


PHP is a scripting language widely used in website development. Its superior scalability and convenient operation have led to many websites using PHP as the back-end language. In website development, it is often necessary to present different pages according to the user's clicks, which requires the use of PHP to implement the function of displaying different pages on clicks.
There are many ways to implement the function of clicking to display different pages in PHP. Several implementation methods will be introduced below.
1. Use the if statement to realize clicking to display different pages
The if statement is the most basic branch statement in PHP, which can execute different codes based on logical conditions. In implementing the function of clicking to display different pages, you can use if statements to determine the user's clicking behavior, and then display the corresponding page based on the user's behavior. The following is a sample code:
if(isset($_GET['page'])){ $page = $_GET['page']; if($page == 'home'){ include('home.php'); }elseif($page == 'about'){ include('about.php'); }elseif($page == 'contact'){ include('contact.php'); }else{ include('404.php'); } }else{ include('home.php'); }
In the above code, first use the isset() function to determine whether there is a $_GET['page'] parameter passed. If so, get the value of the parameter and use it according to the The value uses an if statement to determine which page should be displayed. If the user does not specify a page number, the home.php page is displayed by default.
2. Use the switch statement to realize clicking to display different pages
The switch statement is another branch statement in PHP. It is similar to the if statement and can also execute different codes according to different conditions. In the function of clicking to display different pages, you can also use the switch statement to handle the user's click behavior. The following is a sample code:
switch($_GET['page']){ case 'home': include('home.php'); break; case 'about': include('about.php'); break; case 'contact': include('contact.php'); break; default: include('404.php'); break; }
In the above code, use the switch statement to determine the value of $_GET['page'], and include the corresponding page file based on different values. If there is no matching value, the 404.php page is included.
3. Use arrays to realize clicking to display different pages
In addition to using if statements and switch statements to realize the function of clicking to display different pages, you can also use arrays to store information on all pages. Then get the corresponding page based on the value clicked by the user. The following is a sample code:
$pages = array( 'home' => 'home.php', 'about' => 'about.php', 'contact' => 'contact.php' ); if(isset($_GET['page'])){ $page = $_GET['page']; if(array_key_exists($page, $pages)){ include($pages[$page]); }else{ include('404.php'); } }else{ include('home.php'); }
In the above code, an array $pages containing all page information is first defined, and then the isset() function is used to determine whether there is a $_GET['page'] parameter passed, and get the value of this parameter. Then use the array_key_exists() function to determine whether $page exists in the $pages array. If it exists, the corresponding page is included. If it does not exist, the 404.php page is included.
Summary
Whether you use if statements, switch statements or arrays to implement the function of clicking to display different pages, the essence is to make different responses based on the user's behavior. In actual website development, different implementation methods can be selected according to specific needs to achieve the best user experience.
The above is the detailed content of How to implement the function of clicking to display different pages in PHP. For more information, please follow other related articles on the PHP Chinese website!
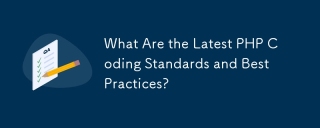
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
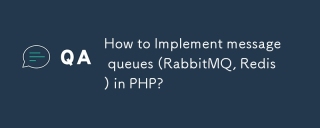
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
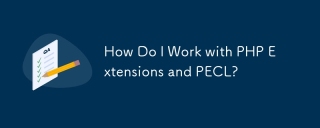
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
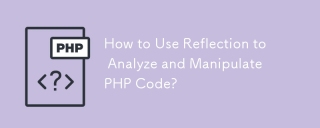
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
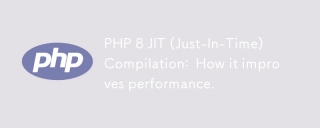
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
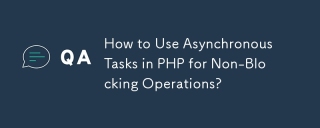
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
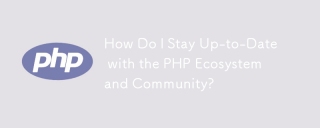
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
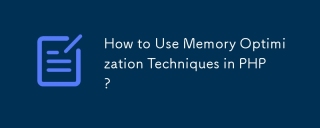
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
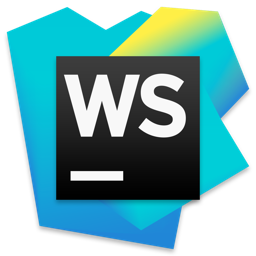
WebStorm Mac version
Useful JavaScript development tools
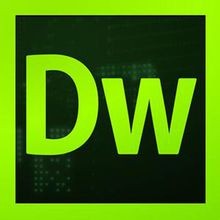
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
