After the program is written in the Golang language, it needs to be set up and run, which includes some operations that interact with the operating system.
1. Compile
In Golang, you need to execute the following instructions when compiling:
go build
This instruction will compile the main package into an executable file and create it in the current directory.
You can also use the go install
command to install the compiled executable file in the $GOPATH/bin directory or the $GOBIN directory (the $GOBIN directory needs to be set in the environment variable set up).
2. Cross-compilation
If you need to run Golang programs on other system platforms, you need to cross-compile. Cross-compiling refers to compiling a program into a binary file that can run on other operating systems.
Cross compilation is very simple, you only need to set the two environment variables GOOS and GOARCH during compilation. For example, the following is an example of compiling a Mac version executable file under Linux:
GOOS=darwin GOARCH=amd64 go build
3. Environment variables
Golang supports setting environment variables to affect the running of the program. The following are some commonly used environment variables:
- GOPATH: Set the location of the Golang workspace directory (workspace). This directory contains all Golang source code, libraries and executable files.
- GOBIN: Set the default storage path for Golang executable files.
- GOROOT: Set the installation directory of Golang.
4. Running parameters and flags
In Golang, the program can receive parameters and flags (flag) to affect the operation. The following is an example of a program that passes name and age through parameters:
package main import ( "flag" "fmt" ) func main() { var name string var age int flag.StringVar(&name, "name", "", "姓名") flag.IntVar(&age, "age", 0, "年龄") flag.Parse() fmt.Printf("你好,我叫%s,今年%d岁", name, age) }
You can pass parameters like this during runtime:
./example -name=张三 -age=18
The output result is:
你好,我叫张三,今年18岁
Pass parameters It is very convenient to pass data with and flags, and different judgments and processing can be performed in the code. Especially when writing network services, parameters such as IP and port can be passed in a similar way.
5. Conclusion
The above is a brief introduction to Golang settings and operations. Of course, this is far from the most comprehensive. The operation settings of Golang language are very powerful, and also include version control. , dependency management and other various settings. It is recommended that you use Golang more in your daily work and have the opportunity to gain in-depth knowledge of its operating settings so that you can better deal with various scenarios.
The above is the detailed content of Let's talk about some settings and operations of golang. For more information, please follow other related articles on the PHP Chinese website!
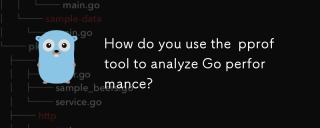
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
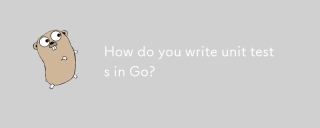
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
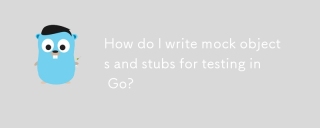
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
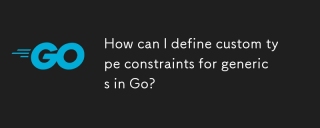
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
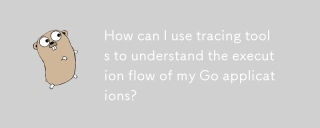
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
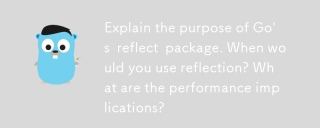
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
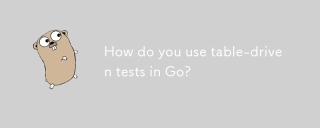
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
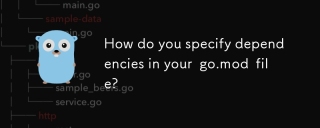
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
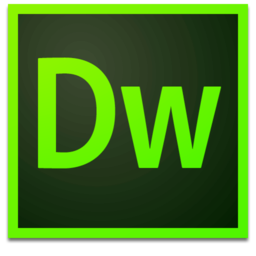
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
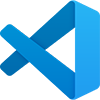
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
