


During development using Golang, we may need to convert different types of data into common []interface{} types. This problem is not complicated in Golang, but some details need to be paid attention to.
First, let us look at a simple example:
var slice []interface{} slice = append(slice, 1) slice = append(slice, "hello") slice = append(slice, []int{2, 3, 4}) fmt.Println(slice)
This code snippet creates an empty []interface{} slice, and then adds an int type, A string type and a []int type value. Finally, we output this slice using the Println method from the fmt package. The output is as follows:
[1 hello [2 3 4]]
As you can see, this slice contains values of different types, but they are all converted to interface{} types.
In the above example, we used the append method of the slice to operate on the slice. This is because when we add a value to the slice, Golang will automatically convert the value to the interface{} type. In other words, the following line of code:
slice = append(slice, 1)
is actually equivalent to this code:
slice = append(slice, interface{}(1))
, which converts the value 1 of the int type into the interface{} type.
However, if we want to convert a slice into the []interface{} type, the situation will be somewhat different. Because the slice type in Golang is known, converting it to the []interface{} type requires us to manually convert it. Here is an example:
arr := []int{1, 2, 3, 4} slice := make([]interface{}, len(arr)) for i, v := range arr { slice[i] = v } fmt.Println(slice)
This code snippet converts the slice []int{1, 2, 3, 4} to the []interface{} type, and then outputs the slice of this []interface{} type. The output result is as follows:
[1 2 3 4]
As you can see, each element in the slice has been converted to the interface{} type.
In the above example, we first created a slice of type []int and assigned the value {1, 2, 3, 4}. Then, we create a new slice of type []interface{} with the same length as arr. Next, we use a for loop to traverse the arr slice and assign each element to the corresponding position of the slice. Note that we need to use interface{}(v) to convert elements of type int to type interface{}. Finally, we output the slice slice.
It should be noted that if we use the append method to add elements to a slice of type []interface{}, the newly added elements need to be converted. For example, the following code snippet adds a slice of type []int to a slice of type []interface{}:
arr := []int{1, 2, 3, 4} slice := make([]interface{}, 0) slice = append(slice, arr...) fmt.Println(slice)
The output is as follows:
[1 2 3 4]
In this code snippet , we first created a slice arr of type []int. Then, we create an empty slice of type []interface{}. The syntactic sugar of slice... is used here to convert arr into the form of variable parameters. Finally, we add arr to the slice using the slice's append method.
In short, it is not difficult to convert different types of data into the common []interface{} type. We can use the append method to add values to the slice one by one, or use a for loop to convert the elements in a slice to the interface{} type and add them to a new []interface{} type slice. However, some details need to be paid attention to, such as newly added elements need to be converted manually, etc.
The above is the detailed content of How to convert different types of data into []interface{} type in golang. For more information, please follow other related articles on the PHP Chinese website!
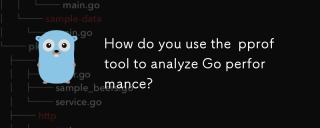
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
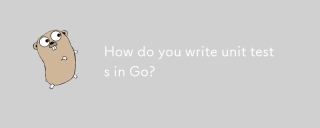
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
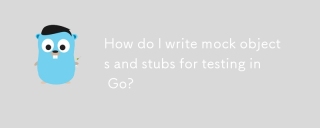
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
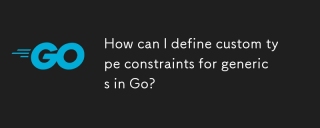
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
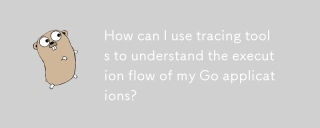
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
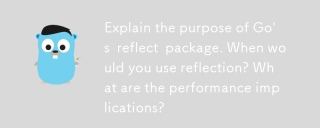
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
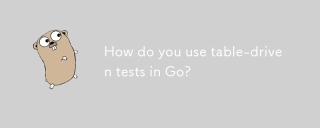
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
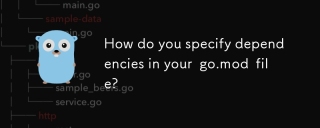
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
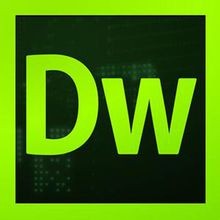
Dreamweaver CS6
Visual web development tools
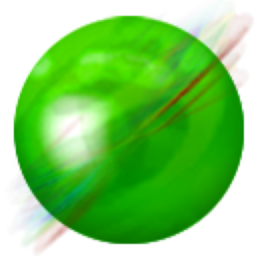
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
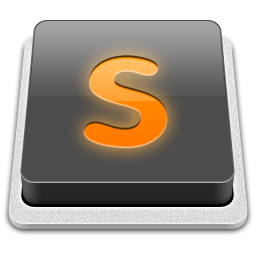
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
