Golang is a modern programming language that attracts more and more developers with its simplicity and efficiency. In Golang, channel is a very important feature, which can help developers achieve data synchronization and communication in a concurrent environment. However, in the actual development process, we also need to understand how to close the channel. This article will introduce how to use Golang to close channels.
When do you need to close the channel?
In Golang, channel is a data type used to implement data synchronization and communication. Normally, we use channels to send and receive data. When we send data, if the receiver has received the data, the send operation will be blocked until the receiver takes the data. If there is no receiver, the send operation will be blocked until there is a receiver. Similarly, when we receive data, if the sender has already sent the data, the receiving operation will not be blocked. If there is no sender, the receiving operation will be blocked until there is a sender.
In some cases, we need to close the channel. When we close a channel, it can no longer be used to send data, but receiving operations can still continue until all data has been received. In Golang, we can close the channel through the close() function.
So, under what circumstances do you need to close the channel? Normally, we need to close the channel in the following situations:
- When we know that there is no more data to send, we can close the channel. This lets the recipient know that all data has been sent.
- When we need to tell the receiver that no more data will be sent, we can close the channel. This situation is usually used to notify the receiver that the sender has completed some operation, such as reading a file.
- When we use the select statement and need to close a channel, we can use the close() function. This will prevent the case clause in the select statement from being selected.
How to close channel?
In Golang, we can use the close() function to close the channel. The close() function will close an initialized channel and cannot close an uninitialized channel.
The syntax for closing a channel is as follows:
close(ch)
Among them, ch is the channel that needs to be closed.
It should be noted that closing a closed channel or nil channel will cause panic. Therefore, before calling the close() function, we need to first determine whether the channel has been closed or is nil.
How to determine whether the channel has been closed?
We can use two methods to determine whether the channel has been closed.
The first way, we can use the ok-idiom mode in Golang. When we read data from a channel, if the channel has been closed, the zero value of the channel element and false will be returned. Therefore, we can use this feature to determine whether the channel has been closed. The sample code is as follows:
// 创建一个长度为3的int类型channel ch := make(chan int, 3) // 往channel中发送3条数据 ch <p>The second way, we can use the range iterator in Golang to traverse the channel. When the channel has been closed, the range The iterator will automatically exit the loop. The sample code is as follows: </p><pre class="brush:php;toolbar:false">// 创建一个长度为3的int类型channel ch := make(chan int, 3) // 往channel中发送3条数据 ch <h4 id="Channel-blocking-problem">Channel blocking problem</h4><p>When using channel, we need to pay attention to the channel blocking problem. When we send data to a channel that is full, the sending operation will be blocked until there is a free space in the channel; when we read data from an empty channel, the receiving operation will be blocked until there is a free space in the channel. data. </p><p>When closing the channel, there will also be blocking problems. When we send data to a closed channel, the send operation will be blocked until a receiver takes all the data from the channel. For example, the following code: </p><pre class="brush:php;toolbar:false">// 创建一个长度为1的string类型channel ch := make(chan string, 1) // 关闭channel close(ch) // 往channel中发送数据 ch <p>In this example, we create a string type channel with a length of 1, and then close the channel. Next, we try to send data to the channel, but because the channel has been closed, the sending operation will be blocked until a receiver takes all the data from the channel. </p><h4 id="Summary">Summary</h4><p>Through the introduction of this article, we have learned how to close the channel in Golang, and also learned how to determine whether the channel has been closed. When we use channels, we need to pay attention to channel blocking issues to avoid deadlocks and other problems. When using the select statement, you also need to note that closing a channel can prevent the case clause in the select statement from being selected. Hope this article is helpful to you. </p>
The above is the detailed content of How to close channel in golang. For more information, please follow other related articles on the PHP Chinese website!
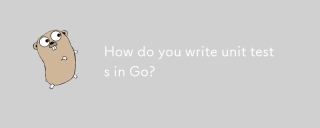
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
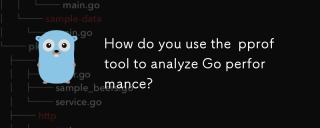
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
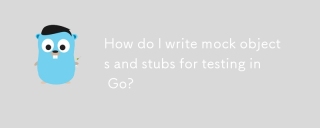
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
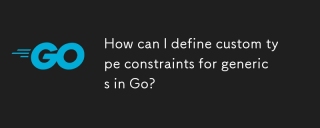
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
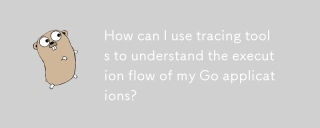
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
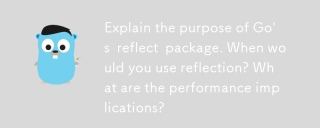
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
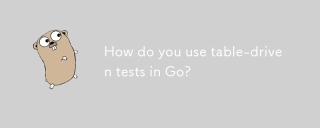
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
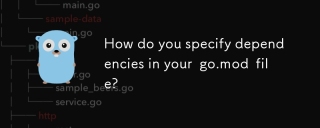
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
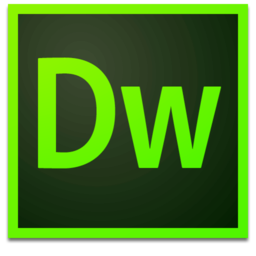
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
