Golang is an efficient and concise programming language, which has attracted the attention of more and more developers in recent years. Among them, Golang is increasingly used in network communications, among which heartbeat is one of the important applications. This article will introduce how to use Golang to implement the heartbeat function.
1. What is heartbeat
The reliability of network connections is a matter of concern to everyone. One of the methods is to maintain the status of the connection through a heartbeat mechanism. The heartbeat mechanism refers to sending some messages regularly within a certain time interval to ensure the activity of the connection. When the receiver does not receive these messages within the specified time, it means that the connection is disconnected, and reconnection or other operations are required.
2. How the heartbeat works
The working process of the heartbeat mechanism is as follows:
- Establish a connection between the client and the server.
- The client sends a heartbeat packet to the server at certain intervals.
- After receiving the heartbeat data packet, the server replies to the client with a data packet.
- If the server fails to reply to the data packet within the specified time, it means that the connection has been disconnected at this time.
- The client reconnects from the disconnected state in the previous step.
3. Golang code to implement heartbeat
The following is a simple Golang code implementation:
package main import ( "fmt" "net" "time" ) func main() { tcpAddr, _ := net.ResolveTCPAddr("tcp4", "127.0.0.1:8080") conn, _ := net.DialTCP("tcp", nil, tcpAddr) // 心跳包发送间隔 interval := 3 * time.Second timer := time.NewTimer(interval) for { select { // 发送心跳包 case 0 { fmt.Printf("recv data: %s\n", string(buf[:n])) } // 睡眠一定时间,避免占用CPU过高 time.Sleep(100 * time.Millisecond) } } }
In the above code, first create a tcp connection, and then use time.NewTimer()
The function creates a timer and sends a heartbeat packet to the server at certain intervals. When the data packet is sent successfully, a log is output. At the same time, use the conn.Read()
method to receive the message returned by the server and output the corresponding data.
4. Summary
This article introduces the method of using Golang language to implement the heartbeat mechanism. The heartbeat mechanism can be used to ensure the reliability of network connections and avoid data transmission errors caused by connection interruptions. With simple Golang code, we can easily implement this functionality.
The above is the detailed content of How to use Golang to implement heartbeat function. For more information, please follow other related articles on the PHP Chinese website!
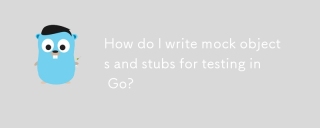
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
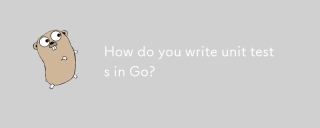
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
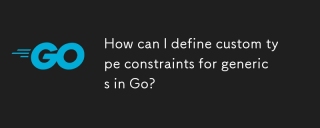
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
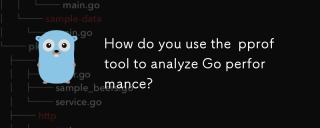
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
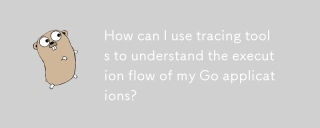
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
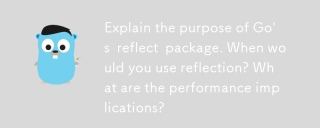
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
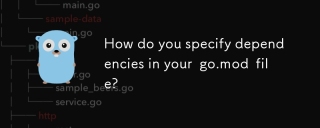
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
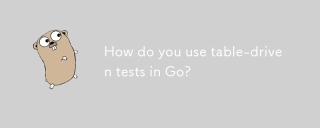
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
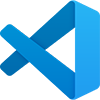
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
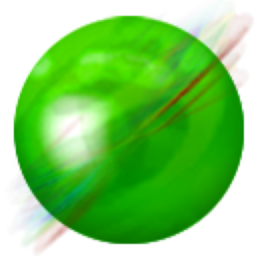
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
