Use the function in the fmt package to print
The Go language is a statically typed, compiled language developed by Google. Its syntax is concise and clear, and its concurrency capabilities are powerful, so it has attracted much attention in recent years. . There are many built-in functions in the Go language, including printing functions. In this article, we will learn how to print using functions of fmt package in Go language.
1. fmt package
In the Go language, the fmt package is a very powerful package that implements the function of formatted input and output. The fmt package can easily perform printing operations. It can print values of various data types and also set formats. The fmt package also provides some other functions such as Scan, Scanln and Scanf for reading data from standard input. Let's take a look at how to use the functions of the fmt package for printing.
2. The Print function in the fmt package
The Print function in the fmt package can print data to the standard output. The code below shows how to use the Print function to print a string to the console.
package main import "fmt" func main() { fmt.Print("Hello, world!") }
In the above code, the Print function of the fmt package is called and the string "Hello, world!" is printed to the console. The printing here will not automatically wrap at the end. If you want to add a newline character at the end, you can use the println function.
package main import "fmt" func main() { fmt.Println("Hello, world!") }
3. The Printf function in the fmt package
The Printf function of the fmt package can print a formatted string to the standard output. The first parameter of the Printf function is the format string, and the subsequent parameters are the content to be filled. The code below demonstrates how to print a formatted string using the Printf function.
package main import "fmt" func main() { name := "Tom" age := 32 fmt.Printf("My name is %s,I'm %d years old.", name, age) }
In the above code, the formatted string in sprintf is "My name is %s, I'm %d years old.", where the % character is followed by a letter, indicating this string The type of data that needs to be populated. For example, %s represents the string type, and %d represents the integer type. In this example, the first %s will be replaced by the value of the string variable name, and the second %d will be replaced by the value of the integer variable age.
4. Other functions in the fmt package
There are other functions in the fmt package, such as Sprintf function, Fprint function and Fprintf function. They are similar to Print and Printf functions, but use The method or output target is different. Below we briefly introduce these functions.
- Sprintf function
. The Sprintf function is similar to the Printf function, but it does not output a formatted string to the console, but returns a string. The code below demonstrates how to use the Sprintf function.
package main import "fmt" func main() { name := "Tom" age := 32 // 将格式化字符串保存到变量str中 str := fmt.Sprintf("My name is %s,I'm %d years old.", name, age) // 输出变量str中的字符串 fmt.Print(str) }
- Fprint function
The first parameter of the Fprint function is an io.Writer (interface), indicating the output destination. It can output formatted strings to files, network connections, standard output, etc., just pass in the appropriate io.Writer. For example, the following code demonstrates how to output a formatted string to a file.
package main import ( "fmt" "os" ) func main() { f, err := os.Create("test.txt") if err != nil { fmt.Println(err) return } defer f.Close() // 将格式化字符串输出到文件中 fmt.Fprint(f, "Hello, world!") }
In the above code, the os.Create function creates a file named test.txt, and the formatted string "Hello, world!" is written to this file.
- Fprintf function
The Fprintf function is similar to the Printf function, but it does not output the formatted string to the console, but outputs it to a file or network Connections, standard output, etc. locations. Similar to the Fprint function, you just need to pass in the appropriate io.Writer. The code below demonstrates how to output a formatted string to a file.
package main import ( "fmt" "os" ) func main() { f, err := os.Create("test.txt") if err != nil { fmt.Println(err) return } defer f.Close() name := "Tom" age := 32 // 将格式化字符串输出到文件中 fmt.Fprintf(f, "My name is %s,I'm %d years old.", name, age) }
In the above code, the fmt.Fprintf function outputs the formatted string to the file, and the variables in the formatted string "My name is %s, I'm %d years old." are replaced with corresponding values.
Summary:
In the Go language, the functions of the fmt package can easily perform printing operations. They can print values of various data types and can also perform formatting operations. By using some other functions, such as Sprintf function, Fprint function and Fprintf function, the output content can be made more flexible and diverse. Proficient in the functions in the fmt package can enable us to develop Go language programs more effectively.
The above is the detailed content of How to print in golang. For more information, please follow other related articles on the PHP Chinese website!
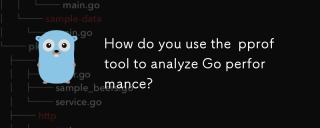
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
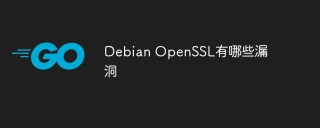
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
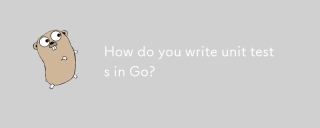
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
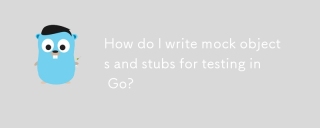
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
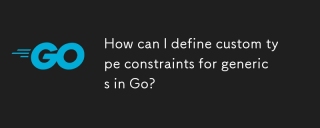
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
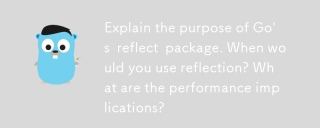
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
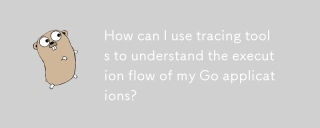
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
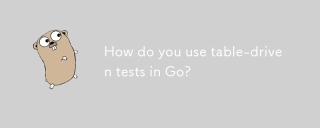
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
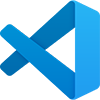
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.