How to change the AJAX response header without writing PHP
AJAX (Asynchronous JavaScript and XML) is a commonly used Web development technology, which can request data asynchronously without refreshing the entire web page, thus Improve user experience. During the development process, in addition to the requested URL and POST parameters, the AJAX response header is also a very important part.
In some complex applications, developers need to control the content of the AJAX response header to make it consistent with the application's logic. In PHP, the header() function is usually used to set the AJAX response header. However, in some cases, we may need to modify the AJAX response headers without using PHP. This article will introduce some very practical ways to achieve this purpose.
1. Using jQuery
jQuery is a very popular JavaScript library that simplifies interaction with the DOM and AJAX. In jQuery, we can use the following code to modify the AJAX response header:
$.ajaxSetup({ beforeSend: function(jqXHR) { jqXHR.overrideMimeType("text/plain; charset=x-user-defined"); } });
Through the beforeSend function, we can modify the properties of the jqXHR object to achieve the purpose of modifying the AJAX response header.
2. Using XMLHttpRequest
XMLHttpRequest is a native API in JavaScript, which can request data from the server without refreshing the entire page. Through XMLHttpRequest, we can request data from the server and modify the response headers after receiving the response. The following is an example of using XMLHttpRequest:
var xhr = new XMLHttpRequest(); xhr.open('GET', '/mydata', true); xhr.onreadystatechange = function() { if (xhr.readyState == XMLHttpRequest.DONE && xhr.status == 200) { xhr.getResponseHeader('Content-Type'); // 获取响应头 xhr.overrideMimeType('text/plain; charset=x-user-defined'); // 修改响应头 console.log(xhr.responseText); } }; xhr.send();
Through the overrideMimeType() function, we can modify the response header of the XMLHttpRequest object.
3. Using Fetch API
Fetch API is a JavaScript API for making network requests. It provides a simple and elegant way so that we can send HTTP requests and get responses. data. Through the Fetch API, we can request data from the server and modify the response headers after receiving the response. The following is an example of using the Fetch API:
fetch('/mydata') .then(function(response) { response.headers.get('Content-Type'); // 获取响应头 response = new Response(response.body, { headers: { 'Content-Type': 'text/plain; charset=x-user-defined' // 修改响应头 } }); console.log(response); });
By setting the headers property of the Response object, we can modify the response headers.
4. Summary
This article introduces three ways to modify the AJAX response header without using PHP. Using jQuery, XMLHttpRequest and the Fetch API are all very simple and elegant ways to give us better control over the content of the AJAX response headers in order to implement our application logic.
The above is the detailed content of How to change ajax response header without writing php. For more information, please follow other related articles on the PHP Chinese website!
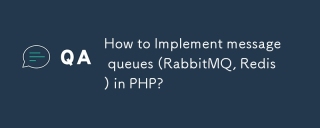
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
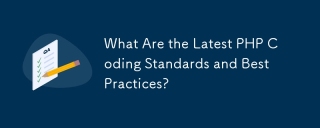
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
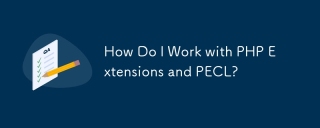
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
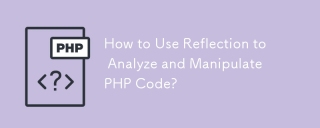
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
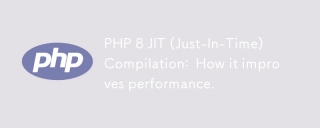
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
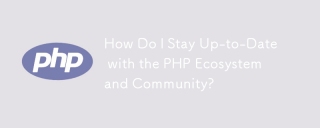
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
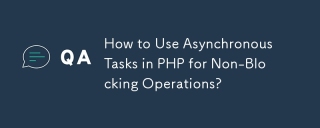
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
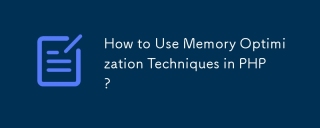
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
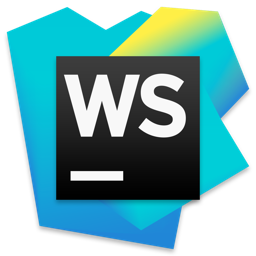
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
