Golang is a very popular programming language and its time processing function is very powerful. In this post, I will introduce how to do time conversion in Golang.
First, we need to understand the time type in Golang. There are two time types in Golang: time.Time and time.Duration. time.Time represents a specific point in time, and time.Duration represents a time period.
We can use the time.Parse function to convert the string to the time.Time type. For example, the following code will convert the string "2022-01-01 00:00:00" to the time.Time type:
str := "2022-01-01 00:00:00" layout := "2006-01-02 15:04:05" t, err := time.Parse(layout, str) if err != nil { fmt.Println(err) return }
In the above code, layout is the date format string, which Specifies how to convert a string to a time. In Golang, the date format string must be in a specific format and consist of fixed characters. For example, 2006 represents the year, 01 represents the month, 02 represents the date, 15 represents the hour, 04 represents the minute, and 05 represents the second.
Next, we can use the time.Format function to convert the time.Time type to a string. For example, the following code will convert a variable t of type time.Time into a string:
layout := "2006-01-02 15:04:05" str := t.Format(layout) fmt.Println(str)
In addition to date formatting strings, Golang also provides some other functions for time conversion. For example, a value of type time.Duration represents a time period. We can convert the string to time.Duration type using time.ParseDuration function. For example, the following code will convert the string "1h30m" to the time.Duration type:
