Many websites require a complete backend management system to manage data, update content and interact with users. To implement a complete backend management system, you need to use the PHP language.
This article will introduce how to build a complete backend management system through PHP5 language, and comes with complete file source code.
First of all, we need to understand what the PHP5 language is. PHP5 is a server-side scripting language that can be used to create dynamic web pages. It is open source, easy to learn and use, and runs on various platforms. PHP5 supports integration with databases, so it is very suitable for developing complete backend management systems.
Before we start writing code, we need to prepare some tools and software. First you need a web server such as Apache or Nginx. Secondly, a database is required, such as MySQL or SQLite. Finally you need a text editor such as Sublime Text or Notepad.
We will use PHP5 language to write the following functions:
- Register a new user
- User login
- Modify user information
- Upload files
- Manage files
- Delete files
The following is the complete file source code:
<?php // 连接数据库 $link = mysqli_connect("localhost", "root", "password", "database_name"); // 注册新用户 function register_user($username, $password) { global $link; $username = mysqli_real_escape_string($link, $username); $password = mysqli_real_escape_string($link, $password); $password = password_hash($password, PASSWORD_DEFAULT); $sql = "INSERT INTO users (username, password) VALUES ('$username', '$password')"; mysqli_query($link, $sql); } // 用户登录 function login_user($username, $password) { global $link; $username = mysqli_real_escape_string($link, $username); $password = mysqli_real_escape_string($link, $password); $sql = "SELECT * FROM users WHERE username='$username'"; $result = mysqli_query($link, $sql); if (mysqli_num_rows($result) == 1) { $row = mysqli_fetch_assoc($result); if (password_verify($password, $row['password'])) { $_SESSION['user'] = $row['id']; return true; } } return false; } // 修改用户信息 function update_user($username, $password) { global $link; $username = mysqli_real_escape_string($link, $username); $password = mysqli_real_escape_string($link, $password); $password = password_hash($password, PASSWORD_DEFAULT); $id = $_SESSION['user']; $sql = "UPDATE users SET username='$username', password='$password' WHERE id='$id'"; mysqli_query($link, $sql); } // 上传文件 function upload_file($filename, $filepath) { global $link; $filename = mysqli_real_escape_string($link, $filename); $filepath = mysqli_real_escape_string($link, $filepath); $user_id = $_SESSION['user']; $sql = "INSERT INTO files (filename, filepath, user_id) VALUES ('$filename', '$filepath', '$user_id')"; mysqli_query($link, $sql); } // 管理文件 function manage_files() { global $link; $user_id = $_SESSION['user']; $sql = "SELECT * FROM files WHERE user_id='$user_id'"; $result = mysqli_query($link, $sql); while ($row = mysqli_fetch_assoc($result)) { echo "<tr><td>{$row['filename']}</td><td>{$row['filepath']}</td><td><a>Delete</a></td>"; } } // 删除文件 function delete_file($id) { global $link; $id = mysqli_real_escape_string($link, $id); $user_id = $_SESSION['user']; $sql = "DELETE FROM files WHERE id='$id' AND user_id='$user_id'"; mysqli_query($link, $sql); } ?>
The above code uses the mysqli extension function, which provides Secure data processing and MySQL data access capabilities. It should be noted that some global variables are used in the code, such as $link and $_SESSION. These variables need to be defined or initialized in other parts of the code.
Functions such as registering new users, logging in, modifying user information, uploading files, managing files, and deleting files are all explained in detail in the code. Developers can freely modify and adjust according to their own needs.
Summary: This article introduces how to use PHP5 language to build a complete backend management system, and comes with complete file source code. Security and performance need to be paid attention to during development to ensure the correctness and reliability of the code.
The above is the detailed content of Front and backend php5 build complete file source code. For more information, please follow other related articles on the PHP Chinese website!
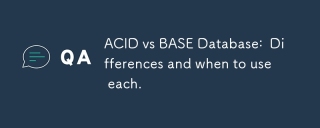
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
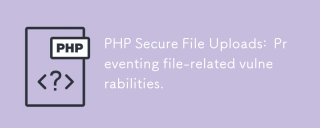
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
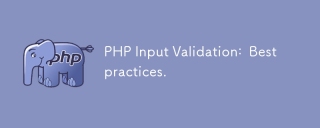
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
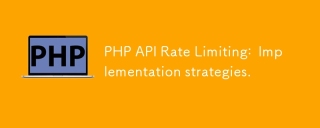
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
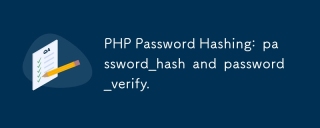
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
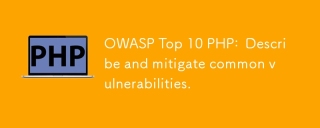
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
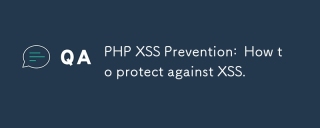
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor
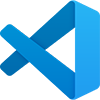
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software