Stochastic Gradient Descent (SGD) is an optimization algorithm commonly used for parameter optimization in machine learning. In this article, we will introduce how to implement SGD using Go language (Golang) and give implementation examples.
- SGD algorithm
The basic idea of the SGD algorithm is to randomly select some samples in each iteration and calculate the loss function of these samples under the current model parameters. The gradient is then calculated on these samples and the model parameters are updated according to the direction of the gradient. This process will be repeated several times until the stopping condition is met.
Specifically, let $f(x)$ be the loss function, $x_i$ be the feature vector of the $i$-th sample, $y_i$ be the output of the $i$-th sample, $w $ is the current model parameter, and the update formula of SGD is:
$$w = w - \alpha \nabla f(x_i, y_i, w)$$
where $\alpha$ is Learning rate, $\nabla f(x_i, y_i, w)$ represents the calculation of the loss function gradient of the $i$th sample under the current model parameters.
- Golang implementation
The libraries required to implement the SGD algorithm in Golang are: gonum
, gonum/mat
and gonum/stat
. Among them, gonum
is a mathematical library that provides many commonly used mathematical functions. gonum/mat
is a library used to process matrices and vectors. gonum/stat
is Statistical functions (such as mean, standard deviation, etc.) are provided.
The following is a simple Golang implementation:
package main import ( "fmt" "math/rand" "gonum.org/v1/gonum/mat" "gonum.org/v1/gonum/stat" ) func main() { // 生成一些随机的数据 x := mat.NewDense(100, 2, nil) y := mat.NewVecDense(100, nil) for i := 0; i <p>The data set of this implementation is a $100 \times 2$ matrix, each row represents a sample, and each sample has two features. Label $y$ is a $100 \times 1$ vector, where each element is either 0 or 1. The number of iterations in the code is 1000 and the learning rate $\alpha$ is 0.01. </p><p>In each iteration, a sample is randomly selected and the loss function gradient is calculated on this sample. After the gradient calculation is completed, update the model parameters using the formula above. Finally, the model parameters are output. </p><ol start="3"><li>Summary</li></ol><p>This article introduces how to use Golang to implement the SGD algorithm and gives a simple example. In practical applications, there are also some variations of the SGD algorithm, such as SGD with momentum, AdaGrad, Adam, etc. Readers can choose which algorithm to use based on their own needs. </p>
The above is the detailed content of How to implement sgd in golang. For more information, please follow other related articles on the PHP Chinese website!
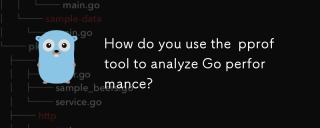
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
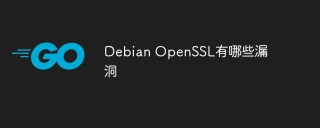
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
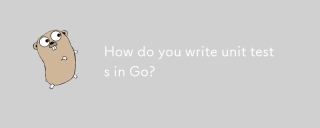
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
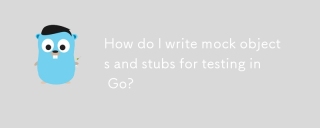
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
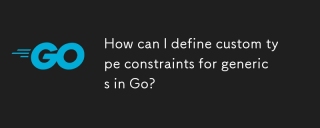
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
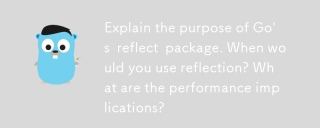
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
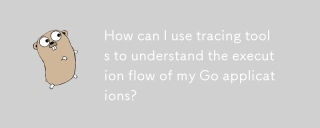
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
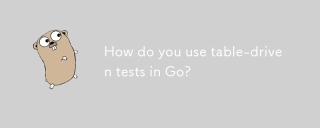
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
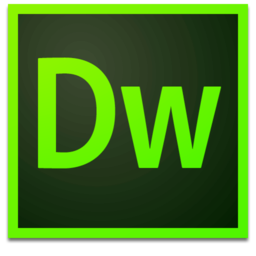
Dreamweaver Mac version
Visual web development tools
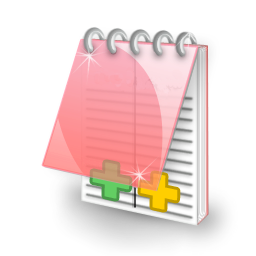
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.