str := "1h30m" duration, err := time.ParseDuration(str) if err != nil { fmt.Println(err) return }
Finally, we can use various functions provided by the time package to operate on time. For example, we can use the time.Add function to calculate the time after a point in time. For example, the following code will calculate the time 3 hours after the current time:
duration := 3 * time.Hour t := time.Now().Add(duration)
Time conversion in Golang is very simple. We can easily convert time from one format to another just by using the functions of the time package. Hope this article can help you deal with time in Golang.
The above is the detailed content of How to do time conversion in Golang. For more information, please follow other related articles on the PHP Chinese website!
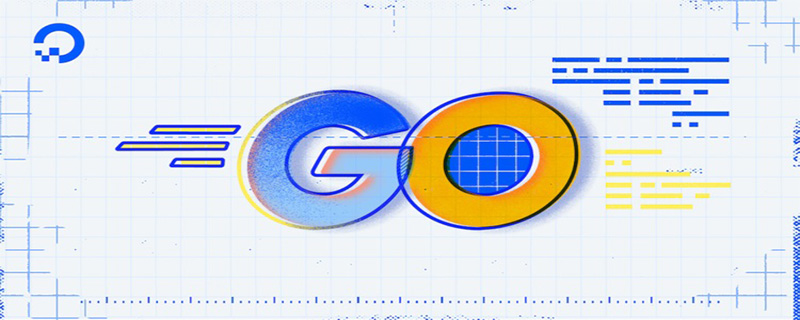
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
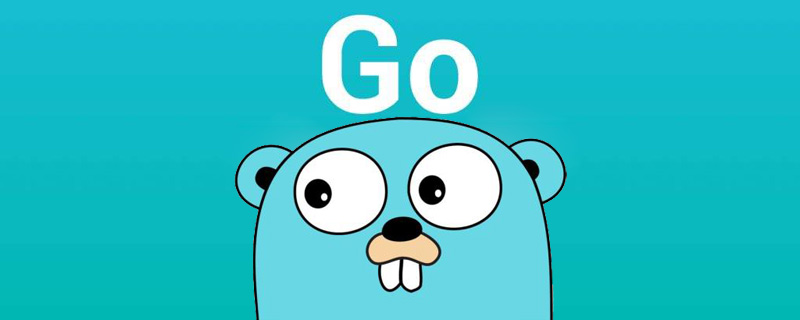
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
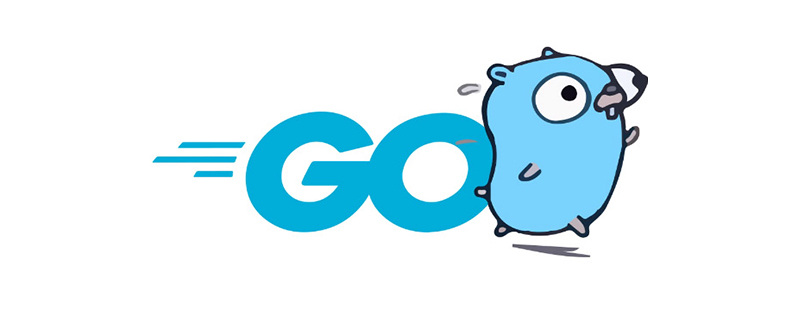
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
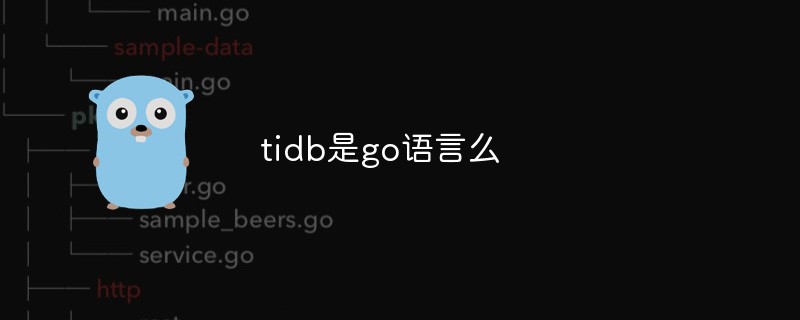
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
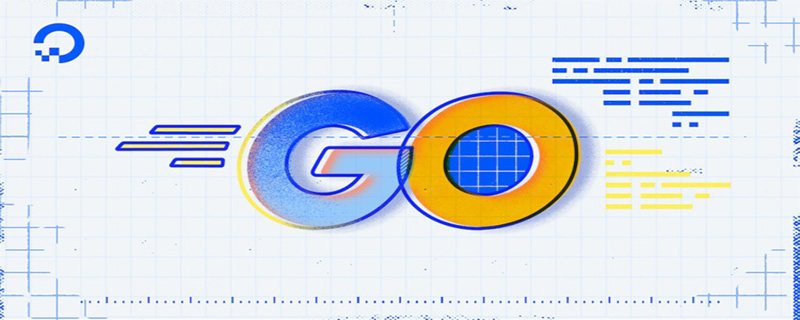
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
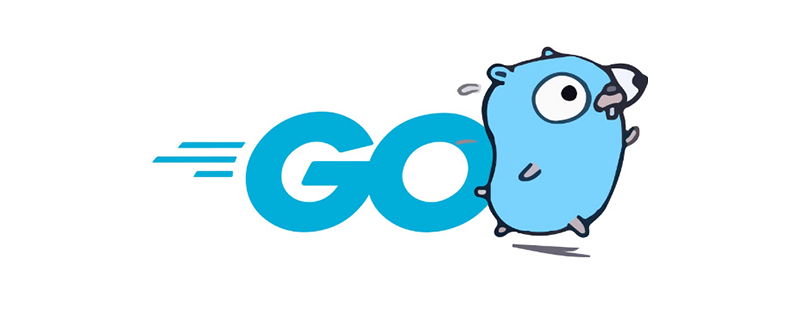
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
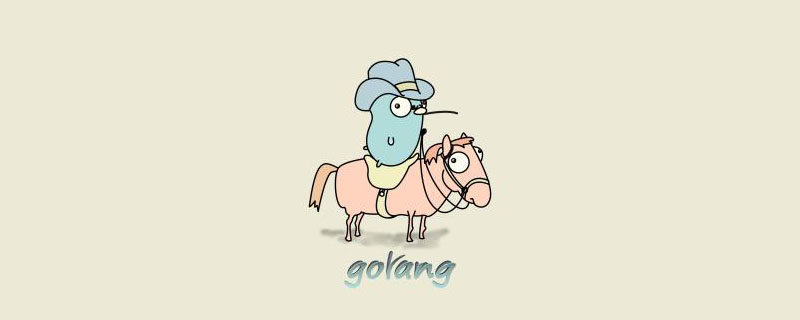
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
