


How golang solves the problem of memory overflow caused by too large structures
With the rapid development of cloud computing and big data technology, more and more enterprises and developers are beginning to choose to use Go language to develop high-performance and high-concurrency applications. In the Go language, structure is a very commonly used data type. It can be used to represent a set of related data, and methods can be defined like objects to operate on this data. However, in the actual development process, we may encounter some problems, such as memory overflow caused by too large a structure. This article will introduce how to solve the problem of memory overflow caused by too large structures in Go language.
1. Why does a structure that is too large lead to memory overflow?
In the Go language, a structure is a value type data type, and its size is determined by its member variables. In the Go language, memory is a limited resource. If we define a very large structure, it will occupy a lot of memory space. When we create this structure object, if there is not enough memory, memory overflow and garbage collection problems will occur. These issues not only affect the performance of the application, but may also cause the application to crash.
2. How to solve the problem of memory overflow caused by too large structures?
1. Use pointers
In Go language, we can use pointers to avoid the problem of excessively large structures. By using pointers, we can implement a reference to the structure instead of copying it directly. This can significantly reduce the memory space occupied by the structure. The sample code is as follows:
type Person struct{ Name string Age int Phone string } type Student struct { Person *Person Id string Class string }
In the above sample code, we define a Person structure and a Student structure. Since the Person structure is very large, we put it into the Student structure as a member variable of pointer type. This can significantly reduce the size of the structure and avoid memory overflow problems.
2. Use slicing or mapping
In the Go language, we can use slicing or mapping to store a large amount of structure data. Using slicing or mapping can easily store and access large amounts of data, and can avoid memory overflow problems. The sample code is as follows:
type Person struct{ Name string Age int Phone string } var persons []Person func addPerson(p Person) bool { persons = append(persons, p) return true } func findPerson(name string) (*Person, bool) { for _, p := range persons { if p.Name == name { return &p, true } } return nil, false }
In the above sample code, we define a Person structure and a global persons slice. Through the addPerson function and findPerson function, we can easily add and find a large amount of Person structure data.
3. Use paging query
In practical applications, we often do not need to load all structure data into memory at one time, but can use paging query to avoid memory overflow. The problem. Through paging queries, we can load a large amount of data into small chunks to avoid occupying too much memory space at one time. The sample code is as follows:
type Person struct{ Name string Age int Phone string } var persons []Person func getPersons(page int, pageSize int) ([]Person, bool) { start := page * pageSize end := start + pageSize if start >= len(persons){ return nil, false } if end > len(persons){ end = len(persons) } return persons[start:end], true }
In the above sample code, we define a getPersons function. By passing in the page number and page size, we can get paginated data from the persons slice. This can avoid taking up too much memory space at one time and improve application performance.
Summary
Structure is a very commonly used data type in the Go language, but when the structure is too large, it can easily lead to memory overflow problems. In actual development, we can solve the problem of memory overflow caused by too large structures by using pointers, slicing or mapping, paging queries, etc. These methods can not only improve the performance of the application, but also ensure the stability of the application.
The above is the detailed content of How golang solves the problem of memory overflow caused by too large structures. For more information, please follow other related articles on the PHP Chinese website!
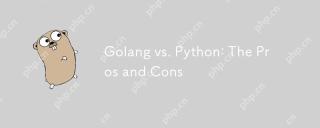
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
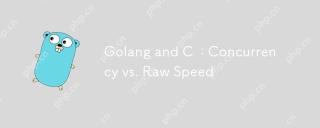
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
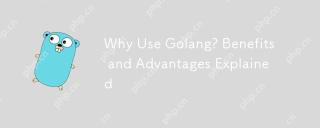
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
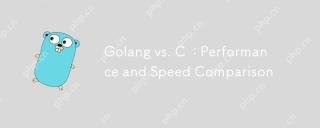
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
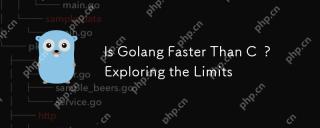
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
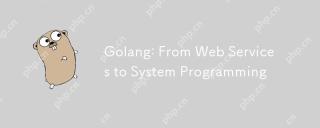
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
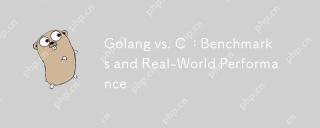
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
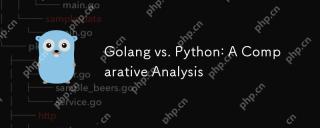
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.