(['userInfo','friendInfo']) }, onLoad() { this.getChatList() }, methods: { async getChatList() { const res = await uniCloud.callFunction({ name: 'getChatList', data: { openid: this.userInfo.openid, friendOpenid: this.friendInfo.openid } }) this.messages = res.result }, async sendMessage() { if (this.inputContent === '') { return } socket.emit('chat message', { openid: this.userInfo.openid, friendOpenid: this.friendInfo.openid, content: this.inputContent.trim(), type: 'text' }) this.inputContent = '' } }, created() { socket.on('chat message', message => { this.messages.push(message) }) } } </script> <style> .chat-page { display: flex; flex-direction: column; height: 100%; } .chat-messages { flex: 1; overflow-y: scroll; } .chat-message { display: flex; margin: 10px; max-width: 60%; } .chat-message .avatar { margin-right: 10px; } .chat-message .message-info { display: flex; flex-direction: column; justify-content: space-around; flex-grow: 1; max-width: 80%; } .chat-message.right .message-info { align-items: flex-end; } .chat-message .avatar-img { display: block; border-radius: 50%; width: 40px; height: 40px; } .message-content { word-wrap: break-word; white-space: pre-wrap; background-color: #eee; padding: 7px; border-radius: 10px; } .chat-input { padding: 10px; background: #fff; } </style>
IV. Summary
Through the introduction of this article, we understand how uniapp implements the session function and shows the specific code to implement the chat page . During the actual development process, we can also expand and optimize these functions according to our own needs. I hope it can be helpful to everyone in uniapp development.
The above is the detailed content of How to implement session in uniapp. For more information, please follow other related articles on the PHP Chinese website!
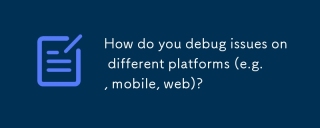
The article discusses debugging strategies for mobile and web platforms, highlighting tools like Android Studio, Xcode, and Chrome DevTools, and techniques for consistent results across OS and performance optimization.

The article discusses debugging tools and best practices for UniApp development, focusing on tools like HBuilderX, WeChat Developer Tools, and Chrome DevTools.

The article discusses end-to-end testing for UniApp applications across multiple platforms. It covers defining test scenarios, choosing tools like Appium and Cypress, setting up environments, writing and running tests, analyzing results, and integrat
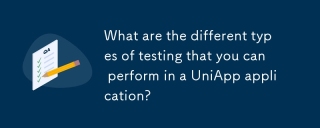
The article discusses various testing types for UniApp applications, including unit, integration, functional, UI/UX, performance, cross-platform, and security testing. It also covers ensuring cross-platform compatibility and recommends tools like Jes
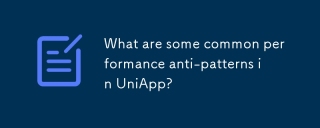
The article discusses common performance anti-patterns in UniApp development, such as excessive global data use and inefficient data binding, and offers strategies to identify and mitigate these issues for better app performance.
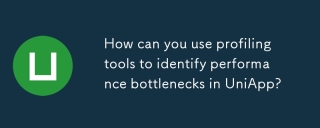
The article discusses using profiling tools to identify and resolve performance bottlenecks in UniApp, focusing on setup, data analysis, and optimization.
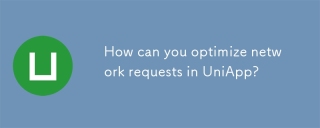
The article discusses strategies for optimizing network requests in UniApp, focusing on reducing latency, implementing caching, and using monitoring tools to enhance application performance.
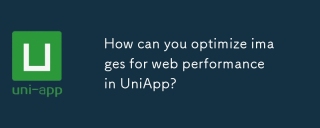
The article discusses optimizing images in UniApp for better web performance through compression, responsive design, lazy loading, caching, and using WebP format.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.