In web development, sometimes it is necessary to display files in the browser instead of letting the browser download the files locally. This process can be accomplished through PHP code, and this article will detail how to use PHP to turn off browser downloads.
- Using HTTP header files
The HTTP header file is part of the HTTP request and response, and it contains the information required for the HTTP response. We can use the header function in PHP to set the HTTP header file to open the file in the browser.
The following is a simple example showing how to use the header function to display a PDF file in a browser:
<?php $file = 'sample.pdf'; header('Content-type: application/pdf'); header('Content-Disposition: inline; filename="' . $file . '"'); header('Content-Transfer-Encoding: binary'); header('Content-Length: ' . filesize($file)); header('Accept-Ranges: bytes'); @readfile($file); ?>
This code first opens a PDF file, and then uses the header function to set the HTTP header document. Among them, Content-type tells the browser that the response content is in PDF format. Content-Disposition: inline allows the browser to display the file inline in the page. Content-Transfer-Encoding: binary specifies that the file is transferred in binary mode. Content -Length specifies the data size of the response, and Accept-Ranges: bytes specifies that the server supports requests in byte ranges.
Finally, use the readfile function to read the file content and display it in the browser in HTML format.
- Handling different file types
In addition to PDF, we can also use the header function to display other types of files, such as pictures, audio, videos, etc. Just specify the file type in Content-type.
The following are some common file types and their Content-type values:
File type | Content-type |
---|---|
Picture | image/jpeg, image/png, image/gif, image/bmp |
application/pdf | |
text/plain | |
audio/mpeg, audio/ogg, audio/wav | |
video/mp4, video/ogg, video/webm |
<?php $file = 'sample.jpg'; header('Content-type: image/jpeg'); header('Content-Disposition: inline; filename="' . $file . '"'); header('Content-Transfer-Encoding: binary'); header('Content-Length: ' . filesize($file)); header('Accept-Ranges: bytes'); @readfile($file); ?>
- Download file
<?php $file = 'sample.zip'; $filename = 'download.zip'; header('Content-type: application/zip'); header('Content-Disposition: attachment; filename="' . $filename . '"'); header('Content-Transfer-Encoding: binary'); header('Content-Length: ' . filesize($file)); header('Accept-Ranges: bytes'); @readfile($file); ?>In this example, we set the Content-Disposition header to instruct the browser to download the file. The filename parameter is used to specify the file name of the downloaded file.
- Handling large files
<?php $file = 'bigfile.zip'; $filename = 'download.zip'; $chunksize = 4096; header('Content-type: application/zip'); header('Content-Disposition: attachment; filename="' . $filename . '"'); header('Content-Transfer-Encoding: binary'); header('Accept-Ranges: bytes'); header('Content-Length: ' . filesize($file)); $handle = fopen($file, 'rb'); while (!feof($handle)) { $buffer = fread($handle, $chunksize); echo $buffer; ob_flush(); flush(); } fclose($handle); ?>In this example, we read the file content of 4096 bytes each time and then output it block by block. Notice that inside the loop, we use the ob_flush and flush functions to push the contents of the buffer to the browser. Summary: Use PHP to turn off browser downloadsIn this article, we introduced how to use PHP to turn off browser downloads, including displaying files, processing different file types, downloading files, and processing large files. document. This knowledge is very important for web developers. I hope this article can help you.
The above is the detailed content of How to close browser download using php. For more information, please follow other related articles on the PHP Chinese website!
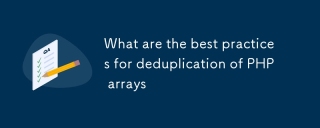
This article explores efficient PHP array deduplication. It compares built-in functions like array_unique() with custom hashmap approaches, highlighting performance trade-offs based on array size and data type. The optimal method depends on profili
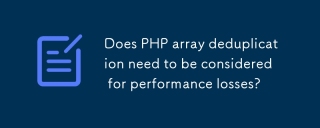
This article analyzes PHP array deduplication, highlighting performance bottlenecks of naive approaches (O(n²)). It explores efficient alternatives using array_unique() with custom functions, SplObjectStorage, and HashSet implementations, achieving
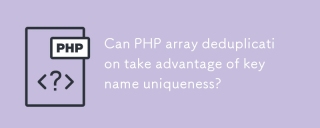
This article explores PHP array deduplication using key uniqueness. While not a direct duplicate removal method, leveraging key uniqueness allows for creating a new array with unique values by mapping values to keys, overwriting duplicates. This ap
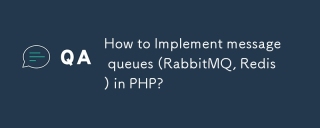
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
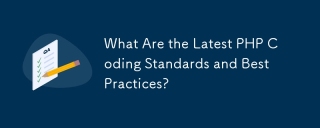
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
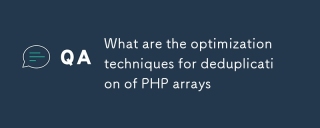
This article explores optimizing PHP array deduplication for large datasets. It examines techniques like array_unique(), array_flip(), SplObjectStorage, and pre-sorting, comparing their efficiency. For massive datasets, it suggests chunking, datab
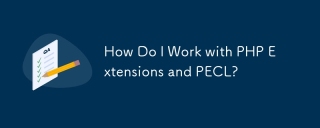
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
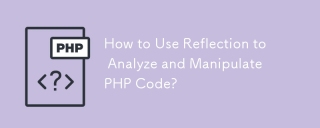
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
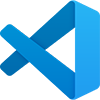
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
